Android Update Data to Server via Web Service |
Android Update Data to Server via Web Service การเขียน Android เพื่อแก้ไข Update ข้อมูลที่อยู่บน Server Database ผ่าน Web Service ที่ทำงานร่วมกับ PHP กับ MySQL หลักการก็คือ ออกแบบ Web Service ไว้ 3 ตัวคือ
- getMember.php ว้สำหรับแสดงรายการข้อมูลทั้งหมดลงใน ListView ของ Android
- resultByMemberID.php ใช้สำหรับแสดงข้อมูลที่ได้เลือกทำการ Update
- updateMemberData.php ใช้สำหรับ Update ข้อมูลที่ถูกแก้ไขและส่งมาจาก Android
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET" />
ในการเขียน Android เพื่อติดต่อกับ Internet จะต้องกำหนด Permission ในส่วนนี้ด้วยทุกครั้ง
Web Service
member
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');

getMember.php
<?
ob_start();
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/getMember.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("resultMember");
// Register our method and argument parameters
$varname = array(
'strName' => "xsd:string"
);
$server->register('resultMember',$varname, array('return' => 'xsd:string'));
function resultMember($strName)
{
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE 1 AND Name LIKE '%".$strName."%' ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
header('Content-type: application/json');
return json_encode($resultArray);
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
resultByMemberID.php
<?
ob_start();
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/resultByMemberID.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("resultMember");
// Register our method and argument parameters
$varname = array(
'strMemberID' => "xsd:string"
);
$server->register('resultMember',$varname, array('return' => 'xsd:string'));
function resultMember($strMemberID)
{
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM member WHERE 1
AND MemberID = '".$strMemberID."'
";
$objQuery = mysql_query($strSQL);
$obResult = mysql_fetch_array($objQuery);
if($obResult)
{
$arr["MemberID"] = $obResult["MemberID"];
$arr["Username"] = $obResult["Username"];
$arr["Password"] = $obResult["Password"];
$arr["Name"] = $obResult["Name"];
$arr["Email"] = $obResult["Email"];
$arr["Tel"] = $obResult["Tel"];
}
mysql_close($objConnect);
header('Content-type: application/json');
return json_encode($arr);
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
updateMemberData.php
<?
ob_start();
require_once("lib/nusoap.php");
//Create a new soap server
$server = new soap_server();
//Define our namespace
$namespace = "https://www.thaicreate.com/android/updateMemberData.php";
$server->wsdl->schemaTargetNamespace = $namespace;
//Configure our WSDL
$server->configureWSDL("updateMember");
// Register our method and argument parameters
$varname = array(
'strMemberID' => "xsd:string",
'strPassword' => "xsd:string",
'strName' => "xsd:string",
'strEmail' => "xsd:string",
'strTel' => "xsd:string"
);
$server->register('updateMember',$varname, array('return' => 'xsd:string'));
function updateMember($strMemberID,$strPassword,$strName,$strEmail,$strTel)
{
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
/*** Check Email Exists ***/
$strSQL = "SELECT * FROM member WHERE Email = '".$strEmail."' AND MemberID != '".$strMemberID."' ";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
if($objResult)
{
$arr['StatusID'] = "0";
$arr['Error'] = "Email Exists!";
header('Content-type: application/json');
mysql_close($objConnect);
return json_encode($arr);
}
/*** Update ***/
$strSQL = " UPDATE member SET
Password = '".$strPassword."'
,Name = '".$strName."'
,Email = '".$strEmail."'
,Tel = '".$strTel."'
WHERE MemberID = '".$strMemberID."'
";
$objQuery = mysql_query($strSQL);
if(!$objQuery)
{
$arr['StatusID'] = "0";
$arr['Error'] = "Cannot save data!";
}
else
{
$arr['StatusID'] = "1";
$arr['Error'] = "";
}
/**
$arr['StatusID'] // (0=Failed , 1=Complete)
$arr['Error'] // Error Message
*/
header('Content-type: application/json');
mysql_close($objConnect);
return json_encode($arr);
}
// Get our posted data if the service is being consumed
// otherwise leave this data blank.
$POST_DATA = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : '';
// pass our posted data (or nothing) to the soap service
$server->service($POST_DATA);
exit();
?>
Android Proejct
โครงสร้างของไฟล์ประกอบด้วย 5 ไฟล์คือ MainActivity.java, activity_main.xml, activity_column.xml และ UpdateActivity.java, activity_update.xml
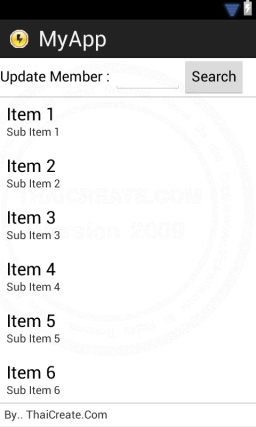
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Update Member : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtKeySearch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="4" >
</EditText>
<Button
android:id="@+id/btnSearch"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Search" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColMemberID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.2"
android:text="MemberID"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1.0"
android:text="Name"
android:textAppearance="?android:attr/textAppearanceSmall" />
<TextView
android:id="@+id/ColTel"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Tel"
android:textAppearance="?android:attr/textAppearanceSmall" />
</LinearLayout>
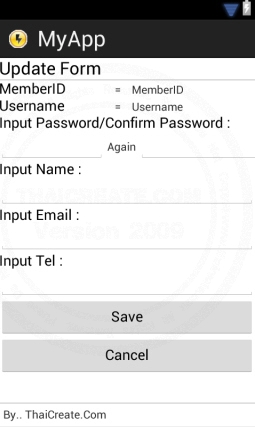
activity_update.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Update Form "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TableRow>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="MemberID "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:layout_weight="0.2"
android:text=" = " />
<TextView
android:id="@+id/txtMemberID"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="MemberID" />
</TableRow>
<TableRow>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:layout_weight="0.2"
android:text=" = " />
<TextView
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username" />
</TableRow>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Password/Confirm Password :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<TableRow>
<EditText
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="6"
android:inputType="textPassword" >
</EditText>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=" Again " />
<EditText
android:id="@+id/txtConPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="7"
android:inputType="textPassword" >
</EditText>
</TableRow>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Name :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" >
</EditText>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Email :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:ems="10" >
</EditText>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Tel :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtTel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="phone"
android:ems="10" >
</EditText>
<Button
android:id="@+id/btnSave"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Save" />
<Button
android:id="@+id/btnCancel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Cancel" />
</TableLayout >
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
MainActivity.java
package com.myapp;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import org.xmlpull.v1.XmlPullParserException;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.view.ContextMenu;
import android.view.ContextMenu.ContextMenuInfo;
import android.view.LayoutInflater;
import android.view.MenuItem;
import android.view.View;
import android.view.Menu;
import android.view.ViewGroup;
import android.view.inputmethod.InputMethodManager;
import android.widget.AdapterView;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
// for List Data
private final String NAMESPACE = "https://www.thaicreate.com/android/getMember.php";
private final String URL = "https://www.thaicreate.com/android/getMember.php?wsdl"; // WSDL URL
private final String SOAP_ACTION = "https://www.thaicreate.com/android/getMember.php/resultMember";
private final String METHOD_NAME = "resultMember"; // Method on web service
ArrayList<HashMap<String, String>> MyArrList;
String[] Cmd = {"View","Update","Delete"};
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
ShowData();
// btnSearch
final Button btnSearch = (Button) findViewById(R.id.btnSearch);
//btnSearch.setBackgroundColor(Color.TRANSPARENT);
// Perform action on click
btnSearch.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
ShowData();
}
});
}
public void ShowData()
{
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
// keySearch
EditText strKeySearch = (EditText)findViewById(R.id.txtKeySearch);
// Disbled Keyboard auto focus
InputMethodManager imm = (InputMethodManager)getSystemService(
Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(strKeySearch.getWindowToken(), 0);
/** Web Service return JSON Code
* [{"MemberID":"1","Username":"weerachai","Password":"weerachai@1","Name":"Weerachai Nukitram","Tel":"0819876107","Email":"[email protected]"},
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"},
* {"MemberID":"3","Username":"surachai","Password":"surachai@3","Name":"Surachai Sirisart","Tel":"0876543210","Email":"[email protected]"}]
*/
SoapObject request = new SoapObject(NAMESPACE, METHOD_NAME);
request.addProperty("strName", strKeySearch.getText().toString());
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL);
try {
androidHttpTransport.call(SOAP_ACTION, envelope);
SoapObject result = (SoapObject) envelope.bodyIn;
if (result != null) {
/**
* [{"MemberID":"1","Username":"weerachai","Password":"weerachai@1","Name":"Weerachai Nukitram","Tel":"0819876107","Email":"[email protected]"},
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"},
* {"MemberID":"3","Username":"surachai","Password":"surachai@3","Name":"Surachai Sirisart","Tel":"0876543210","Email":"[email protected]"}]
*/
MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
JSONArray data = new JSONArray(result.getProperty(0).toString());
for(int i = 0; i < data.length(); i++){
JSONObject c = data.getJSONObject(i);
map = new HashMap<String, String>();
map.put("MemberID", c.getString("MemberID"));
map.put("Username", c.getString("Username"));
map.put("Password", c.getString("Password"));
map.put("Name", c.getString("Name"));
map.put("Email", c.getString("Email"));
map.put("Tel", c.getString("Tel"));
MyArrList.add(map);
}
lisView1.setAdapter(new ImageAdapter(this));
registerForContextMenu(lisView1);
} else {
Toast.makeText(getApplicationContext(),
"Web Service not Response!", Toast.LENGTH_LONG)
.show();
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public void onCreateContextMenu(ContextMenu menu, View v, ContextMenuInfo menuInfo) {
menu.setHeaderIcon(android.R.drawable.btn_star_big_on);
menu.setHeaderTitle("Command");
String[] menuItems = Cmd;
for (int i = 0; i<menuItems.length; i++) {
menu.add(Menu.NONE, i, i, menuItems[i]);
}
}
@Override
public boolean onContextItemSelected(MenuItem item) {
AdapterView.AdapterContextMenuInfo info = (AdapterView.AdapterContextMenuInfo)item.getMenuInfo();
int menuItemIndex = item.getItemId();
String[] menuItems = Cmd;
String CmdName = menuItems[menuItemIndex];
// Check Event Command
if ("View".equals(CmdName)) {
Toast.makeText(MainActivity.this,"Your Selected View",Toast.LENGTH_LONG).show();
/**
* Call the mthod
*/
} else if ("Update".equals(CmdName)) {
Toast.makeText(MainActivity.this,"Your Selected Update",Toast.LENGTH_LONG).show();
String sMemberID = MyArrList.get(info.position).get("MemberID").toString();
String sName = MyArrList.get(info.position).get("Name").toString();
String sTel = MyArrList.get(info.position).get("Tel").toString();
Intent newActivity = new Intent(MainActivity.this,UpdateActivity.class);
newActivity.putExtra("MemberID", sMemberID);
startActivity(newActivity);
} else if ("Delete".equals(CmdName)) {
Toast.makeText(MainActivity.this,"Your Selected Delete",Toast.LENGTH_LONG).show();
/**
* Call the mthod
*/
}
return true;
}
public class ImageAdapter extends BaseAdapter
{
private Context context;
public ImageAdapter(Context c)
{
// TODO Auto-generated method stub
context = c;
}
public int getCount() {
// TODO Auto-generated method stub
return MyArrList.size();
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(final int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColMemberID
TextView txtMemberID = (TextView) convertView.findViewById(R.id.ColMemberID);
txtMemberID.setPadding(10, 0, 0, 0);
txtMemberID.setText(MyArrList.get(position).get("MemberID") +".");
// R.id.ColName
TextView txtName = (TextView) convertView.findViewById(R.id.ColName);
txtName.setPadding(5, 0, 0, 0);
txtName.setText(MyArrList.get(position).get("Name"));
// R.id.ColTel
TextView txtTel = (TextView) convertView.findViewById(R.id.ColTel);
txtTel.setPadding(5, 0, 0, 0);
txtTel.setText(MyArrList.get(position).get("Tel"));
return convertView;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
UpdateActivity.java
package com.myapp;
import java.io.IOException;
import org.json.JSONException;
import org.json.JSONObject;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import org.xmlpull.v1.XmlPullParserException;
import android.os.Bundle;
import android.os.StrictMode;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Intent;
import android.view.View;
import android.view.Menu;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class UpdateActivity extends Activity {
// for Show Data
private final String NAMESPACE1 = "https://www.thaicreate.com/android/resultByMemberID.php";
private final String URL1 = "https://www.thaicreate.com/android/resultByMemberID.php?wsdl"; // WSDL URL
private final String SOAP_ACTION1 = "https://www.thaicreate.com/android/resultByMemberID.php/resultMember";
private final String METHOD_NAME1 = "resultMember"; // Method on web service
// for Update Data
private final String NAMESPACE2 = "https://www.thaicreate.com/android/updateMemberData.php";
private final String URL2 = "https://www.thaicreate.com/android/updateMemberData.php?wsdl"; // WSDL URL
private final String SOAP_ACTION2 = "https://www.thaicreate.com/android/updateMemberData.php/updateMember";
private final String METHOD_NAME2 = "updateMember"; // Method on web service
@SuppressLint("NewApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_update);
// Permission StrictMode
if (android.os.Build.VERSION.SDK_INT > 9) {
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
}
showInfo();
// btnSave
Button btnSave = (Button) findViewById(R.id.btnSave);
// Perform action on click
btnSave.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
if(SaveData())
{
// When Save Complete
Intent newActivity = new Intent(UpdateActivity.this,MainActivity.class);
startActivity(newActivity);
}
}
});
// btnCancel
final Button btnCancel = (Button) findViewById(R.id.btnCancel);
// Perform action on click
btnCancel.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent newActivity = new Intent(UpdateActivity.this,MainActivity.class);
startActivity(newActivity);
}
});
}
public void showInfo()
{
// txtMemberID,txtUsername,txtPassword,txtConPassword,txtName,txtEmail,txtTel
final TextView tMemberID = (TextView)findViewById(R.id.txtMemberID);
final TextView tUsername = (TextView)findViewById(R.id.txtUsername);
final TextView tPassword = (TextView)findViewById(R.id.txtPassword);
final TextView tConPassword = (TextView)findViewById(R.id.txtConPassword);
final TextView tName = (TextView)findViewById(R.id.txtName);
final TextView tEmail = (TextView)findViewById(R.id.txtEmail);
final TextView tTel = (TextView)findViewById(R.id.txtTel);
Button btnSave = (Button) findViewById(R.id.btnSave);
Button btnCancel = (Button) findViewById(R.id.btnCancel);
Intent intent= getIntent();
final String MemberID = intent.getStringExtra("MemberID");
SoapObject request = new SoapObject(NAMESPACE1, METHOD_NAME1);
request.addProperty("strMemberID", MemberID);
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL1);
String resultServer = null;
/** Get result from Server (Return the JSON Code)
*
* {"MemberID":"2","Username":"adisorn","Password":"adisorn@2","Name":"Adisorn Bunsong","Tel":"021978032","Email":"[email protected]"}
*/
try {
androidHttpTransport.call(SOAP_ACTION1, envelope);
SoapObject result = (SoapObject) envelope.bodyIn;
resultServer = result.getProperty(0).toString();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
String strMemberID = "";
String strUsername = "";
String strPassword = "";
String strName = "";
String strEmail = "";
String strTel = "";
JSONObject c;
try {
c = new JSONObject(resultServer);
strMemberID = c.getString("MemberID");
strUsername = c.getString("Username");
strPassword = c.getString("Password");
strName = c.getString("Name");
strEmail = c.getString("Email");
strTel = c.getString("Tel");
if(!strMemberID.equals(""))
{
tMemberID.setText(strMemberID);
tUsername.setText(strUsername);
tPassword.setText(strPassword);
tConPassword.setText(strPassword);
tName.setText(strName);
tEmail.setText(strEmail);
tTel.setText(strTel);
}
else
{
tMemberID.setText("-");
tUsername.setText("-");
tName.setText("-");
tEmail.setText("-");
tTel.setText("-");
btnSave.setEnabled(false);
btnCancel.requestFocus();
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public boolean SaveData()
{
// txtMemberID,txtPassword,txtName,txtEmail,txtTel
final TextView txtMemberID = (TextView)findViewById(R.id.txtMemberID);
final EditText txtPassword = (EditText)findViewById(R.id.txtPassword);
final EditText txtConPassword = (EditText)findViewById(R.id.txtConPassword);
final EditText txtName = (EditText)findViewById(R.id.txtName);
final EditText txtEmail = (EditText)findViewById(R.id.txtEmail);
final EditText txtTel = (EditText)findViewById(R.id.txtTel);
// Dialog
final AlertDialog.Builder ad = new AlertDialog.Builder(this);
ad.setTitle("Error! ");
ad.setIcon(android.R.drawable.btn_star_big_on);
ad.setPositiveButton("Close", null);
// Check Password
if(txtPassword.getText().length() == 0 || txtConPassword.getText().length() == 0 )
{
ad.setMessage("Please input [Password/Confirm Password] ");
ad.show();
txtPassword.requestFocus();
return false;
}
// Check Password and Confirm Password (Match)
if(!txtPassword.getText().toString().equals(txtConPassword.getText().toString()))
{
ad.setMessage("Password and Confirm Password Not Match! ");
ad.show();
txtConPassword.requestFocus();
return false;
}
// Check Name
if(txtName.getText().length() == 0)
{
ad.setMessage("Please input [Name] ");
ad.show();
txtName.requestFocus();
return false;
}
// Check Email
if(txtEmail.getText().length() == 0)
{
ad.setMessage("Please input [Email] ");
ad.show();
txtEmail.requestFocus();
return false;
}
// Check Tel
if(txtTel.getText().length() == 0)
{
ad.setMessage("Please input [Tel] ");
ad.show();
txtTel.requestFocus();
return false;
}
SoapObject request = new SoapObject(NAMESPACE2, METHOD_NAME2);
request.addProperty("strMemberID", txtMemberID.getText().toString());
request.addProperty("strPassword", txtPassword.getText().toString());
request.addProperty("strName", txtName.getText().toString());
request.addProperty("strEmail", txtEmail.getText().toString());
request.addProperty("strTel", txtTel.getText().toString());
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.setOutputSoapObject(request);
HttpTransportSE androidHttpTransport = new HttpTransportSE(URL2);
String resultServer = null;
try {
androidHttpTransport.call(SOAP_ACTION2, envelope);
SoapObject result = (SoapObject) envelope.bodyIn;
resultServer = result.getProperty(0).toString();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (XmlPullParserException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
/** Get result from Server (Return the JSON Code)
* StatusID = ? [0=Failed,1=Complete]
* Error = ? [On case error return custom error message]
*
* Eg Save Failed = {"StatusID":"0","Error":"Email Exists!"}
* Eg Save Complete = {"StatusID":"1","Error":""}
*/
/*** Default Value ***/
String strStatusID = "0";
String strError = "Unknow Status!";
JSONObject c;
try {
c = new JSONObject(resultServer);
strStatusID = c.getString("StatusID");
strError = c.getString("Error");
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// Prepare Save Data
if(strStatusID.equals("0"))
{
ad.setMessage(strError);
ad.show();
return false;
}
else
{
Toast.makeText(UpdateActivity.this, "Update Data Successfully", Toast.LENGTH_SHORT).show();
}
return true;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
AndroidManifest.xml
<activity
android:name="UpdateActivity"
android:theme="@style/AppTheme"
android:screenOrientation="portrait"
android:label="@string/title_activity_main" />
Screenshot
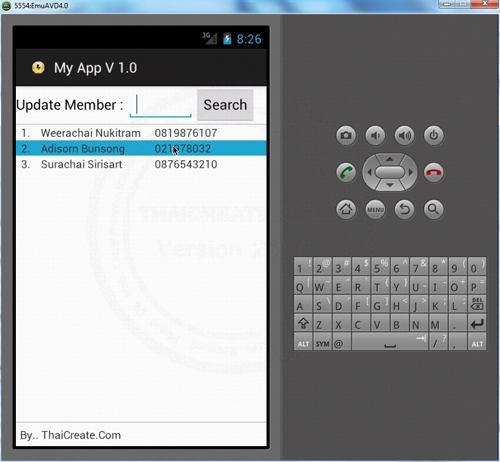
แสดงรายการข้อมูลจาก Web Service
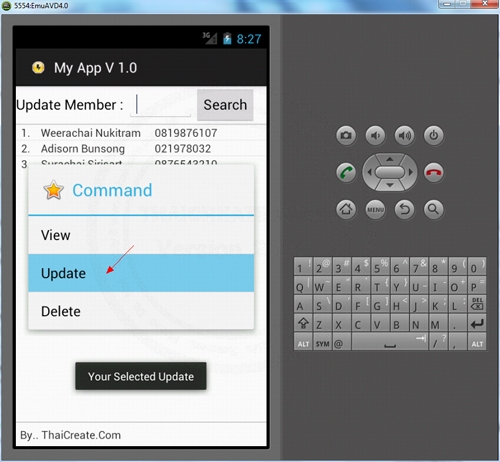
เลือกรายการที่จะแก้ไข โดยใช้การ LongClick และมี Context Menu ให้เลือก Update
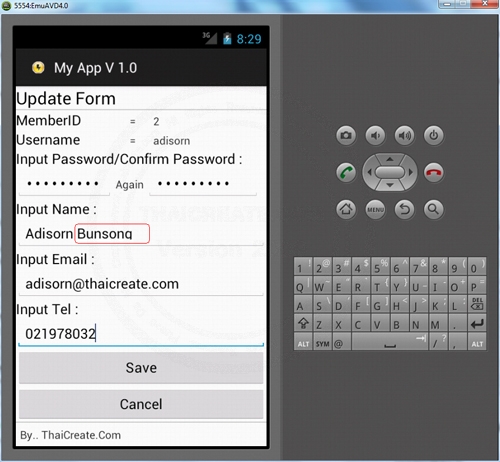
Activity Form สำหรับแก้ไขข้อมูล จะลองแก้ไข นามสกุลในช่อง Name
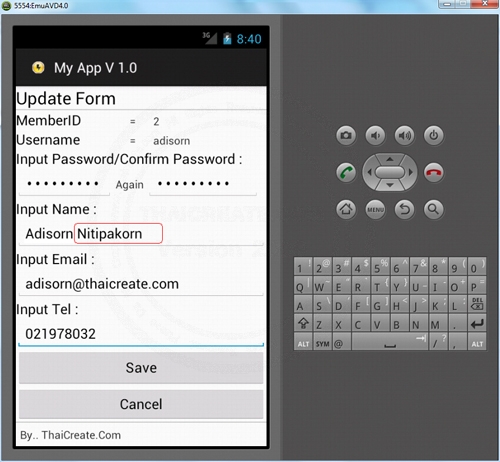
ทดสอบการแก้ไข
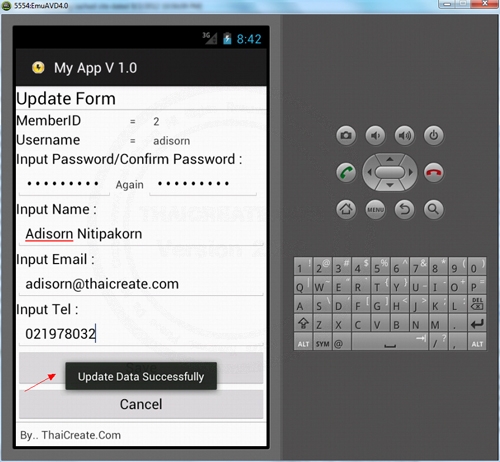
เลือกบันทึกข้อมูล

หลังจากที่บันทึกข้อมุลเรียบร้อยแล้ว และกลับไปดูข้อมูลของ MySQL บน Web Service ก็จะเห็นว่าข้อมูลได้ถูกแก้ไขเรียบร้อยแล้ว
Property & Method (Others Related) |
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2012-08-11 16:06:48 /
2012-08-13 15:51:20 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|