ตอนที่ 6 : Windows Store App : Login User Password (Azure Mobile Services) |
ตอนที่ 6 : Windows Store App : Login User Password (Azure Mobile Services) หลังจากบทความก่อนหน้านี้เราได้ทำระบบ Register Form บน Windows Store App และส่งข้อมูลไปเก็บไว้ที่ Mobile Services ไปเรียบร้อยแล้ว บทความนี้จะเป็นการทำระบบ Login Form ด้วย Username และ Password โดยข้อมูลและ Table ถูกจัดเก็บไว้ที่ Mobile Services บน Windows Azure
Windows Store App Mobile Services Login Form
สำหรับขั้นตอนนั้นก็คือสร้าง Form Page สำหรับตรวจสอบ Username และ Password และเมื่อ Login ผ่านก็จะทำการ Redirect ไปยังอีก Page เพื่อแสดงข้อมูลของ User นั้น ๆ ที่ได้ทำการ Login เข้ามาในระบบ
Example ตัวอย่างการทำระบบล็อกอิน Member Login Form บน Windows Store App / Mobile Services

ตอนนี้เรามี Mobile Services อยู่ 1 รายการ
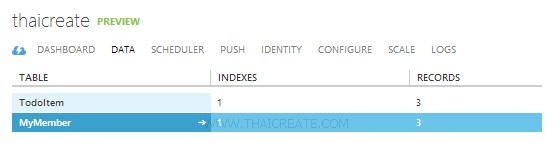
ชื่อตารางว่า MyMember
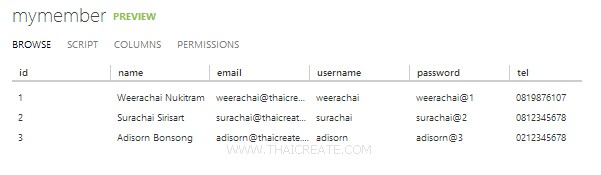
มีข้อมูลอยู่ 3 รายการ
กลับมายัง Project ของ Windows Store App บน Visual Studio
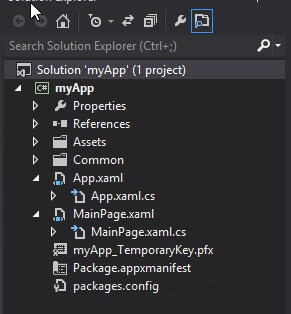
โครงสร้างไฟล์
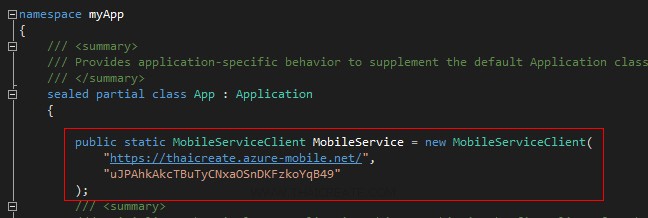
ในไฟล์ App.xaml.cs ให้ Copy Url และ key มาวางดังรูป จากนั้นออกแบบ Layout และเขียน Code ดังนี้
MainPage.xaml
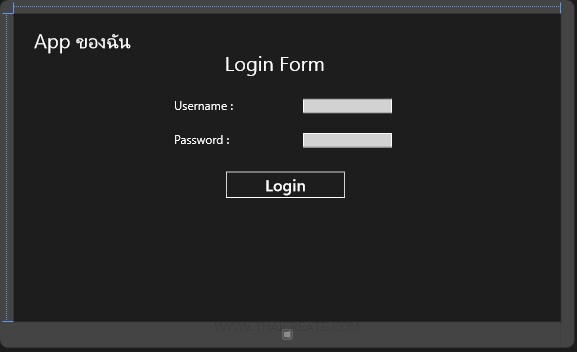
<Page
x:Class="myApp.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:myApp"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<TextBlock HorizontalAlignment="Left" Margin="50,41,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="50" Width="355" Height="80">
<Run Text="App ของฉัน"/>
<LineBreak/>
<Run/>
</TextBlock>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Margin="12,2,12,-2">
<TextBlock HorizontalAlignment="Left" Margin="515,95,0,0" TextWrapping="Wrap" Text="Login Form" VerticalAlignment="Top" FontSize="50"/>
<TextBlock HorizontalAlignment="Left" Margin="388,210,0,0" TextWrapping="Wrap" Text="Username :" VerticalAlignment="Top" FontSize="30"/>
<TextBox x:Name="txtUsername" HorizontalAlignment="Left" Height="36" Margin="711,210,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="222" FontSize="20"/>
<TextBlock HorizontalAlignment="Left" Margin="388,296,0,0" TextWrapping="Wrap" Text="Password :" VerticalAlignment="Top" FontSize="30"/>
<PasswordBox x:Name="txtPassword" HorizontalAlignment="Left" Margin="711,296,0,0" VerticalAlignment="Top" Width="222" RenderTransformOrigin="1.072,1.969" Height="36"/>
<Button x:Name="btnLogin" Content="Login" HorizontalAlignment="Left" Margin="515,389,0,0" VerticalAlignment="Top" Click="btnLogin_Click" RenderTransformOrigin="8.238,3.789" FontSize="40" Width="303"/>
</Grid>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
using Windows.UI.Popups;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace myApp
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public class MyMember
{
public int Id { get; set; }
[JsonProperty(PropertyName = "username")]
public string Username { get; set; }
[JsonProperty(PropertyName = "password")]
public string Password { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "tel")]
public string Tel { get; set; }
[JsonProperty(PropertyName = "email")]
public string Email { get; set; }
}
public sealed partial class MainPage : Page
{
private MobileServiceCollection<MyMember, MyMember> items;
private IMobileServiceTable<MyMember> memberTable = App.MobileService.GetTable<MyMember>();
public MainPage()
{
this.InitializeComponent();
}
private async void btnLogin_Click(object sender, RoutedEventArgs e)
{
try
{
items = await memberTable
.Where(memberItem => memberItem.Username == txtUsername.Text
&& memberItem.Password == txtPassword.Password)
.ToCollectionAsync();
if (items.Count > 0)
{
MyMember member = items[0];
this.Frame.Navigate(typeof(PageInfo), member.Id.ToString());
}
else
{
// Dialog
MessageDialog msgDialog = new MessageDialog("Incorrect Username and Password.", "Status :");
await msgDialog.ShowAsync();
}
}
catch (MobileServiceInvalidOperationException ex)
{
throw;
}
}
/// <summary>
/// Invoked when this page is about to be displayed in a Frame.
/// </summary>
/// <param name="e">Event data that describes how this page was reached. The Parameter
/// property is typically used to configure the page.</param>
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
}
}
เพิ่มไฟล์ชุดที่ 2 สำหรับแสดง User Info
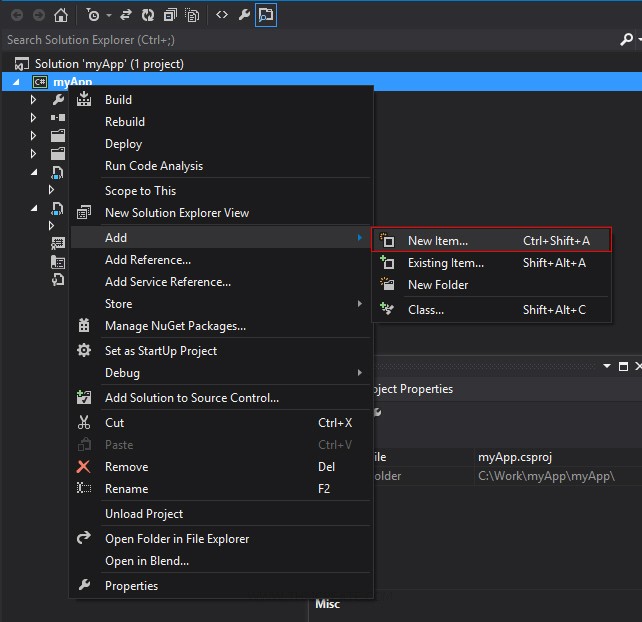
คลิกขวาที่ Solution -> Add -> New Item...
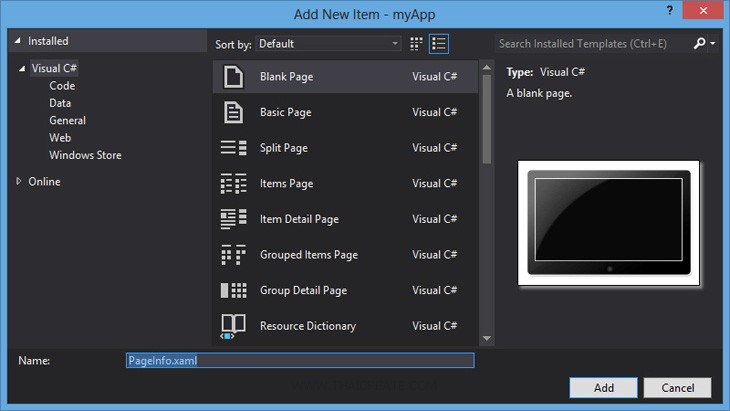
เลือกเป็นแบบ Windows Store แบบ Blank Page แบบแนวตั้ง ตั้งชื่อเป็น PageInfo.xaml
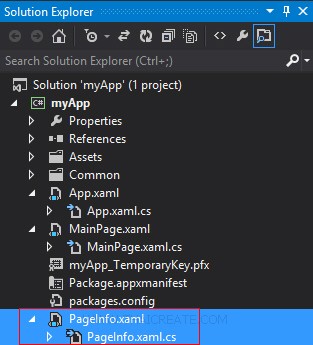
ได้ไฟล์ขึ้นมาอีกชุด PageInfo.xaml และ PageInfo.xaml.cs
PageInfo.xaml
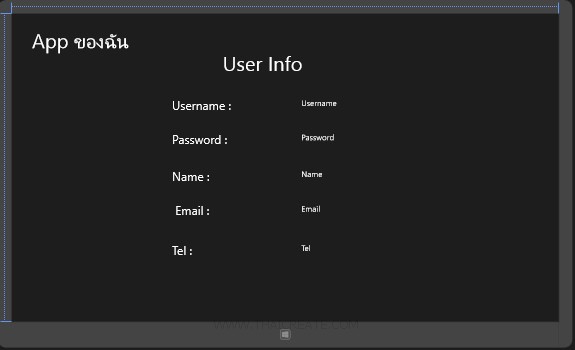
<Page
x:Class="myApp.PageInfo"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:myApp"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<TextBlock HorizontalAlignment="Left" Margin="50,41,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="50" Width="355" Height="80">
<Run Text="App ของฉัน"/>
<LineBreak/>
<Run/>
</TextBlock>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Margin="12,2,12,-2">
<TextBlock HorizontalAlignment="Left" Margin="515,95,0,0" TextWrapping="Wrap" Text="User Info" VerticalAlignment="Top" FontSize="50"/>
<TextBlock HorizontalAlignment="Left" Margin="388,210,0,0" TextWrapping="Wrap" Text="Username :" VerticalAlignment="Top" FontSize="30"/>
<TextBlock x:Name="lblUsername" HorizontalAlignment="Left" Height="36" Margin="711,210,0,0" TextWrapping="Wrap" Text="Username" VerticalAlignment="Top" Width="222" FontSize="20"/>
<TextBlock HorizontalAlignment="Left" Margin="388,296,0,0" TextWrapping="Wrap" Text="Password :" VerticalAlignment="Top" FontSize="30"/>
<TextBlock x:Name="lblPassword" HorizontalAlignment="Left" Margin="711,296,0,0" VerticalAlignment="Top" Text="Password" Width="222" RenderTransformOrigin="1.072,1.969" Height="36" FontSize="20"/>
<TextBlock HorizontalAlignment="Left" Margin="388,388,0,0" TextWrapping="Wrap" Text="Name :" VerticalAlignment="Top" FontSize="30"/>
<TextBlock x:Name="lblName" HorizontalAlignment="Left" Height="36" Margin="711,388,0,0" TextWrapping="Wrap" Text="Name" VerticalAlignment="Top" Width="319" FontSize="20"/>
<TextBlock HorizontalAlignment="Left" Margin="396,474,0,0" TextWrapping="Wrap" Text="Email :" VerticalAlignment="Top" FontSize="30"/>
<TextBlock x:Name="lblEmail" HorizontalAlignment="Left" Height="36" Margin="711,474,0,0" TextWrapping="Wrap" Text="Email" VerticalAlignment="Top" Width="326" FontSize="20"/>
<TextBlock HorizontalAlignment="Left" Margin="388,573,0,0" TextWrapping="Wrap" Text="Tel :" VerticalAlignment="Top" FontSize="30"/>
<TextBlock x:Name="lblTel" HorizontalAlignment="Left" Height="36" Margin="711,573,0,0" TextWrapping="Wrap" Text="Tel" VerticalAlignment="Top" Width="222" FontSize="20"/>
</Grid>
</Grid>
</Page>
PageInfo.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
using Windows.UI.Popups;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace myApp
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class PageInfo : Page
{
private MobileServiceCollection<MyMember, MyMember> items;
private IMobileServiceTable<MyMember> memberTable = App.MobileService.GetTable<MyMember>();
public PageInfo()
{
this.InitializeComponent();
}
/// <summary>
/// Invoked when this page is about to be displayed in a Frame.
/// </summary>
/// <param name="e">Event data that describes how this page was reached. The Parameter
/// property is typically used to configure the page.</param>
protected override void OnNavigatedTo(NavigationEventArgs e)
{
string id = e.Parameter as string;
InfoMember(id);
}
private async void InfoMember(String id)
{
try
{
items = await memberTable
.Where(memberItem => memberItem.Id == Convert.ToInt32(id))
.ToCollectionAsync();
if (items.Count > 0)
{
MyMember member = items[0];
lblUsername.Text = member.Username.ToString();
lblPassword.Text = member.Password.ToString();
lblName.Text = member.Name.ToString();
lblEmail.Text = member.Email.ToString();
lblTel.Text = member.Tel.ToString();
}
else
{
MessageDialog msgDialog = new MessageDialog("Not found Data!", "Error :");
await msgDialog.ShowAsync();
}
}
catch (MobileServiceInvalidOperationException ex)
{
throw ex;
}
}
}
}
Screenshot
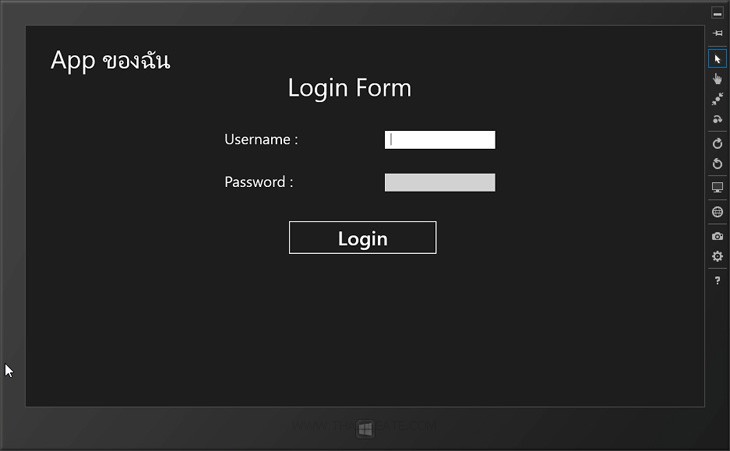
หน้า Login บน Windows Store App ซึ่งจะตรวจสอบ Username และ Password ที่อยู่บน Mobile Services
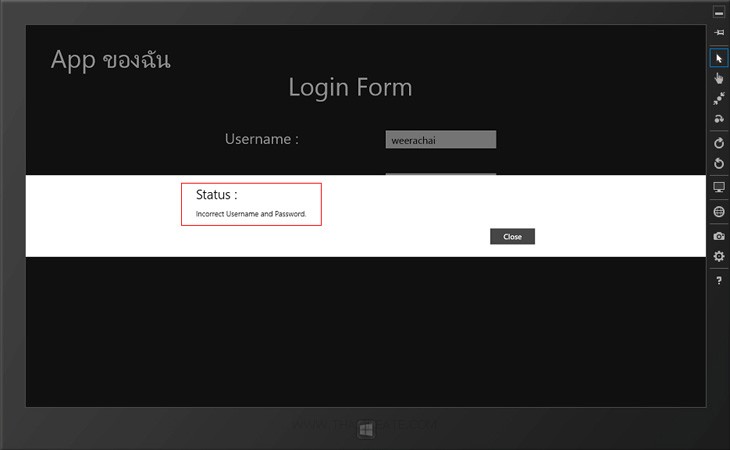
กรณีที่ Login ไม่ถูกต้อง
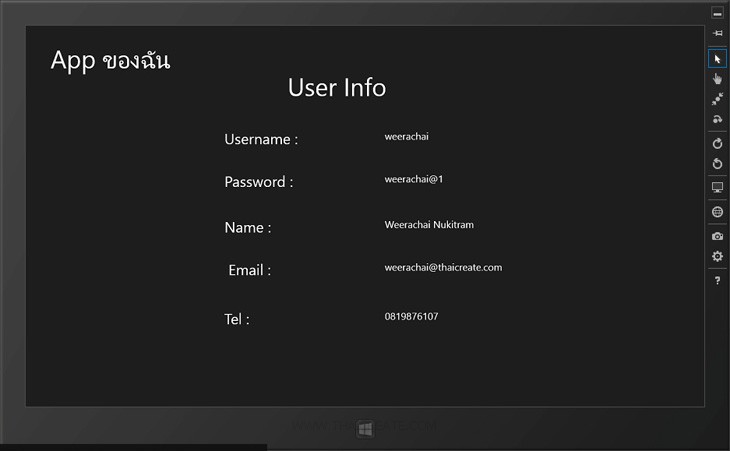
กรณีที่ Login ถูกต้องจะแสดงรายละเอียดของ User นั้น ๆ ที่อ่านข้อมูลจาก Mobile Services ของ Windows Azure
สามารถทำการดาวน์โหลด Code ทั้งหมดได้จากไฟล์แนบของบทความ
|