iOS/iPhone Register Form and Send Data to Web Server (PHP & MySQL) |
iOS/iPhone Register Form and Send Data to Web Server (PHP & MySQL) บทความนี้จะเป็นการเขียน iOS ทำงานร่วมกับ iPhone App โดยสมมุติการลงทะเบียนข้อมูลสมาชิกผ่าน App ของ iPhone และในมุมมองของการเขียนโปรแกรม เราจะใช้ NSURLConnection เพื่อส่งค่า POST ต่าง ๆ ที่ผู้ใช้ได้กรอกในหน้าจอ App โดยจะส่งไปยัง URL ของ Web Server ที่ทำหน้าที่จัดเก็บข้อมูลด้วย MySQL Database ซึ่งในฝั่งของ Web Server ก็จะใช้ PHP ทำการตรวจสอบข้อมูลต่าง ๆ ก่อนที่จะ Insert ลงในฐานข้อมูลอีกครั้ง
iOS/iPhone Register Form and Send Data to Web Server (PHP & MySQL)
ในตัวอย่างนี้จะเหมือนกับตัวอย่างการ Insert ข้อมูลจาก iOS ไปยัง Web Server แต่จะเพิ่มคุณสมบัติการตรวจสอบข้อมูลต่าง ๆ ก่อนที่จะ Inset ลงใน Database เช่น ตรวจสอบ User , Email ว่าข้อมูลต่าง ๆ เหล่านี้ได้มีใน Database แล้วหรือยัง ถ้ามีแล้วก็จะแจ้งให้ User ได้ทราบทันที
iOS/iPhone Add Insert Data to Web Server (URL,Website)
Example การทำ Form สำหรับ Register ข้อมูล และส่งข้อมูลไปจัดเก็บที่ Web Server
MySQL Database
CREATE TABLE `member` (
`MemberID` int(2) NOT NULL auto_increment,
`Username` varchar(50) NOT NULL,
`Password` varchar(50) NOT NULL,
`Name` varchar(50) NOT NULL,
`Tel` varchar(50) NOT NULL,
`Email` varchar(150) NOT NULL,
PRIMARY KEY (`MemberID`),
UNIQUE KEY `Username` (`Username`),
UNIQUE KEY `Email` (`Email`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
--
-- Dumping data for table `member`
--
INSERT INTO `member` VALUES (1, 'weerachai', 'weerachai@1', 'Weerachai Nukitram', '0819876107', '[email protected]');
INSERT INTO `member` VALUES (2, 'adisorn', 'adisorn@2', 'Adisorn Bunsong', '021978032', '[email protected]');
INSERT INTO `member` VALUES (3, 'surachai', 'surachai@3', 'Surachai Sirisart', '0876543210', '[email protected]');
โครงสร้างของ MySQL Database

registerData.php ไฟล์ php สำหรับจัดเก็บข้อมูล
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
/*** for Sample
$_POST["sUsername"] = "a";
$_POST["sPassword"] = "b";
$_POST["sName"] = "c";
$_POST["sEmail"] = "d";
$_POST["sTel"] = "e";
*/
$strUsername = $_POST["sUsername"];
$strPassword = $_POST["sPassword"];
$strName = $_POST["sName"];
$strEmail = $_POST["sEmail"];
$strTel = $_POST["sTel"];
/*** Check Username Exists ***/
$strSQL = "SELECT * FROM member WHERE Username = '".$strUsername."' ";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
$arr = null;
if($objResult)
{
$arr["Status"] = "0";
$arr["Message"] = "Username Exists!";
echo json_encode($arr);
exit();
}
/*** Check Email Exists ***/
$strSQL = "SELECT * FROM member WHERE Email = '".$strEmail."' ";
$objQuery = mysql_query($strSQL);
$objResult = mysql_fetch_array($objQuery);
if($objResult)
{
$arr["Status"] = "0";
$arr["Message"] = "Email Exists!";
echo json_encode($arr);
exit();
}
/*** Insert ***/
$strSQL = "INSERT INTO member (Username,Password,Name,Email,Tel)
VALUES (
'".$strUsername."',
'".$strPassword."',
'".$strName."',
'".$strEmail."',
'".$strTel."'
)
";
$objQuery = mysql_query($strSQL);
if(!$objQuery)
{
$arr["Status"] = "0";
$arr["Message"] = "Cannot Save Data!";
echo json_encode($arr);
exit();
}
else
{
$arr["Status"] = "1";
$arr["Message"] = "Register Successfully!";
echo json_encode($arr);
exit();
}
/**
return
Status // (0=Failed , 1=Complete)
Message // Message
*/
mysql_close($objConnect);
?>

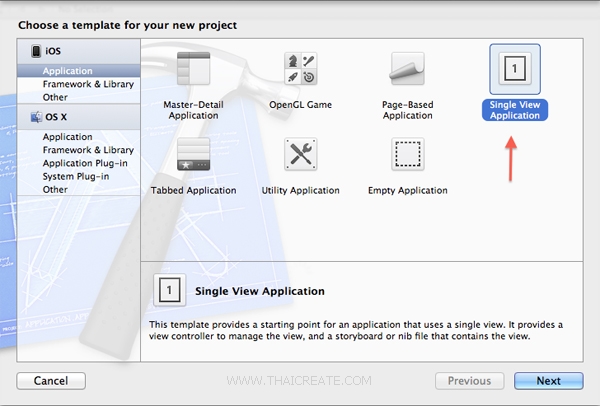
เริ่มต้นด้วยการสร้าง Application บน Xcode แบบ Single View Applcation
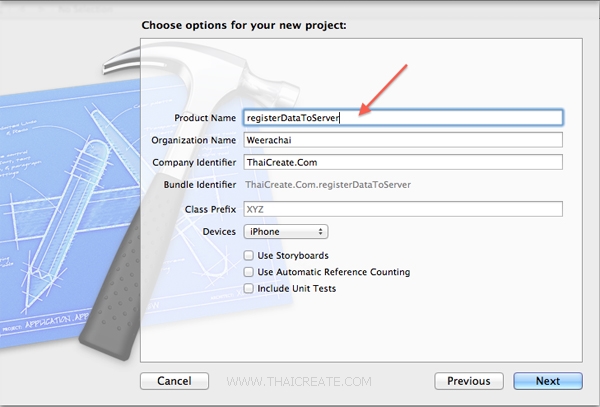
เลือกและไม่เลือกรายการดังรูป
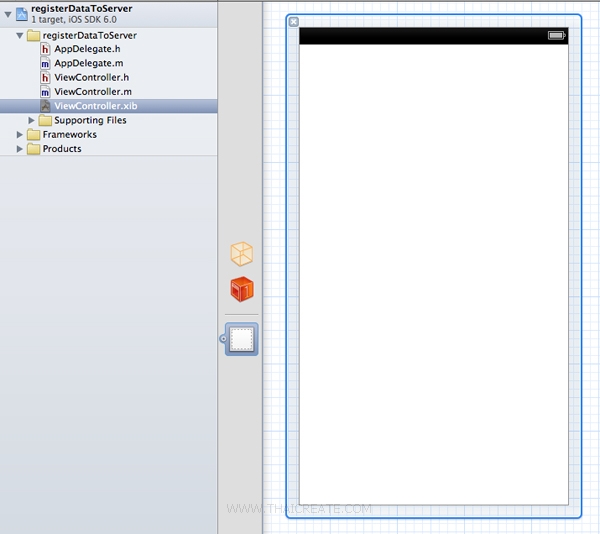
ตอนนี้หน้าจอ View ของเราจะยังว่าง ๆ
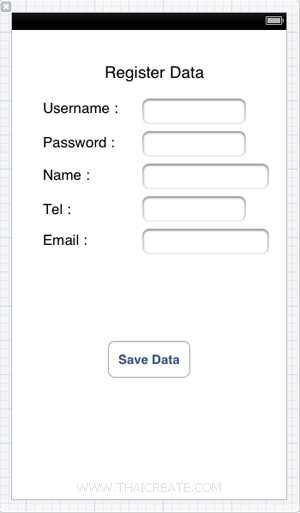
ออกแบบ Register Form ด้วย Label , Text Fields และ Button ดังรูป
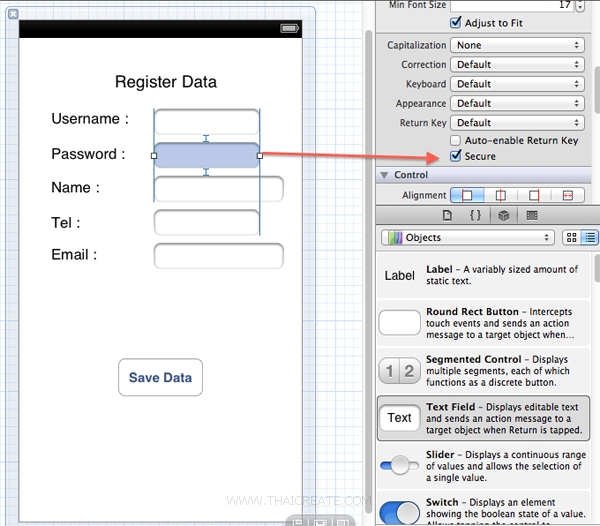
ตรง Password ให้คลิกเลือก Secure
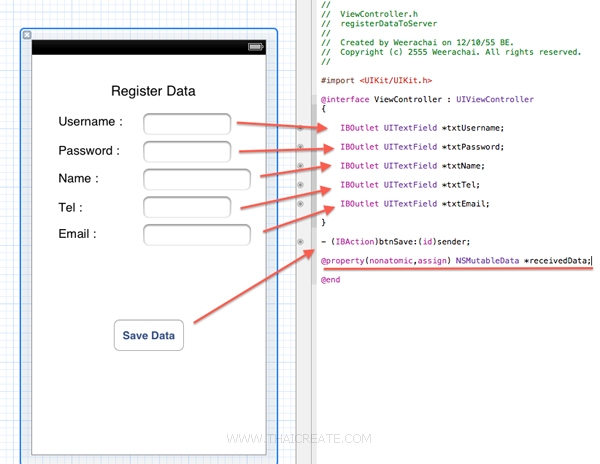
จากนั้นใน Class ของ .h ให้เชื่อม IBOutlet และ IBAction ดังรูป และเขียน Code ต่าง ๆ ดังนี้
ViewController.h
//
// ViewController.h
// registerDataToServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
{
IBOutlet UITextField *txtUsername;
IBOutlet UITextField *txtPassword;
IBOutlet UITextField *txtName;
IBOutlet UITextField *txtTel;
IBOutlet UITextField *txtEmail;
}
- (IBAction)btnSave:(id)sender;
@property(nonatomic,assign) NSMutableData *receivedData;
@end
ViewController.m
//
// ViewController.m
// registerDataToServer
//
// Created by Weerachai on 12/10/55 BE.
// Copyright (c) 2555 Weerachai. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
{
UIAlertView *loading;
}
@end
@implementation ViewController
@synthesize receivedData;
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
// Tap Gesture for Hide Keyboard
UITapGestureRecognizer *oneTapGesture = [[UITapGestureRecognizer alloc]
initWithTarget: self
action: @selector(hideKeyboard:)];
[oneTapGesture setNumberOfTouchesRequired:1];
[[self view] addGestureRecognizer:oneTapGesture];
}
// Event Gesture for Hide Keyboard
- (void)hideKeyboard:(UITapGestureRecognizer *)sender {
[txtUsername resignFirstResponder];
[txtPassword resignFirstResponder];
[txtName resignFirstResponder];
[txtTel resignFirstResponder];
[txtEmail resignFirstResponder];
}
- (IBAction)btnSave:(id)sender {
//sUsername=surapong&sPassword=surapong@4&sName=Surapong Siriphun&[email protected]&sTel=0812345678
NSMutableString *post = [NSString stringWithFormat:@"sUsername=%@&sPassword=%@&sName=%@&sEmail=%@&sTel=%@",
[txtUsername text],[txtPassword text],[txtName text],[txtEmail text],[txtTel text]];
NSData *postData = [post dataUsingEncoding:NSASCIIStringEncoding allowLossyConversion:YES];
NSString *postLength = [NSString stringWithFormat:@"%d", [postData length]];
NSURL *url = [NSURL URLWithString:@"https://www.thaicreate.com/url/registerData.php"];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:url
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setValue:postLength forHTTPHeaderField:@"Content-Length"];
[request setValue:@"application/x-www-form-urlencoded" forHTTPHeaderField:@"Content-Type"];
[request setHTTPBody:postData];
NSURLConnection *theConnection=[[NSURLConnection alloc] initWithRequest:request delegate:self];
// Show Progress Loading...
[UIApplication sharedApplication].networkActivityIndicatorVisible = YES;
loading = [[UIAlertView alloc] initWithTitle:@"" message:@"Please Wait..." delegate:nil cancelButtonTitle:nil otherButtonTitles:nil];
UIActivityIndicatorView *progress= [[UIActivityIndicatorView alloc] initWithFrame:CGRectMake(125, 50, 30, 30)];
progress.activityIndicatorViewStyle = UIActivityIndicatorViewStyleWhiteLarge;
[loading addSubview:progress];
[progress startAnimating];
[progress release];
[loading show];
if (theConnection) {
self.receivedData = nil;
} else {
UIAlertView *connectFailMessage = [[UIAlertView alloc] initWithTitle:@"NSURLConnection " message:@"Failed in viewDidLoad" delegate: self cancelButtonTitle:@"Ok" otherButtonTitles: nil];
[connectFailMessage show];
[connectFailMessage release];
}
}
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
{
receivedData = [[NSMutableData alloc] init];
}
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
{
sleep(2);
[receivedData appendData:data];
}
- (void)connection:(NSURLConnection *)connection didFailWithError:(NSError *)error
{
[connection release];
[receivedData release];
// inform the user
UIAlertView *didFailWithErrorMessage = [[UIAlertView alloc] initWithTitle: @"NSURLConnection " message: @"didFailWithError" delegate: self cancelButtonTitle: @"Ok" otherButtonTitles: nil];
[didFailWithErrorMessage show];
[didFailWithErrorMessage release];
//inform the user
NSLog(@"Connection failed! Error - %@", [error localizedDescription]);
}
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
// Hide Progress
[UIApplication sharedApplication].networkActivityIndicatorVisible = NO;
[loading dismissWithClickedButtonIndex:0 animated:YES];
// Return Status E.g : { "Status":"1", "Message":"Register Successfully" }
// 0 = Error
// 1 = Completed
if(receivedData)
{
//NSLog(@"%@",receivedData);
//NSString *dataString = [[NSString alloc] initWithData:receivedData encoding:NSASCIIStringEncoding];
//NSLog(@"%@",dataString);
id jsonObjects = [NSJSONSerialization JSONObjectWithData:receivedData options:NSJSONReadingMutableContainers error:nil];
// value in key name
NSString *strStatus = [jsonObjects objectForKey:@"Status"];
NSString *strMessage = [jsonObjects objectForKey:@"Message"];
NSLog(@"Status = %@",strStatus);
NSLog(@"Message = %@",strMessage);
// Completed
if( [strStatus isEqualToString:@"1"] ){
UIAlertView *completed =[[UIAlertView alloc]
initWithTitle:@": -) Completed!"
message:strMessage delegate:self
cancelButtonTitle:@"OK" otherButtonTitles: nil];
[completed show];
}
else // Error
{
UIAlertView *error =[[UIAlertView alloc]
initWithTitle:@": ( Error!"
message:strMessage delegate:self
cancelButtonTitle:@"OK" otherButtonTitles: nil];
[error show];
}
}
// release the connection, and the data object
[connection release];
[receivedData release];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (void)dealloc {
[txtUsername release];
[txtPassword release];
[txtName release];
[txtTel release];
[txtEmail release];
[super dealloc];
}
@end

Screenshot
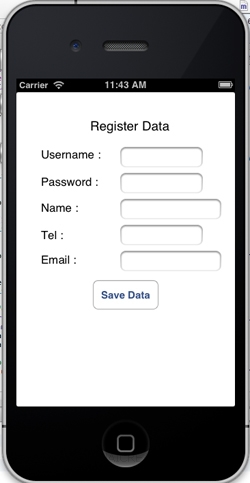
หน้าจอ Form View สำหรับกรอกข้อมูล
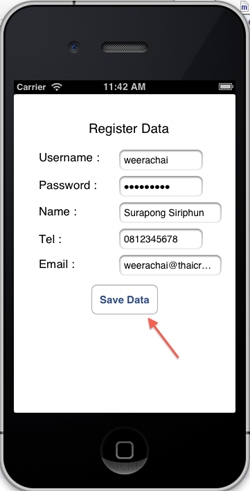
ทดสอบกรอกข้อมูล
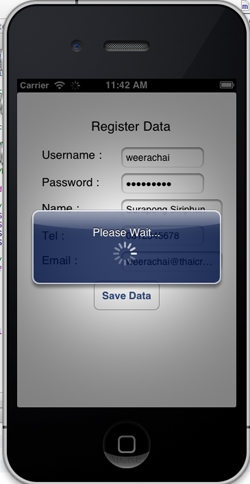
กำลังส่งข้อมูลไปยัง Web Server
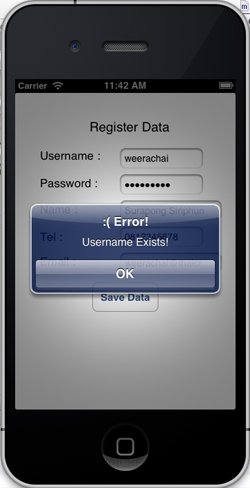
กรณีที่ User ซ้ำ จะแจ้ง Error ดังรูป
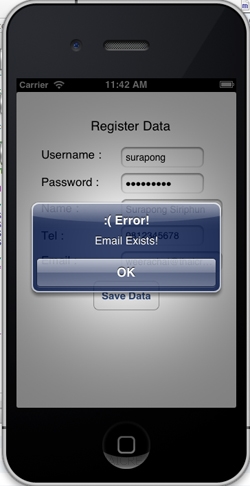
กรณีที่ Email ซ้ำ จะแจ้ง Error ดังรูป
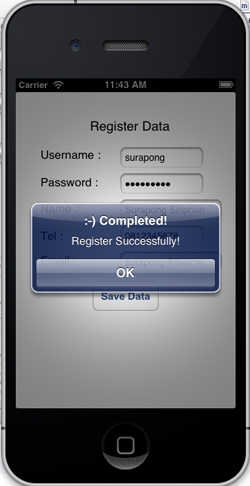
กรณีที่ Insert ผ่านสมบูรณ์

เมื่อกลับไปดูที่ Database MySQL ที่อยู่บน Web Server ก็จะพบกับ Record รายการที่ถูก Insert เข้าไปใหม่
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2012-12-13 12:28:08 /
2017-03-26 08:51:39 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|
|