How to use : Java GUI Choose Data from JDialog to Main Frame |
How to use : Java GUI Choose Data from JDialog to Main Frame ตัวอย่างการเขียน Java GUI และเทคนิคการเขียน GUI การส่งค่าจาก Dialog มายัง Frame หลัก โดยเราจะออกแบบ Frame หลักเป็นตัว Input ข้อมูล แต่จะสามารถเปิด Dialog เพื่อค้นหาข้อมูลต่าง ๆ แล้วเลือกรายการนั้น และหลังจากที่เลือกค่าใน Dialog แล้ว รายการนั้น ๆ จะถูกส่งกลับมายัง Frame หลัก ซึ่งเป็นเทคนิคที่หลาย ๆ Application ใช้ ช่วยให้โปรแกรมใช้งานง่าย และ น่าสนใจยิ่งขึ้น
How to use : Java GUI Choose Data from JDialog to Main Frame
แสดง Dialog ด้วย JDialog เพื่อเลือกข้อมูล
ในตัวอย่างนี้จะเลือกใช้ Database ของ MySQL แต่ในกรณีที่จะใช้ร่วมกับ Database อื่น ๆ ก็สามารถทำได้ง่าย ๆ เพียงแค่เปลี่ยน Connector และ Connection String เท่านั้น และการแสดงข้อมูลจะใช้ JTable ของ Java Swing สามารถอ่านเพิ่มเติมได้ที่บทความนี้
Java Connect to MySQL Database (JDBC)
โครงสร้างของ MySQL และ Table
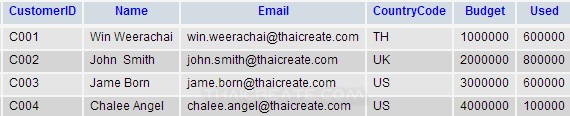
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- dump ตาราง `customer`
--
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
คำสั่งของ SQL ที่สามารถนำไปรันบน Query เพื่อสร้าง Table และ Rows ได้ทันที
Example ตัวอย่างการเขียน Java GUI การสร้าง Dialog Popup เพื่อเลือกข้อมูล
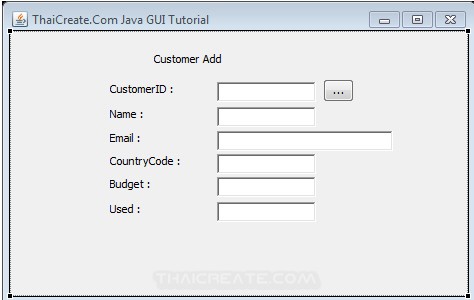
MyForm.java
package com.java.myapp;
import java.awt.EventQueue;
import java.awt.TextField;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class MyForm extends JFrame {
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
MyForm frame = new MyForm();
frame.setVisible(true);
}
});
}
/**
* Create the frame.
*/
public MyForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 474, 303);
setTitle("ThaiCreate.Com Java GUI Tutorial");
getContentPane().setLayout(null);
// Header Customer Add
JLabel hCustomerAdd = new JLabel("Customer Add");
hCustomerAdd.setBounds(144, 21, 132, 14);
getContentPane().add(hCustomerAdd);
// *** Header ***//
JLabel hCustomerID = new JLabel("CustomerID :");
hCustomerID.setBounds(100, 51, 89, 14);
getContentPane().add(hCustomerID);
JLabel hName = new JLabel("Name :");
hName.setBounds(100, 76, 89, 14);
getContentPane().add(hName);
JLabel hEmail = new JLabel("Email :");
hEmail.setBounds(100, 100, 89, 14);
getContentPane().add(hEmail);
JLabel hCountryCode = new JLabel("CountryCode :");
hCountryCode.setBounds(100, 123, 89, 14);
getContentPane().add(hCountryCode);
JLabel hBudget = new JLabel("Budget :");
hBudget.setBounds(100, 146, 89, 14);
getContentPane().add(hBudget);
JLabel hUsed = new JLabel("Used :");
hUsed.setBounds(100, 171, 89, 14);
getContentPane().add(hUsed);
// *** Add Form ***//
// CustomerID
final TextField txtCustomerID = new TextField("");
txtCustomerID.setBounds(207, 51, 99, 20);
getContentPane().add(txtCustomerID);
// Name
final TextField txtName = new TextField("");
txtName.setBounds(207, 76, 99, 20);
getContentPane().add(txtName);
// Email
final TextField txtEmail = new TextField("");
txtEmail.setBounds(207, 100, 176, 20);
getContentPane().add(txtEmail);
// CountryCode
final TextField txtCountryCode = new TextField("");
txtCountryCode.setBounds(207, 123, 99, 20);
getContentPane().add(txtCountryCode);
// Budget
final TextField txtBudget = new TextField("");
txtBudget.setBounds(207, 146, 99, 20);
getContentPane().add(txtBudget);
// Used
final TextField txtUsed = new TextField("");
txtUsed.setBounds(207, 171, 99, 20);
getContentPane().add(txtUsed);
// Choose
JButton btnChoose = new JButton("...");
btnChoose.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent evt) {
MyCustomer customer = new MyCustomer();
customer.setModal(true);
customer.setVisible(true);
txtCustomerID.setText(customer.sCustomerID);
txtName.setText(customer.sName);
txtEmail.setText(customer.sEmail);
txtCountryCode.setText(customer.sCountryCode);
txtBudget.setText(customer.sBudget);
txtUsed.setText(customer.sUsed);
}
});
btnChoose.setBounds(313, 48, 31, 23);
getContentPane().add(btnChoose);
}
}
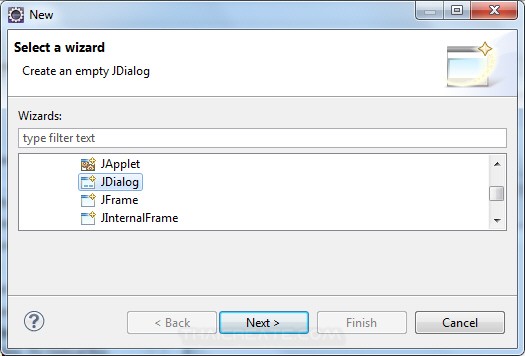
สร้างไฟล์ Class ขึ้นมาใหม่ โดยเลือกเป็น JDialog
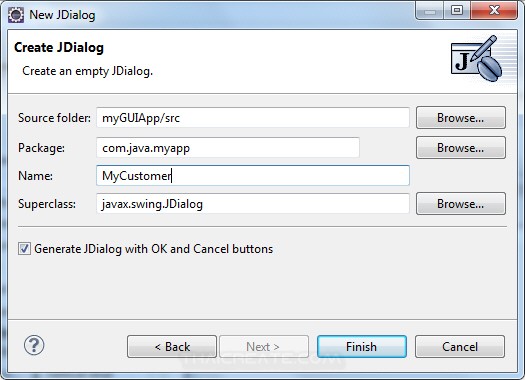
ตั้งชื่อของ Class
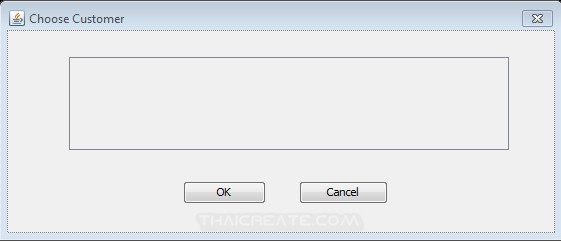
ออกแบบ Dialog ด้วย JTable
MyCustomer.java
package com.java.myapp;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.swing.JButton;
import javax.swing.JDialog;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class MyCustomer extends JDialog {
public String sCustomerID;
public String sName;
public String sEmail;
public String sCountryCode;
public String sBudget;
public String sUsed;
/**
* Launch the application.
*/
public static void main(String[] args) {
try {
MyCustomer dialog = new MyCustomer();
dialog.setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
dialog.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* Create the dialog.
*/
public MyCustomer() {
setBounds(100, 100, 564, 241);
setTitle("Choose Customer");
getContentPane().setLayout(null);
// ScrollPane
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(62, 27, 440, 93);
getContentPane().add(scrollPane);
// Table
final JTable table = new JTable();
scrollPane.setViewportView(table);
// Model for Table
DefaultTableModel model = (DefaultTableModel)table.getModel();
model.addColumn("CustomerID");
model.addColumn("Name");
model.addColumn("Email");
model.addColumn("CountryCode");
model.addColumn("Budget");
model.addColumn("Used");
Connection connect = null;
Statement s = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connect = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase" +
"?user=root&password=root");
s = connect.createStatement();
String sql = "SELECT * FROM customer ORDER BY CustomerID ASC";
ResultSet rec = s.executeQuery(sql);
int row = 0;
while((rec!=null) && (rec.next()))
{
model.addRow(new Object[0]);
model.setValueAt(rec.getString("CustomerID"), row, 0);
model.setValueAt(rec.getString("Name"), row, 1);
model.setValueAt(rec.getString("Email"), row, 2);
model.setValueAt(rec.getString("CountryCode"), row, 3);
model.setValueAt(rec.getFloat("Budget"), row, 4);
model.setValueAt(rec.getFloat("Used"), row, 5);
row++;
}
rec.close();
} catch (Exception e) {
// TODO Auto-generated catch block
JOptionPane.showMessageDialog(null, e.getMessage());
e.printStackTrace();
}
try {
if(s != null) {
s.close();
connect.close();
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Button OK
JButton btnOk = new JButton("OK");
btnOk.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int index = table.getSelectedRow();
sCustomerID = table.getValueAt(index, 0).toString();
sName = table.getValueAt(index, 1).toString();
sEmail = table.getValueAt(index, 2).toString();
sCountryCode = table.getValueAt(index, 3).toString();
sBudget = table.getValueAt(index, 4).toString();
sUsed = table.getValueAt(index, 5).toString();
dispose();
}
});
btnOk.setBounds(176, 151, 83, 23);
getContentPane().add(btnOk);
// Button Cancel
JButton btnCancel = new JButton("Cancel");
btnCancel.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
dispose();
}
});
btnCancel.setBounds(292, 151, 89, 23);
getContentPane().add(btnCancel);
}
}
Output
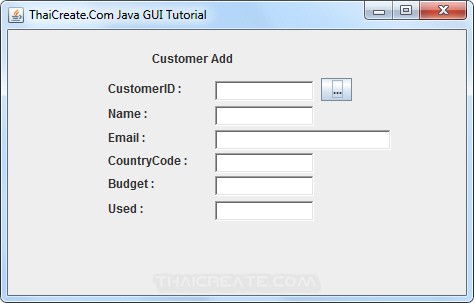
แสดง Frame หลัก ให้คลิกที่ปุ่ม Button เพื่อเปิด Dialog
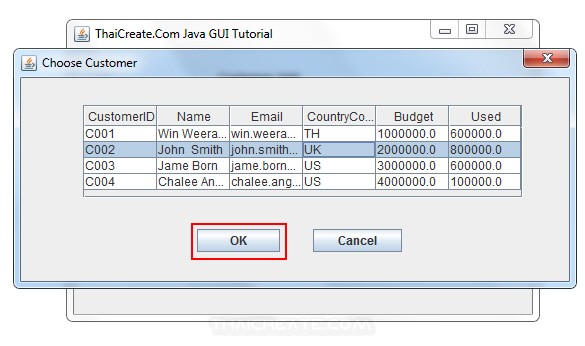
แสดง Dialog และเลือกรายการ
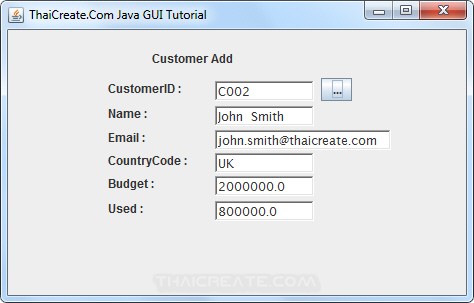
รายการจะถูกส่งกลับมายัง Frame Main
อ่านเพิ่มเติม : พื้นฐาน Java GUI : Dialog และ Popup สร้าง Input Dialog และ Custom Modal Dialog
กรณีที่ใช้ร่วมกับ Database อื่น ๆ สามารถดูวิธีการใช้ Connector และ Connection String ได้ที่นี่
Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2013-09-10 10:06:26 /
2017-03-27 21:55:41 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|