ตอนที่ 1 : การสร้าง Azure Mobile Services ทำงานร่วมกับ HTML และ JavaScript |
ตอนที่ 1 : การสร้าง Mobile Services ทำงานร่วมกับ HTML และ JavaScript อีกความสามารถหนึ่งของ Mobile Services ที่อยู่บน Windows Azure ก็คือ Mobile Services มี API ที่ทำงานร่วมกับ jQuery สามารถเขียนใช้ได้กับ HTML และ JavaScript แสดงผลผ่าน Web Browser โดยอาจจะใช้พวก Library ที่เป็น UI เกี่ยวกับ Mobile อย่างเช่น jQuery Mobile , Foundation Mobile Framework และสามารถใช้งานร่วมกับ Mobile Services ที่ทำงานด้วย Android , iOS , Windows Phone หรือ Windows Store ซึ่งนั้นหมายความว่า Mobile Services ที่เราสร้างขึ้น จะถูกสร้าง และติดต่อกับ Application ได้หลากหลายทาง รองรับการทำงานได้จากอุปกรณ์หลาย ๆ เภท ซึ่งอยู่บน Base บน Mobile Services ตัวเดียวกัน
Azure Mobile Services HTML/JavaScript
แต่การเขียน Azure Mobile Services ในปัจจุบันยังมีข้อจำกัดและความสามารถบางอย่างที่ไม่สามารถทำได้เหมือนกับ Android, iOS , WP และ Windows Store คือ จะสามารถใช้แค่ Interface ง่าย ๆ เช่น การรับส่งข้อมูลจาก Mobile Services แต่ไม่สามารถทำ Push Notification ไปยัง Clientได้
การเขียน Azure Mobile Services ทำงานร่วมกับ HTML/JavaScript
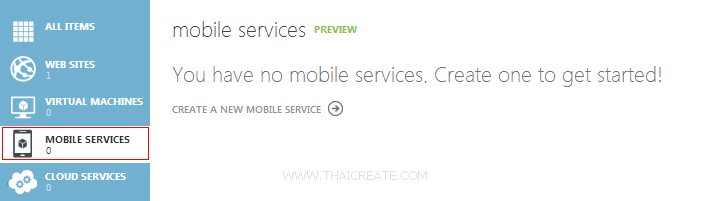
ในหน้าจอบน Portal Management ของ Windows Azure ให้เลือก Service ของ Mobile Services เราสามารถคลิกที่ Create a new Mobile Service ได้ในทันที

หรือจะคลิกที่ New
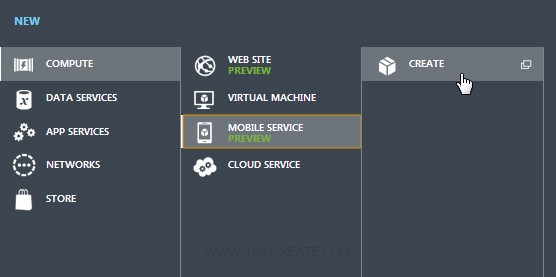
เลือก COMPUTE -> MOBILE SERVICE -> CREATE
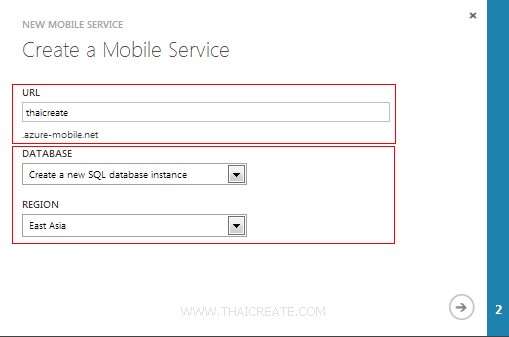
บริการ Mobile Services จะต้องมีการกำหนด URL ซึ่งจะอยู่ภายใต้ Subdomain ของ azure-mobile.net และเลือก Create a new SQL database instance
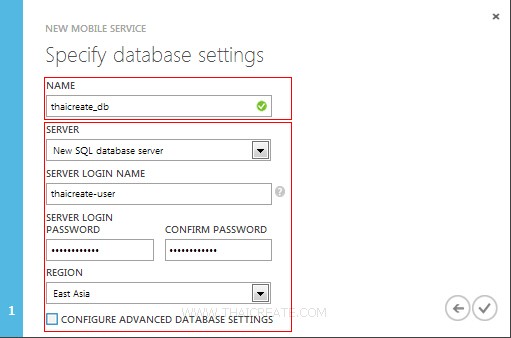
กำหนดชื่อ Database รวมทั้งเลือก New SQL database server พร้อมกับกำหนด Username และ Password ในการ Login

จากนั้นเราจะได้ Mobile Services ขึ้นมา 1 รายการ ให้คลิกเข้าไปในหน้าหลัก
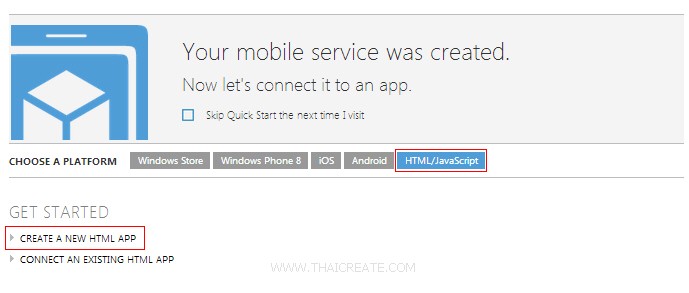
ในหน้าหลักให้เราเลือก Platform เป็นของ HTML/JavaScript และเลือก CREATE A NEW HTML APP
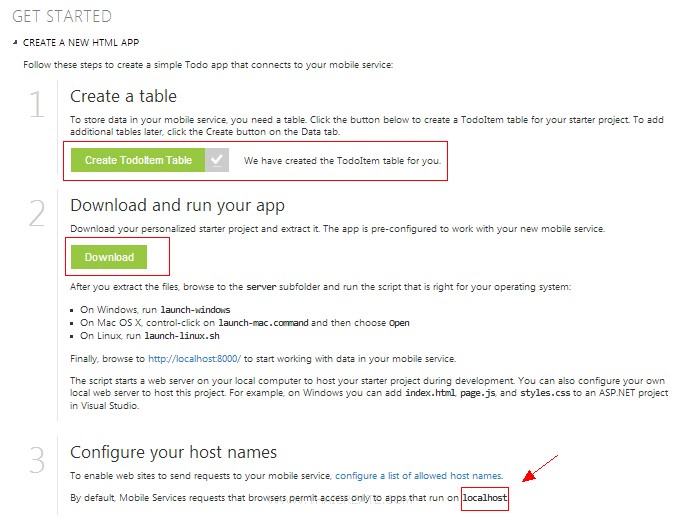
ขั้นตอนนี้จะมีอยู่ 3 Step คือ
- 1. ให้คลิกที่ Create TodoItem Table เพื่อทำการสร้างตารางสำหรับเก็บข้อมูลที่มีชื่อว่า TodoItem
- 2. ให้ดาวน์โหลด Sample Project เพื่อนำไปรันทดสอบบนโปรแกรมผ่าน Web Browser ได้ในทันที
- 3. หรือจะรันผ่าน IIS , Apache ที่เรามีอยู่แล้วก็ได้ โดยจะทำงานผ่าน localhost
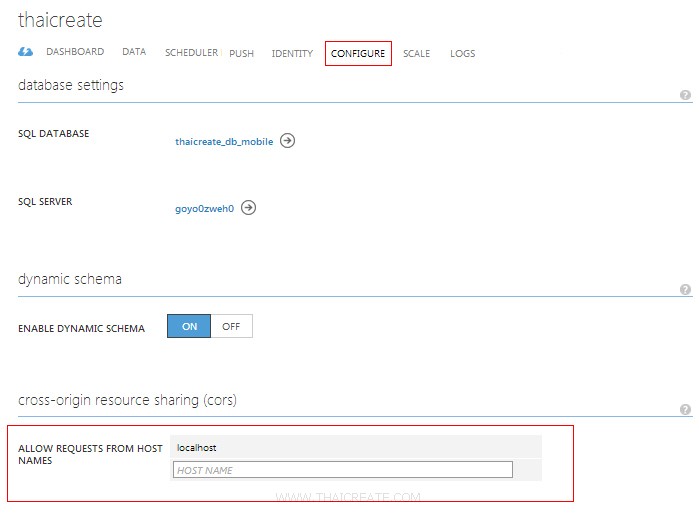
ก่อนก่อนใช้งานให้ตรวจสอบสิทธิ์ของ URL ด้วยว่า URL ที่ทำการ Request นั้นมีสิทธิ์หรือไม่ ซึ่งเป็นระบบรักษาความปลอดภัยที่เราสามารถจะ Allow เฉพาะ Domain ที่เราต้องการได้ ซึ่งในตอนนี้ให้ Allow โดเมน localhost เพราะเราจะทดสอบการ localhost เครื่องของเรา
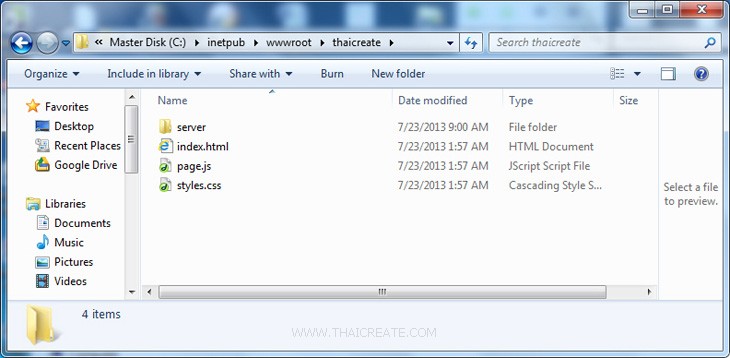
หลังจาก Download เรียบร้อยแล้วให้แตกไฟล์ไปไว้ที่ไหนก็ได้ที่อยู่บนเครื่องของเรา
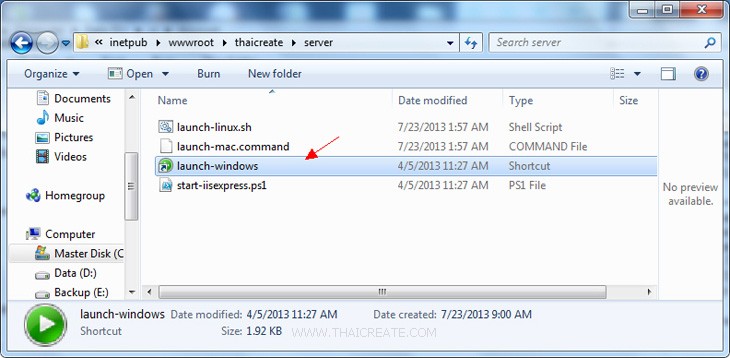
ในไฟล์ที่ Download มาให้จะมี IIS Express มาให้ด้วย นั่นหมายถึงว่า กรณีที่ไม่มี IIS หรือ Apache เราสามารถใช้ตัวนี้ได้ทันที
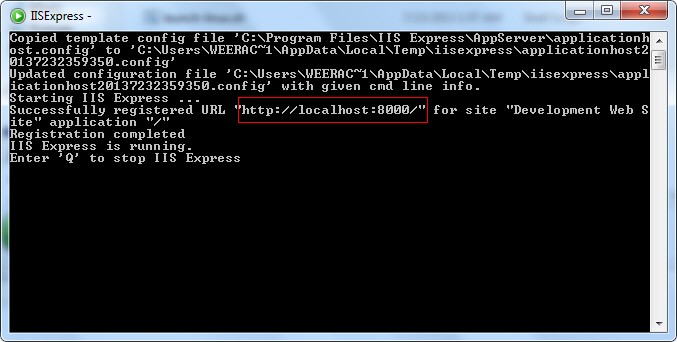
แสดงการทำงานของ IIS Express วึ่งจะทำงานภายใต้ http://localhost:8000/
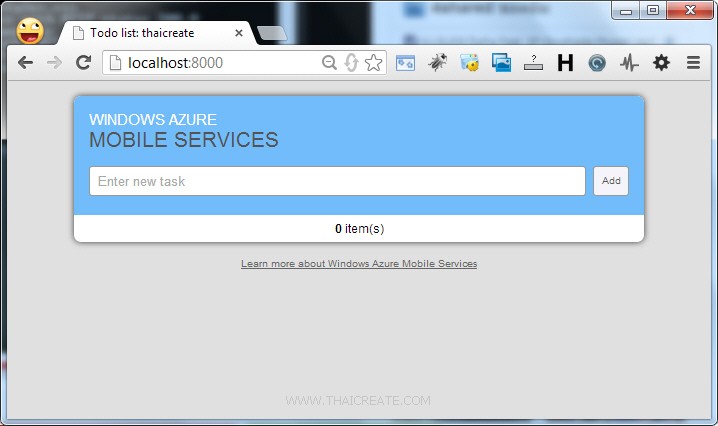
ทดสอบเรียกผ่าน Web Browser ซึ่งเป็นตัวอย่างของ Sample Project
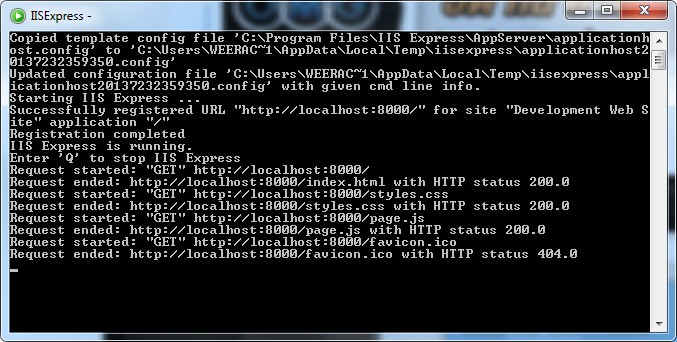
ทุก ๆ ครั้งที่เราเรียก ตัว IIS Express จะมี Log เก็บไว้ด้วย
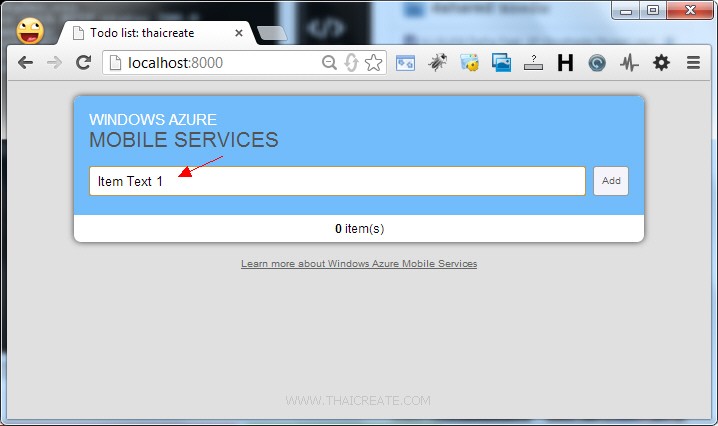
ทดสอบการเพิ่มข้อมูล
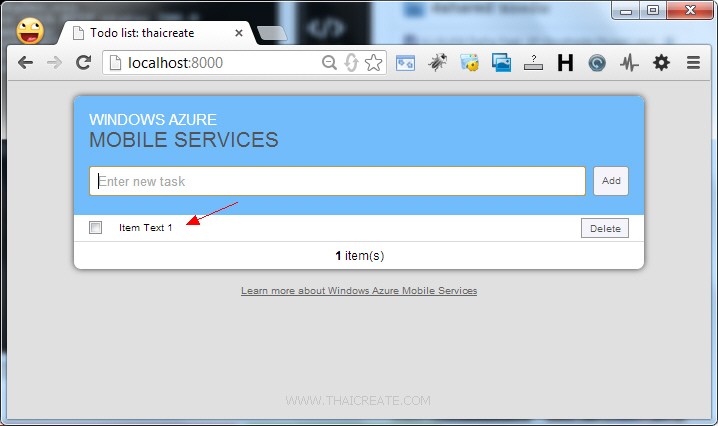
ข้อมูลแสดงใน Item ของ Web
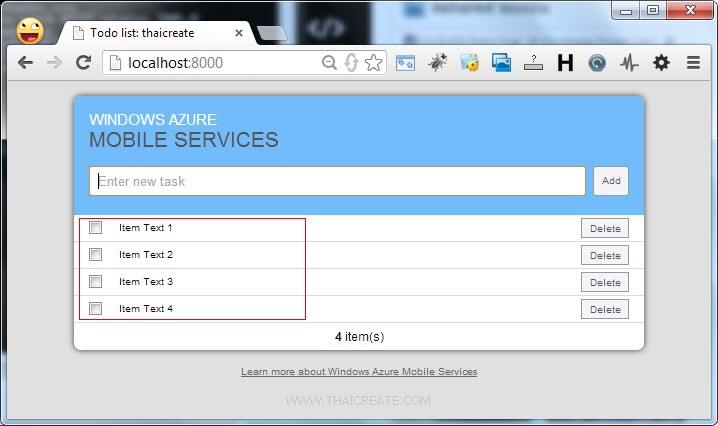
ทดสอบการเพิ่มข้อมูลอื่น ๆ
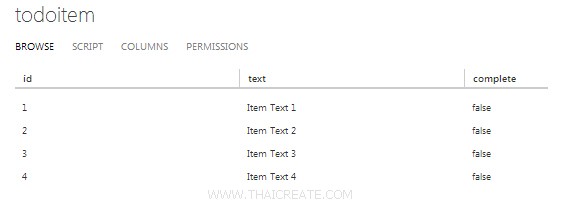
กลับมาที่หน้าจอ Portal Management ของ Mobile Services ให้คลิกที่เมนู DATA เราจะเห็นว่าตอนนี้ตารางที่ชื่อว่า TodoItem จะมีข้อมูลอยู่ 4 รายการ
เพิ่มเติม หรือในกรณีที่เรามี Project อยู่แล้ว ก็เลือกที่จะ Include ด้วยการเรียกใช้งานตามที่ Azure แนะนำ
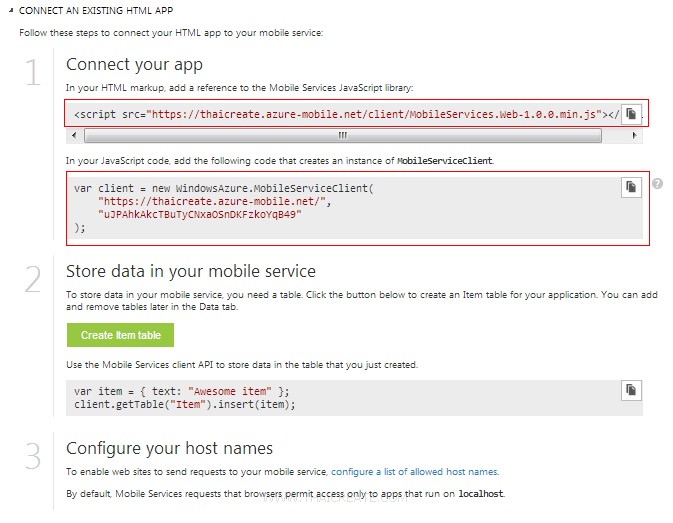
ขั้นตอนการนำ API Library ไปใช้กับ Project ของเรา
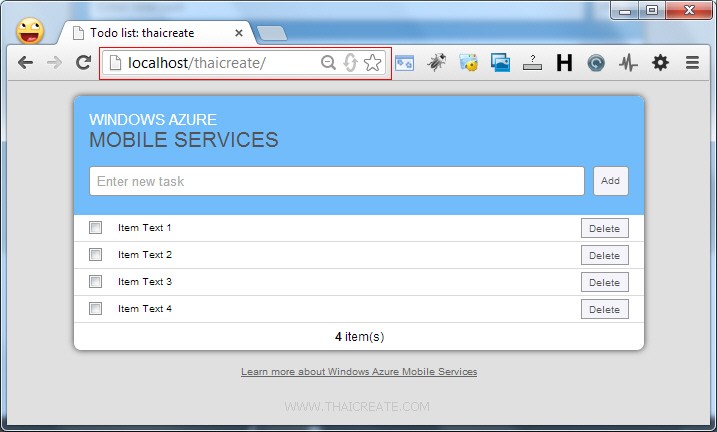
หรือจะ Copy และสร้าง Project ใน IIS / Apache ที่เรามีอยู่แล้วก็ได้เช่นเดียวกัน
โครงสร้างไฟล์ของ API Library ในไฟล์ API เราจะเห็นว่ามีอยู่แค่ 2 ไฟล์ที่สำคัญ ๆ คือ MobileServices.Web-1.0.0.min.js และ page.js

ซึ่งปกติแล้วไฟล์ MobileServices.Web-1.0.0.min.js จะเก็บอยู่ใน Mobile Services ของเราอยู่แล้ว แต่ไฟล์ page.js เป็นไฟล์คำสั่ง JavaScript ต่าง ๆ ที่เราสามารถเขียนเพิ่มได้
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset='utf-8' />
<title>Todo list: thaicreate</title>
<link rel='stylesheet' href='styles.css' />
<meta name='viewport' content='width=device-width' />
<!--[if lt IE 9]><script src="https://cdnjs.cloudflare.com/ajax/libs/html5shiv/3.6.1/html5shiv.js"></script><![endif]-->
</head>
<body>
<div id='wrapper'>
<article>
<header>
<h2>Windows Azure</h2>
<h1>Mobile Services</h1>
<form id='add-item'>
<button type='submit'>Add</button>
<div><input type='text' id='new-item-text' placeholder='Enter new task' /></div>
</form>
</header>
<ul id='todo-items'></ul>
<p id='summary'>Loading...</p>
</article>
<footer>
<a href='http://www.windowsazure.com/en-us/develop/mobile/'>
Learn more about Windows Azure Mobile Services
</a>
<ul id='errorlog'></ul>
</footer>
</div>
<script src='https://ajax.aspnetcdn.com/ajax/jQuery/jquery-1.9.1.min.js'></script>
<script src='https://thaicreate.azure-mobile.net/client/MobileServices.Web-1.0.0.min.js'></script>
<script src='page.js'></script>
</body>
</html>
page.js
$(function() {
var client = new WindowsAzure.MobileServiceClient('https://thaicreate.azure-mobile.net/', 'uJPAhkAkcTBuTyCNxaOSnDKFzkoYqB49'),
todoItemTable = client.getTable('todoitem');
// Read current data and rebuild UI.
// If you plan to generate complex UIs like this, consider using a JavaScript templating library.
function refreshTodoItems() {
var query = todoItemTable.where({ complete: false });
query.read().then(function(todoItems) {
var listItems = $.map(todoItems, function(item) {
return $('<li>')
.attr('data-todoitem-id', item.id)
.append($('<button class="item-delete">Delete</button>'))
.append($('<input type="checkbox" class="item-complete">').prop('checked', item.complete))
.append($('<div>').append($('<input class="item-text">').val(item.text)));
});
$('#todo-items').empty().append(listItems).toggle(listItems.length > 0);
$('#summary').html('<strong>' + todoItems.length + '</strong> item(s)');
}, handleError);
}
function handleError(error) {
var text = error + (error.request ? ' - ' + error.request.status : '');
$('#errorlog').append($('<li>').text(text));
}
function getTodoItemId(formElement) {
return Number($(formElement).closest('li').attr('data-todoitem-id'));
}
// Handle insert
$('#add-item').submit(function(evt) {
var textbox = $('#new-item-text'),
itemText = textbox.val();
if (itemText !== '') {
todoItemTable.insert({ text: itemText, complete: false }).then(refreshTodoItems, handleError);
}
textbox.val('').focus();
evt.preventDefault();
});
// Handle update
$(document.body).on('change', '.item-text', function() {
var newText = $(this).val();
todoItemTable.update({ id: getTodoItemId(this), text: newText }).then(null, handleError);
});
$(document.body).on('change', '.item-complete', function() {
var isComplete = $(this).prop('checked');
todoItemTable.update({ id: getTodoItemId(this), complete: isComplete }).then(refreshTodoItems, handleError);
});
// Handle delete
$(document.body).on('click', '.item-delete', function () {
todoItemTable.del({ id: getTodoItemId(this) }).then(refreshTodoItems, handleError);
});
// On initial load, start by fetching the current data
refreshTodoItems();
});
ตัวอย่างไฟล์ page.js ซึ่งเราสามารถที่จะเขียนในรูปของเราเองก็ได้

ในไฟล์ page.js จะเห็นว่ามี API Key และรายลเอียด Event อื่น ๆ ที่ใช้การ รับ-ส่ง ข้อมูลจาก HTML/Java Script กับ Azure Mobile Services
บทความถัดไปที่แนะนำให้อ่าน
บทความที่เกี่ยวข้อง
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2013-08-26 06:09:59 /
2017-03-24 12:01:05 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|