ตอนที่ 14 : Show Case 2 : Login User Password (iOS / iPhone and Mobile Services) |
ตอนที่ 14 : Show Case 2 : Login User Password (iOS / iPhone and Mobile Services) หลังจากบทความก่อนหน้านี้เราได้ทำระบบ Register Form บน iOS และ iPhone และส่งข้อมูลไปเก็บไว้ที่ Mobile Services ไปเรียบร้อยแล้ว บทความนี้จะเป็นการทำระบบ Login Form ด้วย Username และ Password โดยข้อมูลและ Table ถูกจัดเก็บไว้ที่ Mobile Services บน Windows Azure
iOS / iPhone Mobile Services Login Form
สำหรับขั้นตอนนั้นก็คือสร้าง View สำหรับตรวจสอบ Username และ Password และเมื่อ Login ผ่านก็จะทำการ Redirect ไปยังอีก View เพื่อแสดงข้อมูลของ User นั้น ๆ ที่ได้ทำการ Login เข้ามาในระบบ
Example ตัวอย่างการทำระบบล็อกอิน Member Login Form บน iOS / Mobile Services

ตอนนี้เรามี Mobile Services อยู่ 1 รายการ
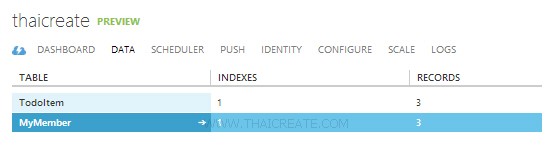
ชื่อตารางว่า MyMember
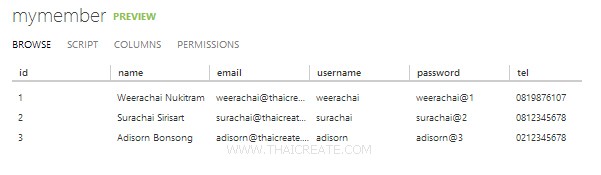
มีข้อมูลอยู่ 3 รายการ
กลับมายัง Project ของ iOS บน Xcode
สำหรับพื้นฐานการสร้าง Project และการสร้าง Table รวมทั้งการติดต่อระหว่าง iOS กับ Mobile Services บน Windows Azure แนะนำให้อ่านบทความในตอนที่ 5
ตอนที่ 5: iOS / iPhone สร้างตาราง Table บน Mobile Services และการ Insert ข้อมูล
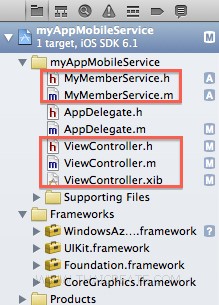
โครงสร้างไฟล์
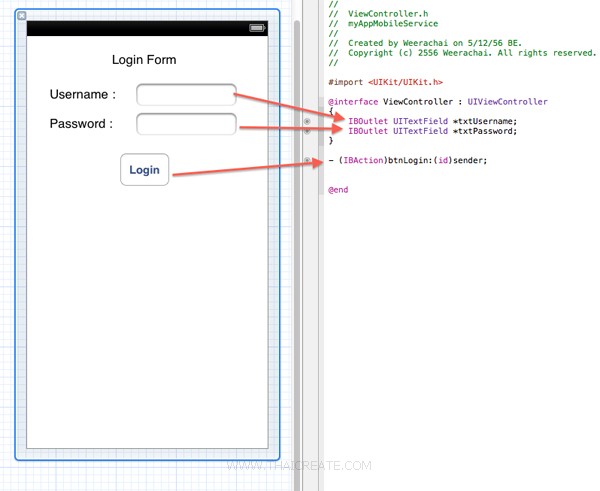
View แรกจะชื่อ ViewController.xib, ViewController.h และ ViewController.m ซึ่งไว้สำหรับ Login ตัว Username และ Password
ในไฟล์ ViewController.h และ ViewController.m เขียน Code ดังนี้
ViewController.h
//
// ViewController.h
// myAppMobileService
//
// Created by Weerachai on 5/12/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
{
IBOutlet UITextField *txtUsername;
IBOutlet UITextField *txtPassword;
}
- (IBAction)btnLogin:(id)sender;
@end
ViewController.m
//
// ViewController.m
// myAppMobileService
//
// Created by Weerachai on 5/12/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import "MyMemberService.h"
#import "ViewController.h"
#import "LoginInfoViewController.h"
@interface ViewController ()
// Private properties
@property (strong, nonatomic) MyMemberService *memberService;
@end
@implementation ViewController
@synthesize memberService;
- (void)viewDidLoad
{
[super viewDidLoad];
// Start Service
self.memberService = [MyMemberService defaultService];
// Tap Gesture for Hide Keyboard
UITapGestureRecognizer *oneTapGesture = [[UITapGestureRecognizer alloc]
initWithTarget: self
action: @selector(hideKeyboard:)];
[oneTapGesture setNumberOfTouchesRequired:1];
[[self view] addGestureRecognizer:oneTapGesture];
}
// Event Gesture for Hide Keyboard
- (void)hideKeyboard:(UITapGestureRecognizer *)sender {
[txtPassword resignFirstResponder];
[txtPassword resignFirstResponder];
}
- (IBAction)btnLogin:(id)sender {
NSDictionary *chk = @{ @"user":txtUsername.text, @"pass":txtPassword.text };
[self.memberService checkLogin:chk completion:^
{
if(memberService.items.count ==0)
{
UIAlertView *av =
[[UIAlertView alloc]
initWithTitle:@"Login Status"
message:@"Login Failed."
delegate:nil
cancelButtonTitle:@"OK"
otherButtonTitles:nil
];
[av show];
}
else
{
/*
UIAlertView *av =
[[UIAlertView alloc]
initWithTitle:@"Login Status"
message:@"Login OK."
delegate:nil
cancelButtonTitle:@"OK"
otherButtonTitles:nil
];
[av show];
*/
LoginInfoViewController *viewInfo = [[[LoginInfoViewController alloc] initWithNibName:nil bundle:nil] autorelease];
NSDictionary *item = [self.memberService.items objectAtIndex:0];
viewInfo.sid = [item objectForKey:@"id"];
[self presentViewController:viewInfo animated:NO completion:NULL];
}
}];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[txtUsername release];
[txtPassword release];
[super dealloc];
}
@end
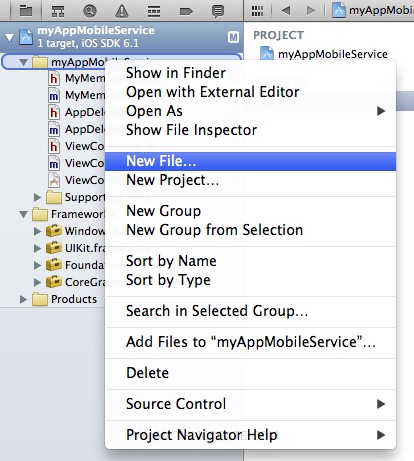
เราจะสร้างชุดของ View และ Class ไว้สำหรับ แสดงรายละเอียด หลังจากที่ได้ Login เรียบร้อยแล้ว โดยใช้การส่งค่าผ่าน View เริ่มการสร้างโดยคลิกขวาที่ Project เลือก New
iOS/iPhone Passing Data Between View (Objective-C,iPhone,iPad)
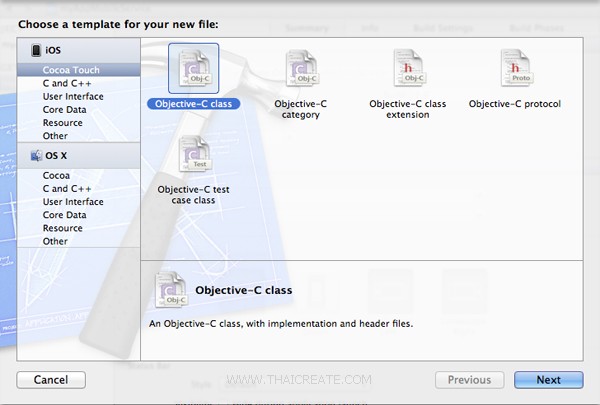
เลือกเป็น Objective-C Class
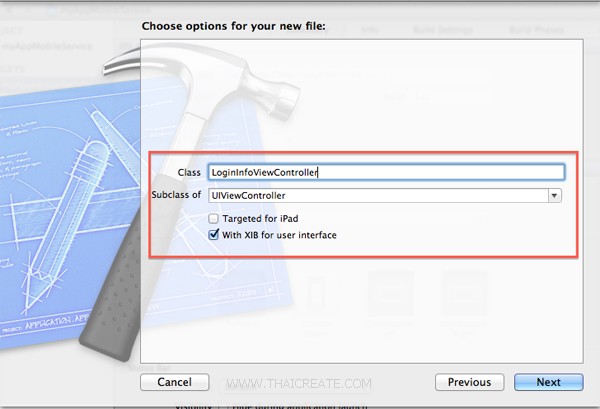
ตั้งชื่อว่า LoginInfoViewController โดยให้เลือก Subclass เป็นว่า UIViewController และอย่าลืมเลือก [x] Width XIB for user Interface
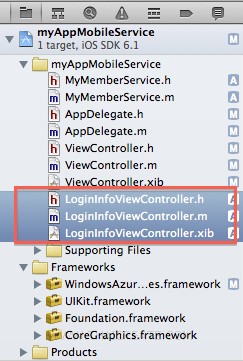
เราจะได้ไฟล์ขึ้นมา 1 ชุด LoginInfoViewController.xib , LoginInfoViewController.h และ LoginInfoViewController.m
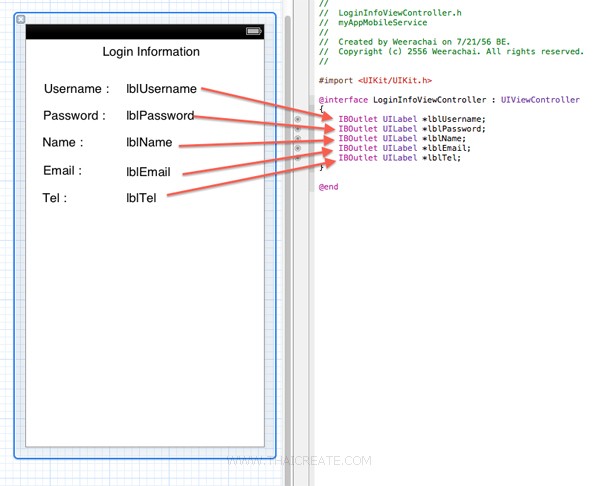
สร้าง Label ต่าง ๆ และ ทำการเชื่อม IBOutlet ให้เรียบร้อย
ในไฟล์ LoginInfoViewController.h และ LoginInfoViewController.m เขียน Code ดังนี้
LoginInfoViewController.h
//
// LoginInfoViewController.h
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface LoginInfoViewController : UIViewController
{
IBOutlet UILabel *lblUsername;
IBOutlet UILabel *lblPassword;
IBOutlet UILabel *lblName;
IBOutlet UILabel *lblEmail;
IBOutlet UILabel *lblTel;
}
@property (strong, nonatomic) id sid;
@end
LoginInfoViewController.m
//
// LoginInfoViewController.m
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import "MyMemberService.h"
#import "LoginInfoViewController.h"
@interface LoginInfoViewController ()
// Private properties
@property (strong, nonatomic) MyMemberService *memberService;
@end
@implementation LoginInfoViewController
@synthesize memberService;
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view from its nib.
// Start Service
self.memberService = [MyMemberService defaultService];
NSString *wid = [NSString stringWithFormat:@"id = %@",[self.sid description]];
[self.memberService getUserInfo:wid completion:^
{
if(memberService.items.count > 0)
{
NSDictionary *item = [self.memberService.items objectAtIndex:0];
lblUsername.text = [item objectForKey:@"username"];
lblPassword.text = [item objectForKey:@"password"];
lblName.text = [item objectForKey:@"name"];
lblEmail.text = [item objectForKey:@"email"];
lblTel.text = [item objectForKey:@"tel"];
}
else
{
UIAlertView *av =
[[UIAlertView alloc]
initWithTitle:@"Get Data"
message:@"Not found data."
delegate:nil
cancelButtonTitle:@"OK"
otherButtonTitles:nil
];
[av show];
}
}];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[lblUsername release];
[lblPassword release];
[lblName release];
[lblTel release];
[lblName release];
[super dealloc];
}
@end
Screenshot
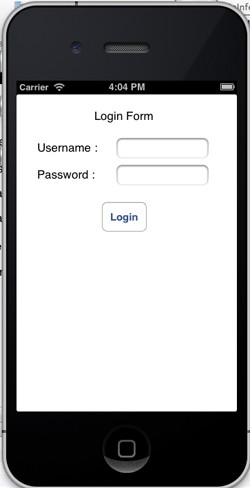
หน้า Login บน iOS / iPhone ซึ่งจะตรวจสอบ Username และ Password ที่อยู่บน Mobile Services
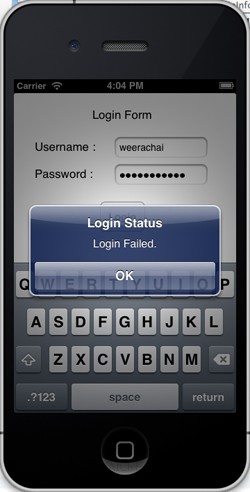
กรณีที่ Login ไม่ถูกต้อง
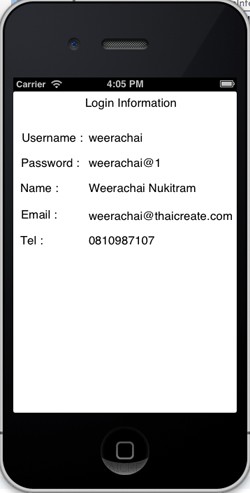
กรณีที่ Login ถูกต้องจะแสดงรายละเอียดของ User นั้น ๆ ที่อ่านข้อมูลจาก Mobile Services ของ Windows Azure
อันนี้ Code ในไฟล์ MyMemberService.h และ MyMemberService.m
MyMemberService.h
//
// MyMemberService.h
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
#import <Foundation/Foundation.h>
typedef void (^QSCompletionBlock) ();
typedef void (^QSBusyUpdateBlock) (BOOL busy);
@interface MyMemberService : NSObject
@property (nonatomic, strong) NSArray *items;
@property (nonatomic, strong) MSClient *client;
@property (nonatomic, copy) QSBusyUpdateBlock busyUpdate;
+ (MyMemberService *)defaultService;
- (void)checkLogin:(NSDictionary *)item
completion:(QSCompletionBlock)completion;
- (void)getUserInfo:(NSString *)sid
completion:(QSCompletionBlock)completion;
- (void)handleRequest:(NSURLRequest *)request
next:(MSFilterNextBlock)next
response:(MSFilterResponseBlock)response;
@end
MyMemberService.m
//
// MyMemberService.m
// myAppMobileService
//
// Created by Weerachai on 7/21/56 BE.
// Copyright (c) 2556 Weerachai. All rights reserved.
//
#import "MyMemberService.h"
#import <WindowsAzureMobileServices/WindowsAzureMobileServices.h>
@interface MyMemberService() <MSFilter>
@property (nonatomic, strong) MSTable *table;
@property (nonatomic) NSInteger busyCount;
@end
@implementation MyMemberService
@synthesize items;
+ (MyMemberService *)defaultService
{
// Create a singleton instance of MyMemberService
static MyMemberService* service;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
service = [[MyMemberService alloc] init];
});
return service;
}
-(MyMemberService *)init
{
self = [super init];
if (self)
{
// Initialize the Mobile Service client with your URL and key
MSClient *client = [MSClient clientWithApplicationURLString:@"https://thaicreate.azure-mobile.net/"
applicationKey:@"uJPAhkAkcTBuTyCNxaOSnDKFzkoYqB49"];
// Add a Mobile Service filter to enable the busy indicator
self.client = [client clientWithFilter:self];
// Create an MSTable instance to allow us to work with the TodoItem table
self.table = [_client tableWithName:@"MyMember"];
self.items = [[NSMutableArray alloc] init];
self.busyCount = 0;
}
return self;
}
-(void)checkLogin:(NSDictionary *)item completion:(QSCompletionBlock)completion
{
// Create a predicate that finds items where username & password
NSPredicate * predicate = [NSPredicate predicateWithFormat:@"Username == %@ and Password == %@",
[item objectForKey:@"user"],
[item objectForKey:@"pass"]];
// Query the TodoItem table and update the items property with the results from the service
[self.table readWithPredicate:predicate completion:^(NSArray *results, NSInteger totalCount, NSError *error)
{
[self logErrorIfNotNil:error];
items = [results mutableCopy];
// Let the caller know that we finished
completion();
}];
}
-(void)getUserInfo:(NSString *)sid completion:(QSCompletionBlock)completion
{
// Create a predicate that finds items where username & password
NSPredicate * predicate = [NSPredicate predicateWithFormat:sid];
// Query the TodoItem table and update the items property with the results from the service
[self.table readWithPredicate:predicate completion:^(NSArray *results, NSInteger totalCount, NSError *error)
{
[self logErrorIfNotNil:error];
items = [results mutableCopy];
// Let the caller know that we finished
completion();
}];
}
- (void)busy:(BOOL)busy
{
// assumes always executes on UI thread
if (busy)
{
if (self.busyCount == 0 && self.busyUpdate != nil)
{
self.busyUpdate(YES);
}
self.busyCount ++;
}
else
{
if (self.busyCount == 1 && self.busyUpdate != nil)
{
self.busyUpdate(FALSE);
}
self.busyCount--;
}
}
- (void)logErrorIfNotNil:(NSError *) error
{
if (error)
{
NSLog(@"ERROR %@", error);
}
}
- (void)handleRequest:(NSURLRequest *)request
next:(MSFilterNextBlock)next
response:(MSFilterResponseBlock)response
{
// A wrapped response block that decrements the busy counter
MSFilterResponseBlock wrappedResponse = ^(NSHTTPURLResponse *innerResponse, NSData *data, NSError *error)
{
[self busy:NO];
response(innerResponse, data, error);
};
// Increment the busy counter before sending the request
[self busy:YES];
next(request, wrappedResponse);
}
@end
สามารถทำการดาวน์โหลด Code ทั้งหมดได้จากท้ายบทความ
บทความถัดไปที่แนะนำให้อ่าน
บทความที่เกี่ยวข้อง
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2013-06-15 19:52:18 /
2017-03-24 11:57:29 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|