ตอนที่ 6 : Show Case 2 : Login User Password (iOS C# (Xamarin.iOS) and Mobile Services) |
ตอนที่ 6 : Show Case 2 : Login User Password (iOS C# (Xamarin.iOS) and Mobile Services) หลังจากบทความก่อนหน้านี้เราได้ทำระบบ Register Form บน iOS C# (Xamarin.iOS) และส่งข้อมูลไปเก็บไว้ที่ Azure Mobile Services ไปเรียบร้อยแล้ว บทความนี้จะเป็นการทำระบบ Login Form ด้วย Username และ Password โดยข้อมูลและ Table ถูกจัดเก็บไว้ที่ Mobile Services บน Windows Azure และหงัจากที่ Login ผ่านแล้ว เราจะดึงข้อมูลของ User ที่ Login มาแสดงในหน้า Info
iOS C# (Xamarin) Mobile Services Login Form
รูปแบบการตรวจสอบ Username และ Password
partial void btnLogin_TouchUpInside(UIButton sender)
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
memberTable = client.GetTable<MyMember>();
var data = memberTable.Where(item => item.Username == txtUsername.Text
&& item.Password == this.txtPassword.Text).ToListAsync();
if (data.Count == 0)
{
new UIAlertView("Result", "Incorrect Username and Password!", null, "OK", null).Show();
}
else
{
loginFlag = true;
}
}
เงื่อนไขการเปลี่ยน View หรือ Action ของ Segue
public override void PrepareForSegue(UIStoryboardSegue segue, NSObject sender)
{
if (loginFlag)
{
((DetailViewController)segue.DestinationViewController).SetDetailItem(this.txtUsername.Text);
}
}
public override bool ShouldPerformSegue(string segueIdentifier, NSObject sender)
{
if (!loginFlag)
{
return false;
}
else
{
return true;
}
}
รูปแบบการแสดง Member Info
object detailItem;
public void SetDetailItem(object newDetailItem)
{
if (detailItem != newDetailItem)
{
detailItem = newDetailItem;
}
}
private void LoadInfo()
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
string strUsername = detailItem.ToString();
memberTable = client.GetTable<MyMember>();
var info = memberTable.Where(item => item.Username == strUsername).ToCollectionAsync();
if (info.Count > 0)
{
MyMember member = info[0];
lblUsername.Text = member.Username.ToString();
lblPassword.Text = member.Password.ToString();
lblName.Text = member.Name.ToString();
lblEmail.Text = member.Email.ToString();
lblTel.Text = member.Tel.ToString();
}
}
Example ตัวอย่างการทำระบบล็อดอิน Member Login และแสดง Member Information
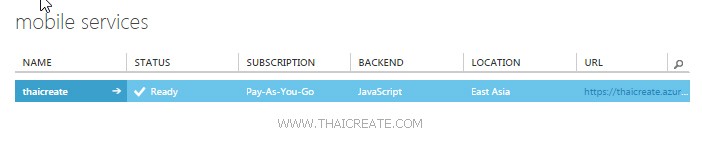
ตอนนี้เรามี Mobile Services อยู่ 1 รายการ
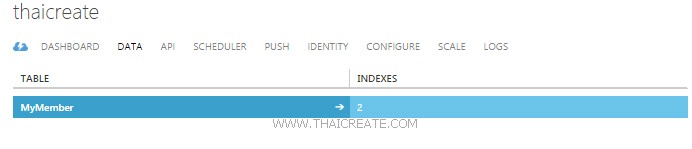
ชื่อตารางว่า MyMember
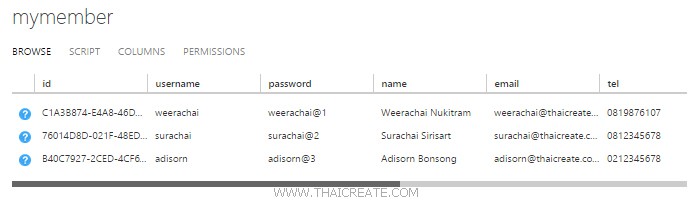
มีข้อมูลอยู่ 3 รายการ
กลับมายัง iOS C# Project บนโปรแกรม Visual Studio
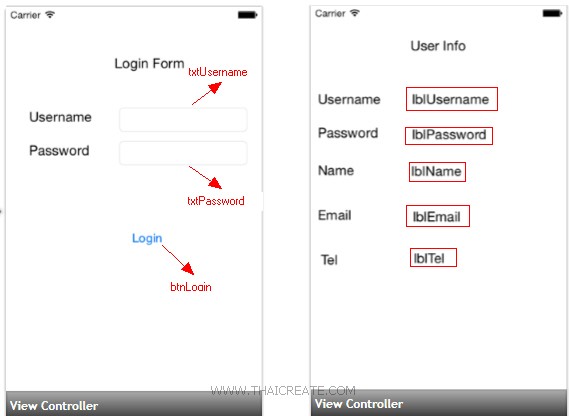
สำหรับขั้นตอนนั้นก็คือสร้าง View สำหรับตรวจสอบ Username และ Password และเมื่อ Login ผ่านก็จะทำการแสดงไปยังอีก View เพื่อแสดงข้อมูลของ User นั้น ๆ ที่ได้ทำการ Login เข้ามาในระบบ
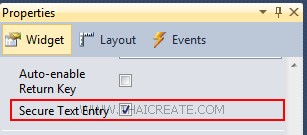
สำหรับ Password Box สามารถกำหนดค่าได้ที่ Properties -> Secure Text Entry
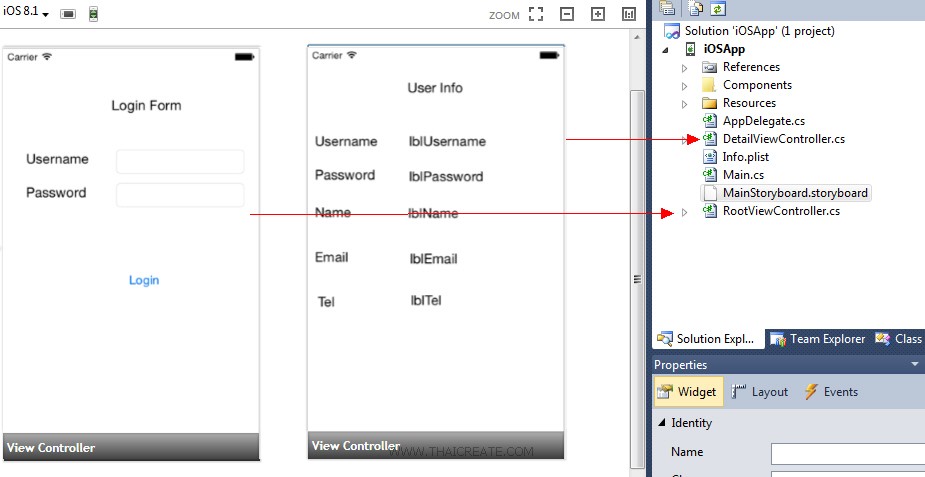
เรามีไฟล์ View และ Class อยู่ 2 ชุดคือ
- View แสดง Login กับ RootViewController.csเป็นไฟล์ที่ทำหน้าที่แสดงหน้า Login และตรวจสอบ Username / Password
- View แสดง Info และ DetailViewController.cs แสดง Member Info และรายละเอียดของ Member ที่ Login เข้ามาในระบบ
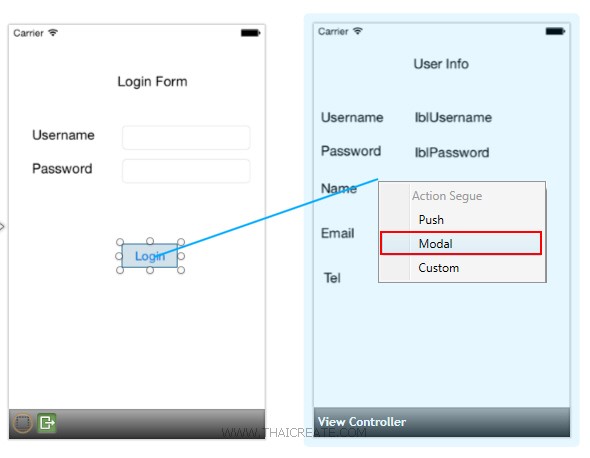
สร้าง Action ของ Segue ใน View แรกด้วยการคลิกที่ปุ่ม Login และกด Ctrl บน Keyboard แล้วลากเส้นไปวางใน View ที่สอง เลือก Action แบบ Modal
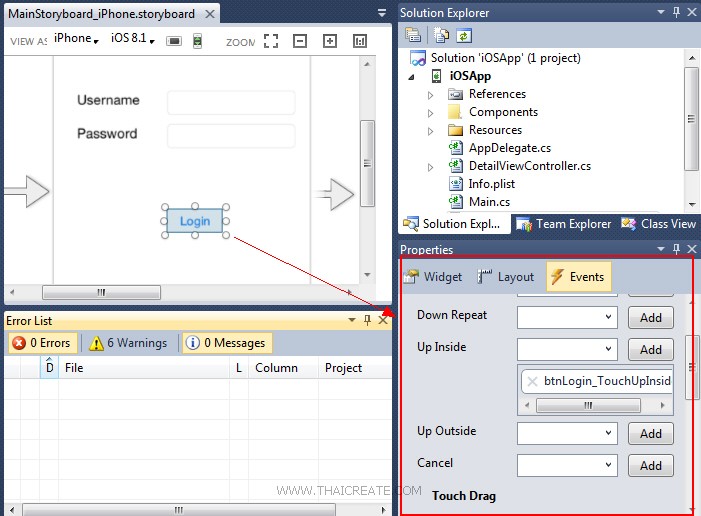
สร้าง Event ของ Button การ Login
RootViewController.cs
using System;
using System.Drawing;
using System.Collections.Generic;
using MonoTouch.Foundation;
using MonoTouch.UIKit;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
namespace iOSApp
{
public class MyMember
{
public int Id { get; set; }
[JsonProperty(PropertyName = "username")]
public string Username { get; set; }
[JsonProperty(PropertyName = "password")]
public string Password { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "email")]
public string Email { get; set; }
[JsonProperty(PropertyName = "tel")]
public string Tel { get; set; }
}
public partial class RootViewController : UIViewController
{
public const string ApplicationURL = @"https://thaicreate.azure-mobile.net/";
public const string ApplicationKey = @"IqeWShAjBflTUrTaaGUNJRyZDpcyeh72";
private MobileServiceClient client; // Mobile Service Client references
private IMobileServiceTable<MyMember> memberTable; // Mobile Service Table used to access data
private bool loginFlag = false;
public RootViewController(IntPtr handle)
: base(handle)
{
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
}
public override void DidReceiveMemoryWarning()
{
// Releases the view if it doesn't have a superview.
base.DidReceiveMemoryWarning();
}
partial void btnLogin_TouchUpInside(UIButton sender)
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
memberTable = client.GetTable<MyMember>();
var data = memberTable.Where(item => item.Username == txtUsername.Text
&& item.Password == this.txtPassword.Text).ToListAsync();
if (data.Count == 0)
{
new UIAlertView("Result", "Incorrect Username and Password!", null, "OK", null).Show();
}
else
{
loginFlag = true;
}
}
public override void PrepareForSegue(UIStoryboardSegue segue, NSObject sender)
{
if (loginFlag)
{
((DetailViewController)segue.DestinationViewController).SetDetailItem(this.txtUsername.Text);
}
}
public override bool ShouldPerformSegue(string segueIdentifier, NSObject sender)
{
if (!loginFlag)
{
return false;
}
else
{
return true;
}
}
}
}

DetailViewController.cs
using System;
using System.Drawing;
using MonoTouch.Foundation;
using MonoTouch.UIKit;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
namespace iOSApp
{
public partial class DetailViewController : UIViewController
{
public const string ApplicationURL = @"https://thaicreate.azure-mobile.net/";
public const string ApplicationKey = @"IqeWShAjBflTUrTaaGUNJRyZDpcyeh72";
private MobileServiceClient client; // Mobile Service Client references
private IMobileServiceTable<MyMember> memberTable; // Mobile Service Table used to access data
object detailItem;
public void SetDetailItem(object newDetailItem)
{
if (detailItem != newDetailItem)
{
detailItem = newDetailItem;
}
}
public DetailViewController(IntPtr handle)
: base(handle)
{
}
public override void DidReceiveMemoryWarning()
{
// Releases the view if it doesn't have a superview.
base.DidReceiveMemoryWarning();
// Release any cached data, images, etc that aren't in use.
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.LoadInfo();
}
private void LoadInfo()
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
string strUsername = detailItem.ToString();
memberTable = client.GetTable<MyMember>();
var info = memberTable.Where(item => item.Username == strUsername).ToCollectionAsync();
if (info.Count > 0)
{
MyMember member = info[0];
lblUsername.Text = member.Username.ToString();
lblPassword.Text = member.Password.ToString();
lblName.Text = member.Name.ToString();
lblEmail.Text = member.Email.ToString();
lblTel.Text = member.Tel.ToString();
}
}
}
}
ทดสอบการทำงาน
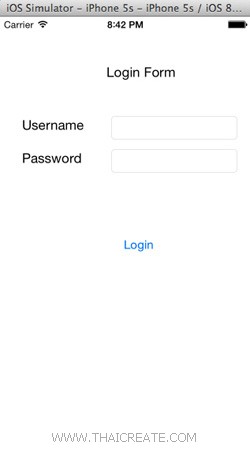
Login Form บน iOS Simulator
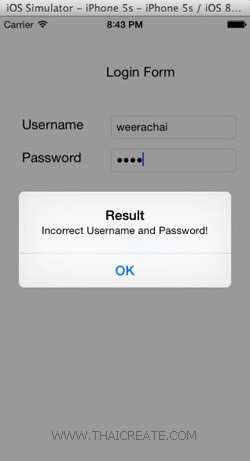
กรณีที่ Login ข้อมูล Username และ Password ผิดพลาด
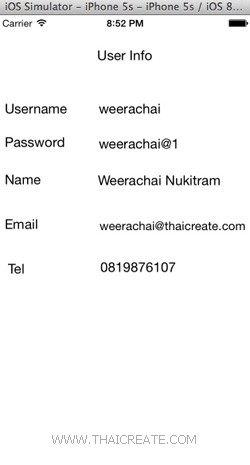
กรณีที่ Login ถูกต้อง จะแสดง Information ของสมาชิกที่ Login เข้ามาในระบบ
อ่านเพิ่มเติม
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-10-24 14:02:34 /
2017-03-26 08:45:36 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|