iOS/iPhone Login Username and Password from Web Server (PHP & MySQL) |
iOS/iPhone Login Username and Password from Web Server (PHP & MySQL) บทความนี้จะเป็นการ Apply การใช้งานระหว่าง Client กับ Web Server (PHP กับ MySQL Database) ด้วยการทำระบบ Login ตรวจสอบ Username และ Password ที่ถูกจัดเก็บไว้บน Web Server โดยใช้ Class ของ NSURLConnection ในการที่จะส่งค่า POST ที่เป็น Username และ Password ไปตรวจสอบในฝั่งของ Web Server ซึ่งจะใช้ PHP ทำหน้าที่นำข้อมูลไปตรวจสอบในฐานข้อมูล MySQL Database พร้อมๆ กับการแจ้งสถานะกลับมายัง Client เพื่อให้ทราบผลการ Login และในตัวอย่างนี้ยังทำการแสดงข้อมูลผู้ที่ Login หลังจากทีได้ Login ผ่านเรียบร้อยแล้ว
iOS/iPhone Login Username and Password from Web Server (PHP & MySQL)
จากบทความก่อนหน้านี้เราได้เขียน iOS สำหรับการ Register Form ด้วยการส่งข้อมูลจากในหน้าจอ View ไปจัดเก็บบนฐานข้อมูลของ MySQL ที่อยู่บน Web Server โดยสามารถอ่านตัวอย่างการ Register Data ได้จากบทความนี้
iOS/iPhone Register Form and Send Data to Web Server (PHP & MySQL)
Example การทำ Login Form ตรวจสอบ Username และ Password และการแสดงข้อมูลผู้ Login
MySQL Database
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');
โครงสร้างของ MySQL Database

checkLogin.php ไฟล์ php สำหรับตรวจสอบการ Login ด้วย Username และ Password
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
//$_POST["sUsername"] = "weerachai"; // for Sample
//$_POST["sPassword"] = "weerachai@1"; // for Sample
$strUsername = $_POST["sUsername"];
$strPassword = $_POST["sPassword"];
$strSQL = "SELECT * FROM member WHERE 1
AND Username = '".$strUsername."'
AND Password = '".$strPassword."'
";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
$intNumRows = mysql_num_rows($objQuery);
if($intNumRows==0)
{
$arr["Status"] = "0";
$arr["MemberID"] = "0";
$arr["Message"] = "Incorrect Username and Password";
echo json_encode($arr);
exit();
}
else
{
$arr["Status"] = "1";
$arr["MemberID"] = $objResult["MemberID"];
$arr["Message"] = "Login Successfully";
echo json_encode($arr);
exit();
}
/**
return
// (0=Failed , 1=Complete)
// MemberID
// Error Message
*/
mysql_close($objConnect);
?>

getUserByMemberID.php ไฟล์ php สำหรับการ แสดงข้อมูลผู้ที่กำลัง Login อยู่ในขณะนั้น
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
//$_POST["sMemberID"] = "1"; // for Sample
$strMemberID = $_POST["sMemberID"];
$strSQL = "SELECT * FROM member WHERE 1 AND MemberID = '".$strMemberID."' ";
$objQuery = mysql_query($strSQL);
$obResult = mysql_fetch_array($objQuery);
if($obResult)
{
$arr["MemberID"] = $obResult["MemberID"];
$arr["Username"] = $obResult["Username"];
$arr["Password"] = $obResult["Password"];
$arr["Name"] = $obResult["Name"];
$arr["Email"] = $obResult["Email"];
$arr["Tel"] = $obResult["Tel"];
}
mysql_close($objConnect);
/*** return JSON by MemberID ***/
/* Eg :
{"MemberID":"2",
"Username":"adisorn",
"Password":"adisorn@2",
"Name":"Adisorn Bunsong",
"Tel":"021978032",
"Email":"[email protected]"}
*/
echo json_encode($arr);
?>
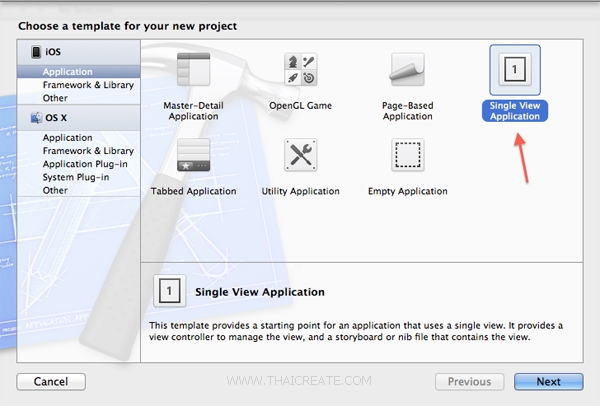
เริ่มต้นด้วยการสร้าง Application บน Xcode แบบ Single View Application
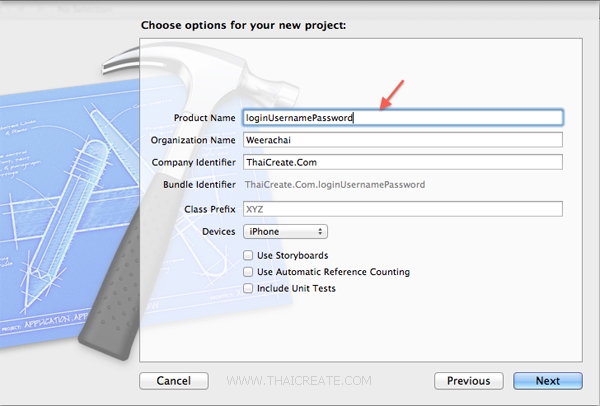
เลือกและไม่เลือกรายการดังรูป
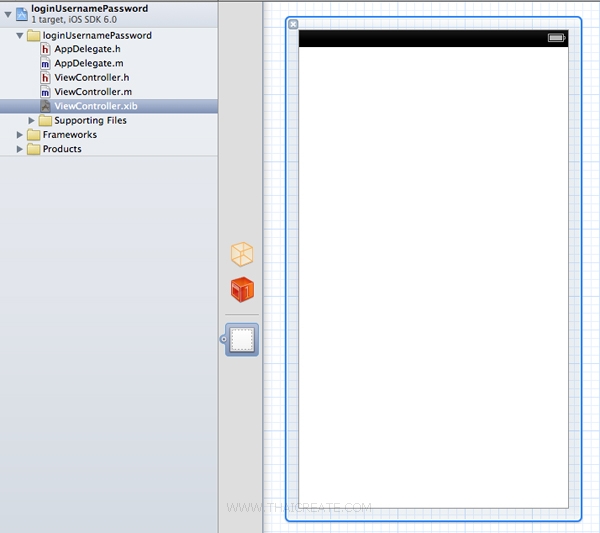
ตอนนี้หน้าจอ View ของเรายังว่าง ๆ
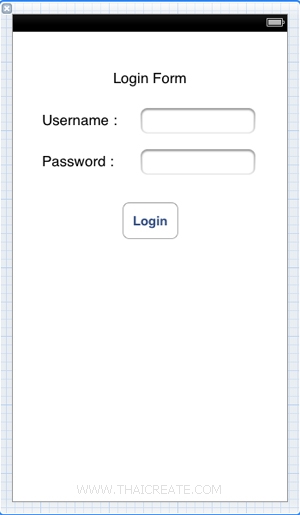
ออกแบบ Form สำหรับ Login ด้วยหน้าจอดังรูป
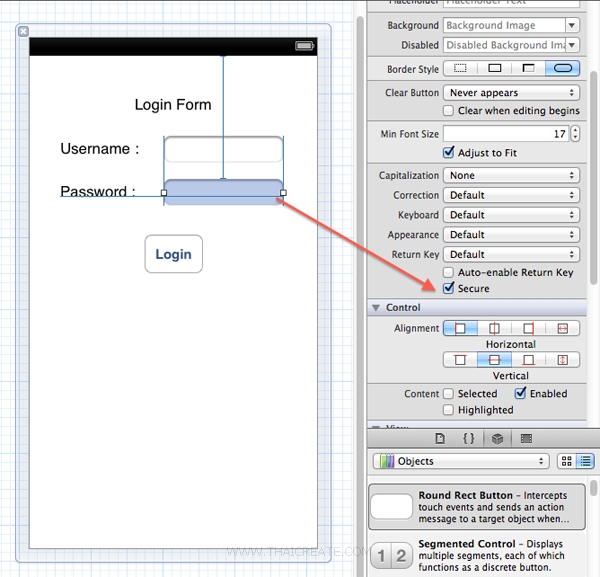
ช่อง Password ให้เลือกเป็นแบบ Secure
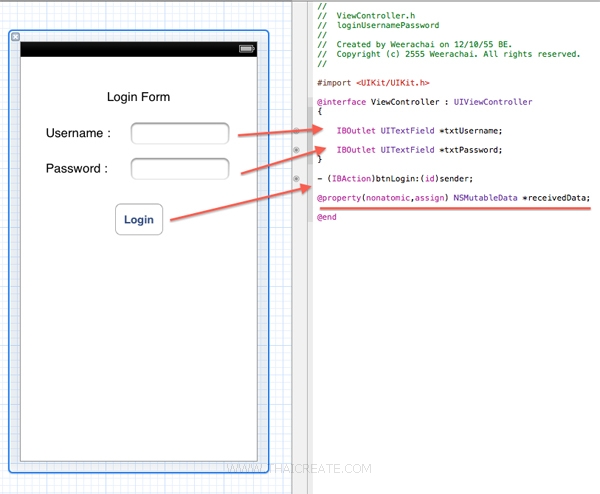
ใน Class ของ .h ให้ทำการเชื่อม IBOutlet และ IBAction ให้เรียบร้อย จากนั้นเขียน Code ต่าง ๆ ดังนี้
ViewController.h
//
// ViewController.h
// loginUsernamePassword
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
{
IBOutlet UITextField *txtUsername;
IBOutlet UITextField *txtPassword;
}
- (IBAction)btnLogin:(id)sender;
@property(nonatomic,assign) NSMutableData *receivedData;
@end
ViewController.m
//
// ViewController.m
// loginUsernamePassword
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
#import "LoginInfoViewController.h"
@interface ViewController ()
{
UIAlertView *loading;
}
@end
@implementation ViewController
@synthesize receivedData;
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
}
- (IBAction)btnLogin:(id)sender {
// Show Progress Loading...
[UIApplication sharedApplication].networkActivityIndicatorVisible = YES;
loading = [[UIAlertView alloc] initWithTitle:@"" message:@"Login Checking..." delegate:nil cancelButtonTitle:nil otherButtonTitles:nil];
UIActivityIndicatorView *progress= [[UIActivityIndicatorView alloc] initWithFrame:CGRectMake(125, 50, 30, 30)];
progress.activityIndicatorViewStyle = UIActivityIndicatorViewStyleWhiteLarge;
[loading addSubview:progress];
[progress startAnimating];
[progress release];
[loading show];
//sUsername=weerachai&sPassword=weerachai@1"
NSMutableString *post = [NSString stringWithFormat:@"sUsername=%@&sPassword=%@",[txtUsername text],[txtPassword text]];
NSData *postData = [post dataUsingEncoding:NSASCIIStringEncoding allowLossyConversion:YES];
NSString *postLength = [NSString stringWithFormat:@"%d", [postData length]];
NSURL *url = [NSURL URLWithString:@"https://www.thaicreate.com/url/checkLogin.php"];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setValue:postLength forHTTPHeaderField:@"Content-Length"];
[request setValue:@"application/x-www-form-urlencoded" forHTTPHeaderField:@"Content-Type"];
[request setHTTPBody:postData];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:request delegate:self];
if (theConnection) {
self.receivedData = nil;
} else {
UIAlertView *connectFailMessage = [[UIAlertView alloc] initWithTitle:@"NSURLConnection " message:@"Failed in viewDidLoad" delegate: self cancelButtonTitle:@"Ok" otherButtonTitles: nil];
[connectFailMessage show];
[connectFailMessage release];
}
}
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
{
receivedData = [[NSMutableData alloc] init];
}
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
{
sleep(3);
[receivedData appendData:data];
}
- (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error
{
[connection release];
[receivedData release];
// inform the user
UIAlertView *didFailWithErrorMessage = [[UIAlertView alloc] initWithTitle: @"NSURLConnection " message: @"didFailWithError" delegate: self cancelButtonTitle: @"Ok" otherButtonTitles: nil];
[didFailWithErrorMessage show];
[didFailWithErrorMessage release];
//inform the user
NSLog(@"Connection failed! Error - %@", [error localizedDescription]);
}
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
// Hide Progress
[UIApplication sharedApplication].networkActivityIndicatorVisible = NO;
[loading dismissWithClickedButtonIndex:0 animated:YES];
// Return Status E.g : { "Status":"1", "MemberID":"1", "Message":"Login Successfully" }
// 0 = Error
// 1 = Completed
if(receivedData)
{
//NSLog(@"%@",receivedData);
//NSString *dataString = [[NSString alloc] initWithData:receivedData encoding:NSASCIIStringEncoding];
//NSLog(@"%@",dataString);
id jsonObjects = [NSJSONSerialization JSONObjectWithData:receivedData options:NSJSONReadingMutableContainers error:nil];
// value in key name
NSString *strStatus = [jsonObjects objectForKey:@"Status"];
NSString *strMemberID = [jsonObjects objectForKey:@"MemberID"];
NSString *strMessage = [jsonObjects objectForKey:@"Message"];
NSLog(@"Status = %@",strStatus);
NSLog(@"MemberID = %@",strMemberID);
NSLog(@"Message = %@",strMessage);
// Login Completed
if( [strStatus isEqualToString:@"1"] ){
/*
UIAlertView *completed =[[UIAlertView alloc]
initWithTitle:@" Completed!"
message:strMessage delegate:self
cancelButtonTitle:@"OK" otherButtonTitles: nil];
[completed show];
*/
LoginInfoViewController *viewInfo = [[[LoginInfoViewController alloc] initWithNibName:nil bundle:nil] autorelease];
viewInfo.sMemberID = strMemberID;
[self presentViewController:viewInfo animated:NO completion:NULL];
}
else // Login Failed
{
UIAlertView *error =[[UIAlertView alloc]
initWithTitle:@":( Error!"
message:strMessage delegate:self
cancelButtonTitle:@"OK" otherButtonTitles: nil];
[error show];
}
}
// release the connection, and the data object
[connection release];
[receivedData release];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[txtUsername release];
[txtPassword release];
[super dealloc];
}
@end
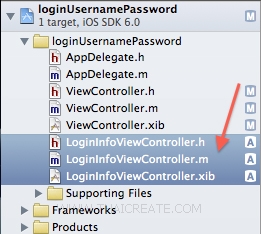
สร้าง View Interface ขึ้นมาอีก 1 ตัว ชื่อว่า LoginInfoViewController โดยใช้ Subclass ของ UIViewController ซึ่งเราจะได้ไฟล์ขึ้นมา 3 ตัวคือ .xib , .m และ .h ดังรูป
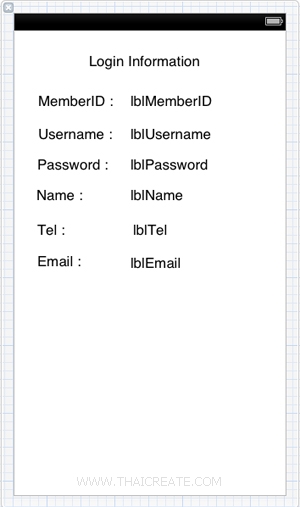
ออกแบบหน้าจอด้วย Label ต่าง ๆ ดังรูป
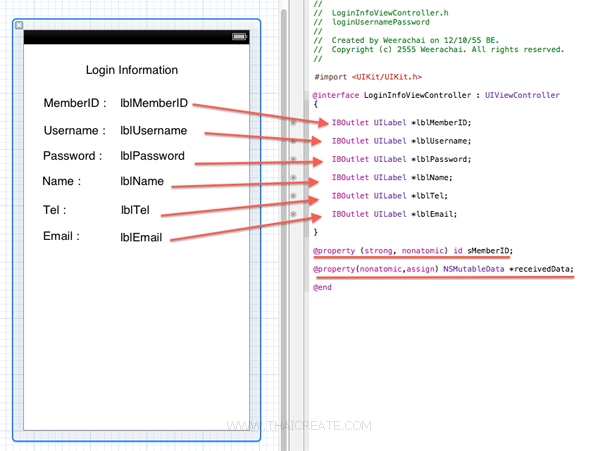
ใน Class ของ .h ให้ทำการเชื่อม IBOutlet ต่าง ๆ และ Code ต่าง ๆ ดังนี้
LoginInfoViewController.h
//
// LoginInfoViewController.h
// loginUsernamePassword
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface LoginInfoViewController : UIViewController
{
IBOutlet UILabel *lblMemberID;
IBOutlet UILabel *lblUsername;
IBOutlet UILabel *lblPassword;
IBOutlet UILabel *lblName;
IBOutlet UILabel *lblTel;
IBOutlet UILabel *lblEmail;
}
@property (strong, nonatomic) id sMemberID;
@property(nonatomic,assign) NSMutableData *receivedData;
@end
LoginInfoViewController.m
//
// LoginInfoViewController.m
// loginUsernamePassword
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "LoginInfoViewController.h"
@interface LoginInfoViewController ()
@end
@implementation LoginInfoViewController
@synthesize receivedData;
- (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil
{
self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view from its nib.
//sMemberID=1
NSMutableString *post = [NSString stringWithFormat:@"sMemberID=%@",[self.sMemberID description]];
NSData *postData = [post dataUsingEncoding:NSASCIIStringEncoding allowLossyConversion:YES];
NSString *postLength = [NSString stringWithFormat:@"%d", [postData length]];
NSURL *url = [NSURL URLWithString:@"https://www.thaicreate.com/url/getUserByMemberID.php"];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setValue:postLength forHTTPHeaderField:@"Content-Length"];
[request setValue:@"application/x-www-form-urlencoded" forHTTPHeaderField:@"Content-Type"];
[request setHTTPBody:postData];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:request delegate:self];
if (theConnection) {
self.receivedData = nil;
} else {
UIAlertView *connectFailMessage = [[UIAlertView alloc] initWithTitle:@"NSURLConnection " message:@"Failed in viewDidLoad" delegate: self cancelButtonTitle:@"Ok" otherButtonTitles: nil];
[connectFailMessage show];
[connectFailMessage release];
}
}
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
{
receivedData = [[NSMutableData alloc] init];
}
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
{
[receivedData appendData:data];
}
- (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error
{
[connection release];
[receivedData release];
// inform the user
UIAlertView *didFailWithErrorMessage = [[UIAlertView alloc] initWithTitle: @"NSURLConnection " message: @"didFailWithError" delegate: self cancelButtonTitle: @"Ok" otherButtonTitles: nil];
[didFailWithErrorMessage show];
[didFailWithErrorMessage release];
//inform the user
NSLog(@"Connection failed! Error - %@", [error localizedDescription]);
}
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
// Return Status E.g : { "MemberID":"1", "Username":"weerachai", "Password":"weerachai@1" , "Name":"Weerachai Nukitram" , "Email":", "Password":"Login Successfully" " , "Tel":"0819876107" }
if(receivedData)
{
//NSLog(@"%@",receivedData);
//NSString *dataString = [[NSString alloc] initWithData:receivedData encoding:NSASCIIStringEncoding];
//NSLog(@"%@",dataString);
id jsonObjects = [NSJSONSerialization JSONObjectWithData:receivedData options:NSJSONReadingMutableContainers error:nil];
// value in key name
NSString *strMemberID = [jsonObjects objectForKey:@"MemberID"];
NSString *strUsername = [jsonObjects objectForKey:@"Username"];
NSString *strPassword = [jsonObjects objectForKey:@"Password"];
NSString *strName = [jsonObjects objectForKey:@"Name"];
NSString *strTel = [jsonObjects objectForKey:@"Tel"];
NSString *strEmail = [jsonObjects objectForKey:@"Email"];
NSLog(@"MemberID = %@",strMemberID);
NSLog(@"Username = %@",strUsername);
NSLog(@"Password = %@",strPassword);
NSLog(@"Name = %@",strName);
NSLog(@"Tel = %@",strTel);
NSLog(@"Email = %@",strEmail);
lblMemberID.text = strMemberID;
lblUsername.text = strUsername;
lblPassword.text = strPassword;
lblName.text = strName;
lblTel.text = strTel;
lblEmail.text = strEmail;
}
// release the connection, and the data object
[connection release];
[receivedData release];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[lblMemberID release];
[lblPassword release];
[lblUsername release];
[lblPassword release];
[lblName release];
[lblTel release];
[lblEmail release];
[super dealloc];
}
@end
Screenshot
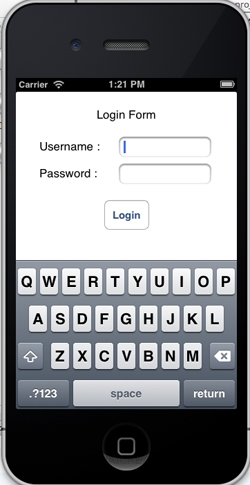
แสดงหน้าจอสำหรับ Login ด้วย Username และ Password
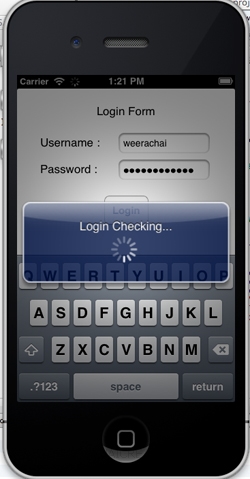
กำลัง Login ตรวจสอบข้อมูล
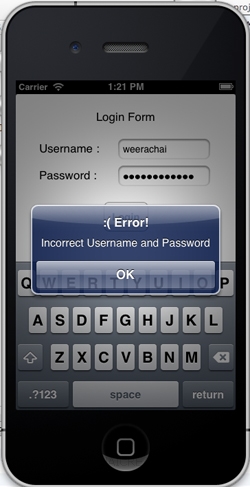
ในกรณีที่ Username และ Password ไม่ถูกต้อง
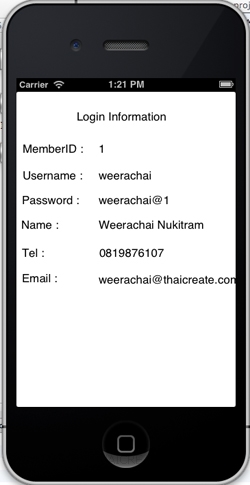
หลังจากที่ Login ผ่านแล้วก ก็จะแสดงข้อมูลผู้ที่ได้ทำการ Login เข้ามาในระบบ
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-12-13 12:28:24 /
2017-03-26 08:50:38 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|