iOS/iPhone Table View and JSON (UITableView from JSON Parser) |
iOS/iPhone Table View and JSON (UITableView from JSON Parser) บทความก่อนหน้านี้เราได้เรียนรู้การเขียน iOS กับ JSON แล้ว และต่อไปนี้เราจะมาประยุกต์การใช้งาน JSON กับ Table View (UITableView) ด้วยการอ่านข้อมูล JSON ที่อยู่บน Web Server ผ่าน URL (Website) จากนั้นนำค่า JSON ทีได้มาทำการ DeCode หรือ Parser ให้อยู่ในรูปแบบของ Array และนำ Array ที่ได้แสดงผลบน Table View (UITableView)
iOS/iPhone Table View and JSON (UITableView from JSON Parser)
ในการแปลงข้อมูลจาก JSON ให้อยู่ในรูปแบบของ Array ซึ่งประกอบด้วยข้อมูลที่มีหลาย Index และหลายมิติ ข้อมูล เราจะใช้ NSMutableArray และ NSDictionary มาทำการจัดเก็บข้อมูลเหล่านี้ ซึ่งบทความต่าง ๆ สามารถอ่านจากตัวอย่างในส่วนของ Table View และ NSDictionary
iOS/iPhone Table View from NSDictionary - UITableview and NSMutableDictionary
iOS/iPhone and JSON (Create JSON and JSON Parsing, Objective-C)
รูปแบบการอ่าน JSON ให้อยู่ในรูปแบบ Array ด้วย NSMutableArray และ NSDictionary
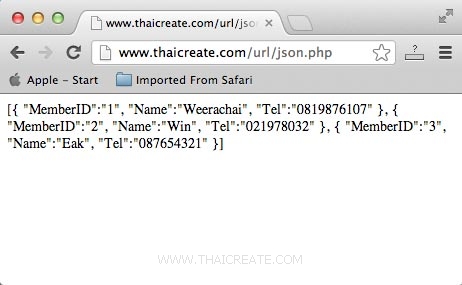
https://www.thaicreate.com/url/json.php
URL ของ JSON Code ซึ่งมีข้อความ JSON ดังนี้
[{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }, { "MemberID":"2", "Name":"Win", "Tel":"021978032" }, { "MemberID":"3", "Name":"Eak", "Tel":"087654321" }]
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *memberid;
NSString *name;
NSString *tel;
// Define keys
memberid = @"MemberID";
name = @"Name";
tel = @"Tel";
// [{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }, { "MemberID":"2", "Name":"Win", "Tel":"021978032" }, { "MemberID":"3", "Name":"Eak", "Tel":"087654321" }]
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
NSData *jsonData = [NSData dataWithContentsOfURL:
[NSURL URLWithString:@"https://www.thaicreate.com/url/json.php"]];
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strMemberID = [dataDict objectForKey:@"MemberID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTel = [dataDict objectForKey:@"Tel"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strMemberID, memberid,
strName, name,
strTel, tel,
nil];
[myObject addObject:dict];
}
จะได้ชุดของข้อมูล NSMutableArray ที่สามารถไปใช้กับ Table View แล้ว
Example การนำ JSON ไปใช้กับ Table View (UITableView)
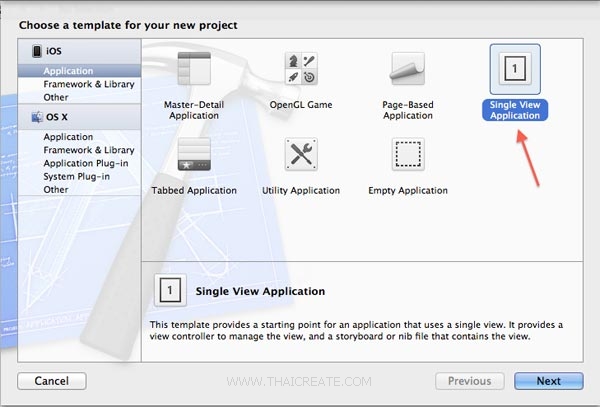
เริ่มต้นด้วยการสร้าง Application บน Xcode แบบ Single View Application
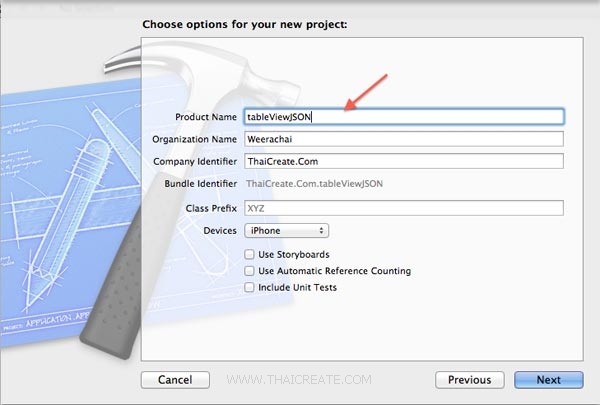
เลือกและไม่เลือกดังรูป
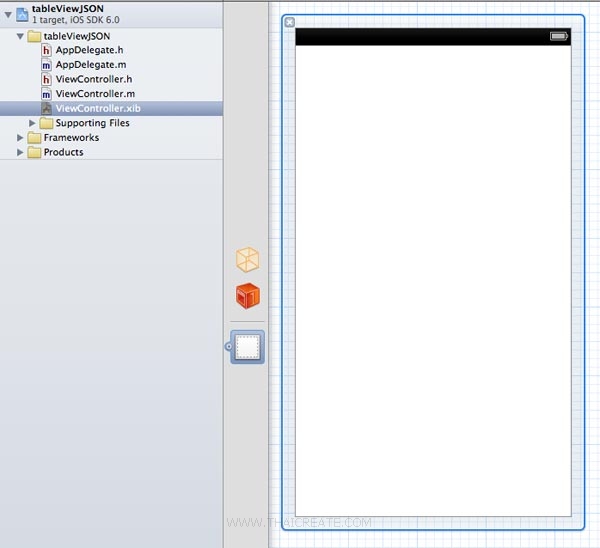
ตอนนี้หน้าจอ View ของเราจะยังว่าง ๆ
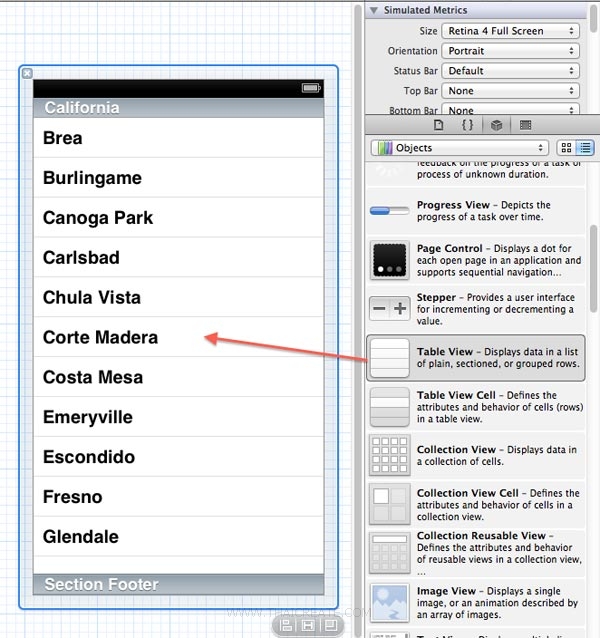
ให้ลาก Table View มาวางไว้ในหน้าจอ View
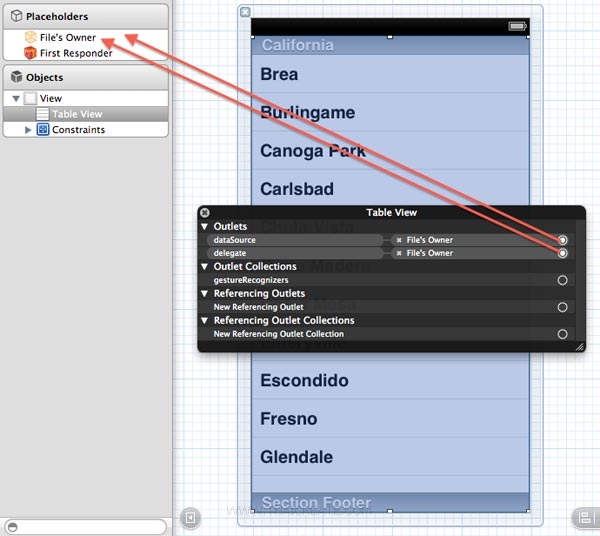
คลิกวาที่ Table View ให้ทำการเชื่อม dataSource และ delegate กับ File's Owner
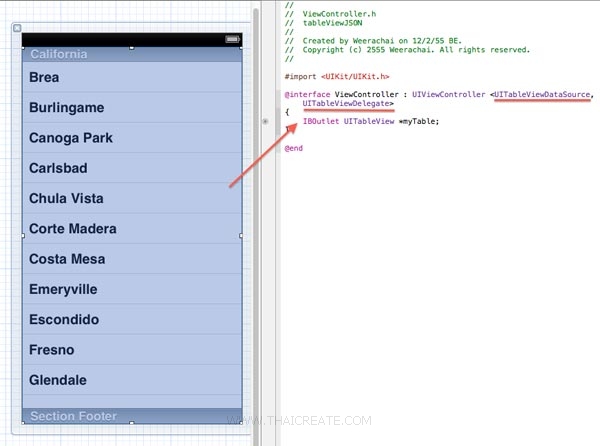
ใน Class ของ .h ให้ delegate ดังนี้ <UITableViewDataSource,UITableViewDelegate> และให้เชื่อม IBOutlet ของ Table View ให้เรียบร้อย
จากนั้นก็จะเป็นส่วนของ Code ซึ่งมีทั้งหมดดังนี้
ViewController.h
//
// ViewController.h
// tableViewJSON
//
// Created by Weerachai on 12/2/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController <UITableViewDataSource,UITableViewDelegate>
{
IBOutlet UITableView *myTable;
}
@end
ViewController.m
//
// ViewController.m
// tableViewJSON
//
// Created by Weerachai on 12/2/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
{
NSMutableArray *myObject;
// A dictionary object
NSDictionary *dict;
// Define keys
NSString *memberid;
NSString *name;
NSString *tel;
}
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
// Define keys
memberid = @"MemberID";
name = @"Name";
tel = @"Tel";
// [{ "MemberID":"1", "Name":"Weerachai", "Tel":"0819876107" }, { "MemberID":"2", "Name":"Win", "Tel":"021978032" }, { "MemberID":"3", "Name":"Eak", "Tel":"087654321" }]
// Create array to hold dictionaries
myObject = [[NSMutableArray alloc] init];
NSData *jsonData = [NSData dataWithContentsOfURL:
[NSURL URLWithString:@"https://www.thaicreate.com/url/json.php"]];
id jsonObjects = [NSJSONSerialization JSONObjectWithData:jsonData options:NSJSONReadingMutableContainers error:nil];
// values in foreach loop
for (NSDictionary *dataDict in jsonObjects) {
NSString *strMemberID = [dataDict objectForKey:@"MemberID"];
NSString *strName = [dataDict objectForKey:@"Name"];
NSString *strTel = [dataDict objectForKey:@"Tel"];
dict = [NSDictionary dictionaryWithObjectsAndKeys:
strMemberID, memberid,
strName, name,
strTel, tel,
nil];
[myObject addObject:dict];
}
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return myObject.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
// Use the default cell style.
cell = [[[UITableViewCell alloc] initWithStyle : UITableViewCellStyleSubtitle
reuseIdentifier : CellIdentifier] autorelease];
}
NSDictionary *tmpDict = [myObject objectAtIndex:indexPath.row];
// MemberID
NSMutableString *text;
text = [NSString stringWithFormat:@"MemberID : %@",[tmpDict objectForKey:memberid]];
// Name & Tel
NSMutableString *detail;
detail = [NSString stringWithFormat:@"Name : %@ , Tel : %@"
,[tmpDict objectForKey:name]
,[tmpDict objectForKey:tel]];
cell.textLabel.text = text;
cell.detailTextLabel.text= detail;
//[tmpDict objectForKey:memberid]
//[tmpDict objectForKey:name]
//[tmpDict objectForKey:tel]
return cell;
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[myTable release];
[super dealloc];
}
@end
สำหรับ Code ทั้งหมดไม่ยากอะไรมากสามารถอ่านเกี่ยวกับพื้นฐาน Table View ได้ตามบทความนี้
iOS/iPhone TableView and UITableView (Objective-C, iPhone, iPad)
Screenshot
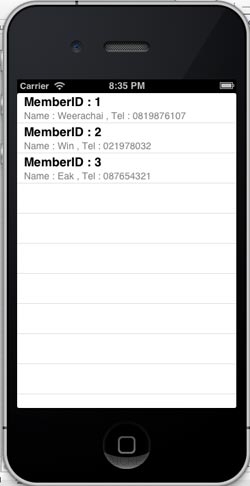
แสดงข้อมูลบน Table View ที่ได้จาก JSON ที่อยู่บน Web Server / URL (Website)
|