Android Vote and Rating (PHP and MySQL) |
Android Vote and Rating (PHP and MySQL) บทความการเขียนโปรแกรมบน Android เพื่อสร้างระบบ Vote ด้วย RatingBar Widgets แบบง่าย ๆ โดยใช้ PHP กับ MySQL ทำงานอยู่ในฝั่งของ Web Server ซึ่งจะจัดเก็บข้อมูลตารางชื่อว่า images ในแถวของแต่ล่ะ รายการจะมีรูปภาพ และคำอธิบายรูปภาพ และข้อมูลเหล่านี้จะถูก Request จาก Android ผ่าน HttpPost และ HttpGet โดยข้อมูลจะถูกส่งในรูปแบบของ JSON เมื่อ Android ได้รับข้อมูลเหล่านั้นก็จะนำข้อมูลทีได้แสดงบน Application รอให้ผู้ใช้เข้าไปทำการ Vote และเมื่อ Vote เรียบร้อยแล้ว จะมีการแสดง Point ตอนแต่ล่ะรายการด้วย RatingBar
จากภาพแสดงการทำงานของ Android ซึ่งประกอบด้วย Activity 2 ตัว ตัวแรกเป็นรายการข้อมูลที่แสดงบน ListView และรายการที่สองเมื่อคลิกแต่ล่ะรายการจะแสดง รายละเอียดพร้อมกับ RatingBar สำหรับการโหวดให้คะแนน พร้อม ๆ กับส่งค่าไป Update ที่ Web Server
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Server (PHP and MySQL)
MySQL Database
CREATE TABLE `images` (
`ImageID` int(11) NOT NULL auto_increment,
`ImageName` varchar(50) NOT NULL,
`ImagePath_Thumbnail` varchar(150) NOT NULL,
`ImagePath_FullPhoto` varchar(150) NOT NULL,
`Rating` float NOT NULL,
PRIMARY KEY (`ImageID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=7 ;
--
-- Dumping data for table `images`
--
INSERT INTO `images` VALUES (1, 'Image 1', 'https://www.thaicreate.com/android/img1_thum.jpg', 'https://www.thaicreate.com/android/img1_full.jpg', 0);
INSERT INTO `images` VALUES (2, 'Image 2', 'https://www.thaicreate.com/android/img2_thum.jpg', 'https://www.thaicreate.com/android/img2_full.jpg', 0);
INSERT INTO `images` VALUES (3, 'Image 3', 'https://www.thaicreate.com/android/img3_thum.jpg', 'https://www.thaicreate.com/android/img3_full.jpg', 0);
INSERT INTO `images` VALUES (4, 'Image 4', 'https://www.thaicreate.com/android/img4_thum.jpg', 'https://www.thaicreate.com/android/img4_full.jpg', 0);
INSERT INTO `images` VALUES (5, 'Image 5', 'https://www.thaicreate.com/android/img5_thum.jpg', 'https://www.thaicreate.com/android/img5_full.jpg', 0);
INSERT INTO `images` VALUES (6, 'Image 6', 'https://www.thaicreate.com/android/img6_thum.jpg', 'https://www.thaicreate.com/android/img6_full.jpg', 0);
โครงสร้างตารางและข้อมูล
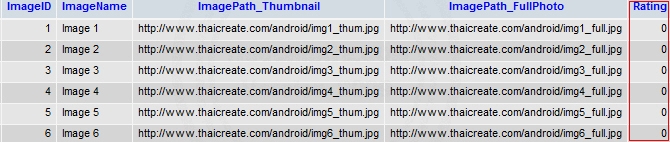
getGallery.php (เป็นไฟล์สำหรับแสดงข้อมูลบน ListView)
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM images WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
updateRating.php (เป็นไฟล์สำหรับ Update ข้อมูลที่ถูกส่งมาจาก Android Client)
<?php
//$_POST["ImageID"] = "1"; // ImageID
//$_POST["ratingPoint"] = "3.5"; // ratingPoint
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
/*** Update ***/
$strSQL = " UPDATE images SET
Rating = '".$_POST["ratingPoint"]."'
WHERE ImageID = '".$_POST["ImageID"]."'
";
$objQuery = mysql_query($strSQL);
if(!$objQuery)
{
$arr['StatusID'] = "0";
$arr['Error'] = "Cannot save data!";
}
else
{
$arr['StatusID'] = "1";
$arr['Error'] = "";
}
/**
$arr['StatusID'] // (0=Failed , 1=Complete)
$arr['Error'] // Error Message
*/
mysql_close($objConnect);
echo json_encode($arr);
?>
Android Project
โครงสร้างของไฟล์ทั้งหมด
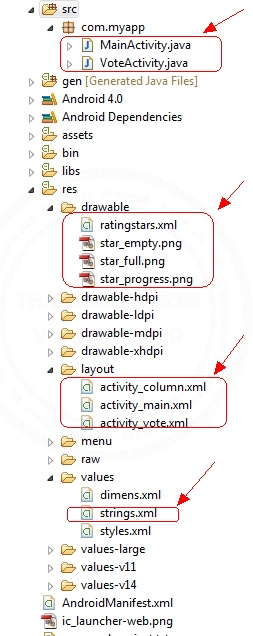
ในตัวอย่างนี้มีการใช้ Custom RatingBar ซึ่งจะสร้าง Style ขึ้นมามีรายละเอียดดังนี้
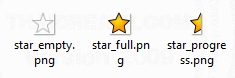
Icons รูปภาพ ที่จะใช้แสดงบน Custom RatingBar
/res/drawable/ratingstars.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+android:id/background"
android:drawable="@drawable/star_empty" />
<item android:id="@+android:id/secondaryProgress"
android:drawable="@drawable/star_empty" />
<item android:id="@+android:id/progress"
android:drawable="@drawable/star_full" />
</layer-list>
สร้าง Style อยู่บนไฟล์ ratingstars.xml
/values/string.xml
<style name="styleRatingBar" parent="@android:style/Widget.RatingBar">
<item name="android:progressDrawable">@drawable/ratingstars</item>
<item name="android:minHeight">22dip</item>
<item name="android:maxHeight">22dip</item>
</style>
เพิ่มคำสั่งชุดนี้ลงใน string.xml
รายละเอียดเพิ่มเติมของ Custom RatingBar สามารถอ่านได้ที่นี่
ออกแบบ XML Layout และ Java ดังนี้
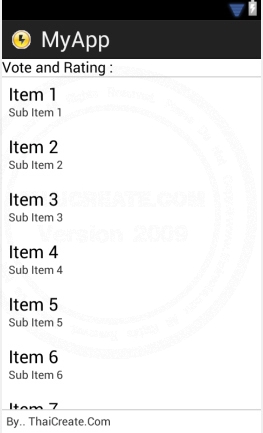
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Vote and Rating : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
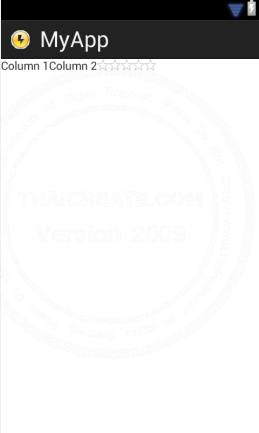
activity_column.xml
<TableLayout android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android">
<TableRow
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<ImageView
android:id="@+id/ColImgPath"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
<TextView
android:id="@+id/ColImgID"
android:text="Column 1" />
<TextView
android:id="@+id/ColImgName"
android:text="Column 2" />
<RatingBar
android:id="@+id/ColratingBar"
style="@style/styleRatingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:max="5"
android:numStars="5" />
</TableRow>
</TableLayout>
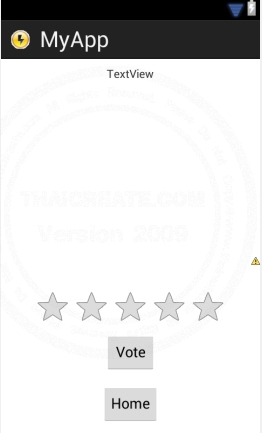
activity_vote.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/txtImageName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:text="TextView"
android:layout_marginTop="10dp" />
<ImageView
android:id="@+id/fullimage"
android:layout_width="match_parent"
android:layout_height="220dp"
android:layout_marginTop="35dp" />
<RatingBar
android:id="@+id/rating"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/fullimage"
android:layout_centerHorizontal="true"
android:max="5"
android:numStars="5"
android:layout_marginTop="25dp" />
<Button
android:id="@+id/btnVote"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/rating"
android:layout_centerHorizontal="true"
android:text="Vote" />
<Button
android:id="@+id/btnHome"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/btnVote"
android:layout_centerHorizontal="true"
android:layout_marginTop="15dp"
android:text="Home" />
</RelativeLayout>
MainActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.ByteArrayOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.Context;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.Menu;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.RatingBar;
import android.widget.TextView;
public class MainActivity extends Activity {
public static final int DIALOG_DOWNLOAD_JSON_PROGRESS = 0;
private ProgressDialog mProgressDialog;
ArrayList<HashMap<String, Object>> MyArrList;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
// Download JSON File
new DownloadJSONFileAsync().execute();
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_DOWNLOAD_JSON_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Downloading.....");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_SPINNER);
mProgressDialog.setCancelable(true);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
// Show All Content
public void ShowAllContent()
{
// listView1
final ListView lstView1 = (ListView)findViewById(R.id.listView1);
lstView1.setAdapter(new ImageAdapter(MainActivity.this,MyArrList));
}
public class ImageAdapter extends BaseAdapter
{
private Context context;
private ArrayList<HashMap<String, Object>> MyArr = new ArrayList<HashMap<String, Object>>();
public ImageAdapter(Context c, ArrayList<HashMap<String, Object>> myArrList)
{
// TODO Auto-generated method stub
context = c;
MyArr = myArrList;
}
public int getCount() {
// TODO Auto-generated method stub
return MyArr.size();
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(final int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColImage
ImageView imageView = (ImageView) convertView.findViewById(R.id.ColImgPath);
imageView.getLayoutParams().height = 80;
imageView.getLayoutParams().width = 80;
imageView.setPadding(10, 10, 10, 10);
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
try
{
imageView.setImageBitmap((Bitmap)MyArr.get(position).get("ImageThumBitmap"));
} catch (Exception e) {
// When Error
imageView.setImageResource(android.R.drawable.ic_menu_report_image);
}
// Click on Image
imageView.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String strImageID = MyArr.get(position).get("ImageID").toString();
String strImageName = MyArr.get(position).get("ImageName").toString();
String strImagePathFull = MyArr.get(position).get("ImagePathFull").toString();
Intent newActivity = new Intent(MainActivity.this,VoteActivity.class);
newActivity.putExtra("ImageID", strImageID);
newActivity.putExtra("ImageName", strImageName);
newActivity.putExtra("ImagePathFull", strImagePathFull);
startActivity(newActivity);
}
});
// ColImgID
TextView txtImgID = (TextView) convertView.findViewById(R.id.ColImgID);
txtImgID.setPadding(5, 0, 0, 0);
txtImgID.setText("ID : " + MyArr.get(position).get("ImageID").toString());
// ColImgName
TextView txtPicName = (TextView) convertView.findViewById(R.id.ColImgName);
txtPicName.setPadding(5, 0, 0, 0);
txtPicName.setText("Name : " + MyArr.get(position).get("ImageName").toString());
// ColratingBar
RatingBar Rating = (RatingBar) convertView.findViewById(R.id.ColratingBar);
Rating.setPadding(10, 0, 0, 0);
Rating.setEnabled(false);
Rating.setMax(5);
Rating.setRating(Float.valueOf(MyArr.get(position).get("Rating").toString()));
return convertView;
}
}
// Download JSON in Background
public class DownloadJSONFileAsync extends AsyncTask<String, Void, Void> {
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
@Override
protected Void doInBackground(String... params) {
// TODO Auto-generated method stub
String url = "https://www.thaicreate.com/android/getGallery.php";
JSONArray data;
try {
data = new JSONArray(getJSONUrl(url));
MyArrList = new ArrayList<HashMap<String, Object>>();
HashMap<String, Object> map;
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, Object>();
map.put("ImageID", (String)c.getString("ImageID"));
map.put("ImageName", (String)c.getString("ImageName"));
// Thumbnail Get ImageBitmap To Object
map.put("ImagePathThum", (String)c.getString("ImagePath_Thumbnail"));
map.put("ImageThumBitmap", (Bitmap)loadBitmap(c.getString("ImagePath_Thumbnail")));
// Full (for View Popup)
map.put("ImagePathFull", (String)c.getString("ImagePath_FullPhoto"));
map.put("Rating", (String)c.getString("Rating"));
MyArrList.add(map);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
protected void onPostExecute(Void unused) {
ShowAllContent(); // When Finish Show Content
dismissDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
removeDialog(DIALOG_DOWNLOAD_JSON_PROGRESS);
}
}
/*** Get JSON Code from URL ***/
public String getJSONUrl(String url) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpGet httpGet = new HttpGet(url);
try {
HttpResponse response = client.execute(httpGet);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Download OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download file..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
/***** Get Image Resource from URL (Start) *****/
private static final String TAG = "Image";
private static final int IO_BUFFER_SIZE = 4 * 1024;
public static Bitmap loadBitmap(String url) {
Bitmap bitmap = null;
InputStream in = null;
BufferedOutputStream out = null;
try {
in = new BufferedInputStream(new URL(url).openStream(), IO_BUFFER_SIZE);
final ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
out = new BufferedOutputStream(dataStream, IO_BUFFER_SIZE);
copy(in, out);
out.flush();
final byte[] data = dataStream.toByteArray();
BitmapFactory.Options options = new BitmapFactory.Options();
//options.inSampleSize = 1;
bitmap = BitmapFactory.decodeByteArray(data, 0, data.length,options);
} catch (IOException e) {
Log.e(TAG, "Could not load Bitmap from: " + url);
} finally {
closeStream(in);
closeStream(out);
}
return bitmap;
}
private static void closeStream(Closeable stream) {
if (stream != null) {
try {
stream.close();
} catch (IOException e) {
android.util.Log.e(TAG, "Could not close stream", e);
}
}
}
private static void copy(InputStream in, OutputStream out) throws IOException {
byte[] b = new byte[IO_BUFFER_SIZE];
int read;
while ((read = in.read(b)) != -1) {
out.write(b, 0, read);
}
}
/***** Get Image Resource from URL (End) *****/
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
VoteActivity.java
package com.myapp;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.ByteArrayOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONException;
import org.json.JSONObject;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.RatingBar;
import android.widget.TextView;
import android.widget.Toast;
public class VoteActivity extends Activity {
public static final int DIALOG_DOWNLOAD_FULL_PHOTO_PROGRESS = 0;
private ProgressDialog mProgressDialog;
public String ImageID;
public String ImageName;
public String ImageFullPath;
public ArrayList<HashMap<String, Object>> MyArrList;
public RatingBar rating;
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_vote);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
Intent intent= getIntent();
ImageID = String.valueOf(intent.getStringExtra("ImageID"));
ImageName = String.valueOf(intent.getStringExtra("ImageName"));
ImageFullPath = String.valueOf(intent.getStringExtra("ImagePathFull"));
// Show Image Full
new DownloadFullPhotoFileAsync().execute();
// rating
final RatingBar rating = (RatingBar) findViewById(R.id.rating);
// Button Home
final Button home = (Button) findViewById(R.id.btnHome);
// Perform action on click
home.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent newActivity = new Intent(VoteActivity.this,MainActivity.class);
startActivity(newActivity);
}
});
final AlertDialog.Builder adb = new AlertDialog.Builder(this);
// Button btnVote
final Button vote = (Button) findViewById(R.id.btnVote);
// Perform action on click
vote.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
if(rating.getRating() <= 0)
{
AlertDialog ad = adb.create();
ad.setMessage("Please select rating point 1-5");
ad.show();
}
else
{
// Disabled Button
vote.setEnabled(false);
home.setEnabled(false);
String url = "https://www.thaicreate.com/android/updateRating.php";
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("ImageID", ImageID));
params.add(new BasicNameValuePair("ratingPoint", String.valueOf(rating.getRating())));
String resultServer = getHttpPost(url,params);
/** Get result from Server (Return the JSON Code)
* StatusID = ? [0=Failed,1=Complete]
* Error = ? [On case error return custom error message]
*
* Eg Save Failed = {"StatusID":"0","Error":"Not Update Data!"}
* Eg Save Complete = {"StatusID":"1","Error":""}
*/
/*** Default Value ***/
String strStatusID = "0";
String strError = "Unknow Status!";
JSONObject c;
try {
c = new JSONObject(resultServer);
strStatusID = c.getString("StatusID");
strError = c.getString("Error");
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Prepare Save Data
if(strStatusID.equals("0"))
{
adb.setMessage(strError);
adb.show();
// Enabled Button
vote.setEnabled(true);
home.setEnabled(true);
}
else
{
// Show Toast
Toast.makeText(VoteActivity.this, "Vote Finished (Point : " + rating.getRating() + ")", Toast.LENGTH_LONG).show();
Intent newActivity = new Intent(VoteActivity.this,MainActivity.class);
startActivity(newActivity);
}
}
}
});
}
@Override
protected void onDestroy() {
super.onDestroy();
}
// Show Image Full
public void ShowImageFull(Bitmap imgFull)
{
// fullimage
TextView imgName = (TextView) findViewById(R.id.txtImageName);
imgName.setText(ImageName);
// fullimage
ImageView image = (ImageView) findViewById(R.id.fullimage);
try {
image.setImageBitmap(imgFull);
} catch (Exception e) {
// When Error
image.setImageResource(android.R.drawable.ic_menu_report_image);
}
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DIALOG_DOWNLOAD_FULL_PHOTO_PROGRESS:
mProgressDialog = new ProgressDialog(this);
mProgressDialog.setMessage("Downloading.....");
mProgressDialog.setProgressStyle(ProgressDialog.STYLE_SPINNER);
mProgressDialog.setCancelable(true);
mProgressDialog.show();
return mProgressDialog;
default:
return null;
}
}
// Download Full Photo in Background
public class DownloadFullPhotoFileAsync extends AsyncTask<String, Void, Void> {
Bitmap ImageFullBitmap = null;
protected void onPreExecute() {
super.onPreExecute();
showDialog(DIALOG_DOWNLOAD_FULL_PHOTO_PROGRESS);
}
@Override
protected Void doInBackground(String... params) {
ImageFullBitmap = (Bitmap)loadBitmap(ImageFullPath);
return null;
}
protected void onPostExecute(Void unused) {
ShowImageFull(ImageFullBitmap); // When Finish Show Images
dismissDialog(DIALOG_DOWNLOAD_FULL_PHOTO_PROGRESS);
removeDialog(DIALOG_DOWNLOAD_FULL_PHOTO_PROGRESS);
}
}
public String getHttpPost(String url,List<NameValuePair> params) {
StringBuilder str = new StringBuilder();
HttpClient client = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.setEntity(new UrlEncodedFormEntity(params));
HttpResponse response = client.execute(httpPost);
StatusLine statusLine = response.getStatusLine();
int statusCode = statusLine.getStatusCode();
if (statusCode == 200) { // Status OK
HttpEntity entity = response.getEntity();
InputStream content = entity.getContent();
BufferedReader reader = new BufferedReader(new InputStreamReader(content));
String line;
while ((line = reader.readLine()) != null) {
str.append(line);
}
} else {
Log.e("Log", "Failed to download result..");
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return str.toString();
}
/***** Get Image Resource from URL (Start) *****/
private static final String TAG = "Image";
private static final int IO_BUFFER_SIZE = 4 * 1024;
public static Bitmap loadBitmap(String url) {
Bitmap bitmap = null;
InputStream in = null;
BufferedOutputStream out = null;
try {
in = new BufferedInputStream(new URL(url).openStream(), IO_BUFFER_SIZE);
final ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
out = new BufferedOutputStream(dataStream, IO_BUFFER_SIZE);
copy(in, out);
out.flush();
final byte[] data = dataStream.toByteArray();
BitmapFactory.Options options = new BitmapFactory.Options();
//options.inSampleSize = 1;
bitmap = BitmapFactory.decodeByteArray(data, 0, data.length,options);
} catch (IOException e) {
Log.e(TAG, "Could not load Bitmap from: " + url);
} finally {
closeStream(in);
closeStream(out);
}
return bitmap;
}
private static void closeStream(Closeable stream) {
if (stream != null) {
try {
stream.close();
} catch (IOException e) {
android.util.Log.e(TAG, "Could not close stream", e);
}
}
}
private static void copy(InputStream in, OutputStream out) throws IOException {
byte[] b = new byte[IO_BUFFER_SIZE];
int read;
while ((read = in.read(b)) != -1) {
out.write(b, 0, read);
}
}
/***** Get Image Resource from URL (End) *****/
}
เพิ่ม VoteActivity ลงในไฟล์ AndroidManifest.xml
<activity
android:name=".VoteActivity"
android:theme="@style/AppTheme"
android:screenOrientation="portrait"
android:label="@string/title_activity_main" />
Screenshot
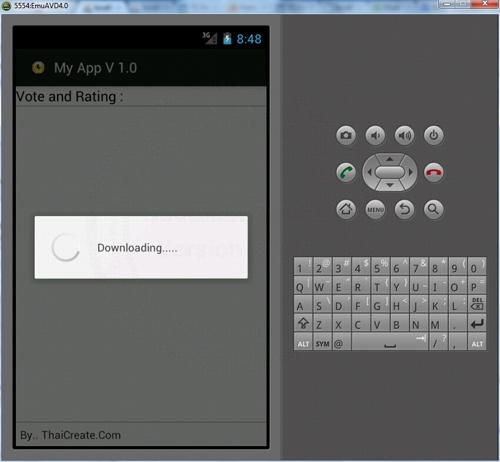
กำลังแสดงข้อมูลจาก Web Server บน ListView
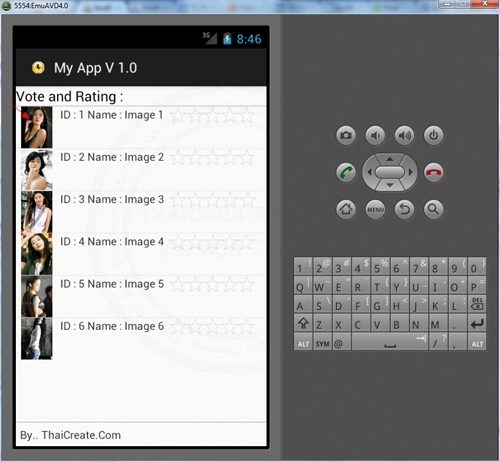
แสดงข้อมูลบน ListView หลังจากที่ได้โหลดเรียบร้อยแล้ว จะเห็นว่าตอนนี้ข้อมูลคะแนน Point ของ RatingBar จะยังเป็น 0 ให้คลิกเพื่อดูรายละเอียด
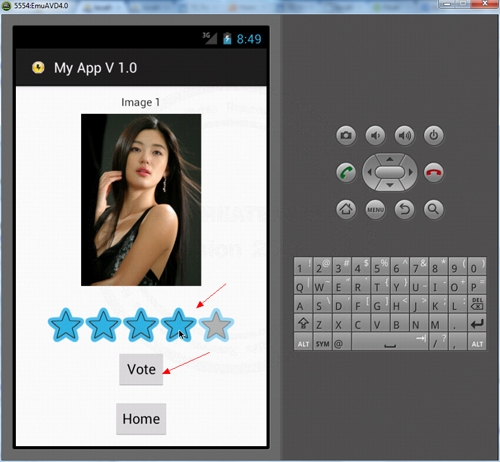
คลิกที่ RatingBar เพื่อโหวด และคลิกเลือก ที่ปุ่ม Vote เพื่อส่งคะแนนไปยัง Web Server
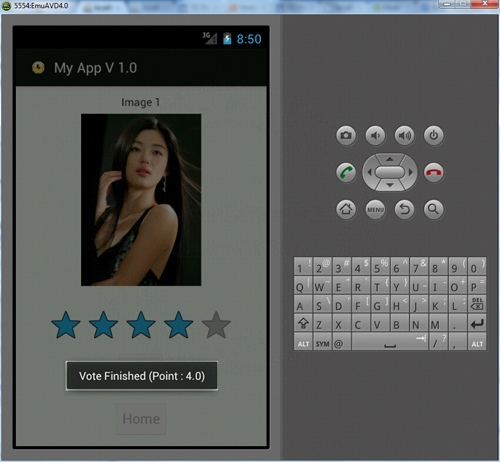
หลังจากที่โหวดเรียบร้อยแล้ว
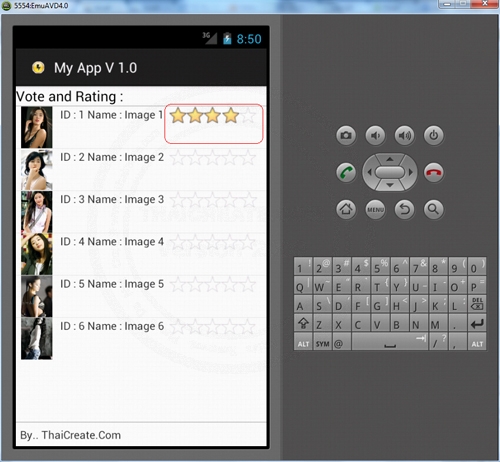
เมื่อกลับมาที่หน้าจอของ ListView ก็จะเห็นว่า RatingBar ได้ขึ้น Point ตามที่ได้โหวตไว้

และเมื่อเปิดดู phpMyAdmin บนฝั่ง Web Server ก็จะเห็นว่ารายการนั้น ๆ ได้ถูก Update ตัว Rating
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-08-26 12:18:27 /
2017-03-26 22:38:34 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|