Windows Store Apps and MS Access Database (C#) |
Windows Store Apps and MS Access Database (C#) บทความนี้จะเป็นการเขียน Windows Store Apps เพื่อติดต่อกับ MS Access Database ด้วยการอ่านข้อมูลที่อยู่บน Table (ตาราง) มาแสดงบนหน้าจอ Apps แบบง่าย ๆ ก่อนที่จะเขียนติดต่อกับ MS Access Database นั้นให้ทำความเข้าใจนิดหนึ่งก่อนว่า WinRT API นั้นจะไม่มี Class ที่เกี่ยวข้องกับ System.Data และ System.Data.OleDb ฉะนั้นการที่จะเขียน Windows Store Apps เพื่อติดต่อกับ MS Access Database โดยตรงเหมือนกับ .NET Application ทั่ว ๆ ไป นั้นจะใช้ไมได้กับ Windows Store Apps ฉะนั้นทางเลือกที่เราจะเขียนติดต่อกันได้คือจะต้องผ่านตัวกลาง อาจจะอยู่ในรูปแบบของ API ,Restful หรือ Web Services แต่วิธที่จะแนะนำและง่ายที่สุดคือ Web Services เพราะมีรูปแบบการใช้งานที่ง่าย โดยเราสามารถเขียนและออกแบบ Method บน Web Services ต่าง ๆ จากนั้นเพียงแค่ใช้ Windows Store Apps ไป Call ตัว Method ที่อยู่บน Web Services ก็จะได้ค่าต่าง ๆ ที่ต้องการกลับมา
Windows Store Apps and MS Access Database (C#)
ซึ่งรูปแบบการใช้ Web Services กับ MS Access Database นั้นเราจะต้องมีตัวกลาง ก็คือจะต้องมี Web Services ที่ทำหน้าที่คอยบริการ Services และติดต่อกับ MS Access Database อยุ่ตลอดเวลา
ตัวอย่างฐานข้อมูลที่อยู่บน MS Access Database

โครงสร้าง Table บน MS Access Database
ขั้นตอนที่ 1 การสร้าง Web Services เพื่อเรียกใช้งาน MS Access ซึ่งในบทความนี้จะใช้ ASP.Net เป็นตัวที่จะทำหน้าที่เป็น Web Services
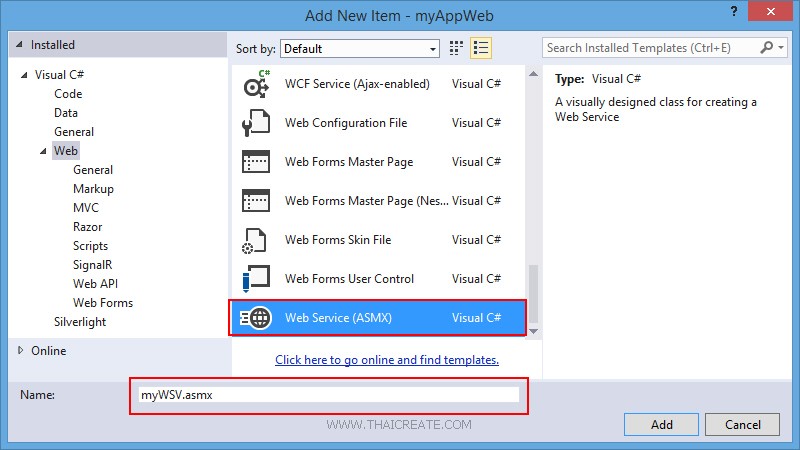
ในการสร้าง Web Services ด้วย ASP.Net ให้เลือกสร้าง Item ชื่อว่า Web Services (ASMX) โดยไฟล์ Web Services ของ ASP.Net จะได้นามสกุลไฟล์เป็น .asmx
เรียกใช้งาน Class สำหรับติดต่อกับ MS Access และการสร้าง JSON
using System.Data;
using Newtonsoft.Json;
using System.Data.OleDb;
คำสั่งการติดต่อกับ MS Access
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" + Server.MapPath("database/mydatabase.mdb") + ";Jet OLEDB:Database Password=;";
strSQL = "SELECT * FROM customer";
objConn.ConnectionString = strConnString;
objCmd.Connection = objConn;
objCmd.CommandText = strSQL;
objCmd.CommandType = CommandType.Text;
การแปลงค่าเป็น JSON
string json = JsonConvert.SerializeObject(dt, Formatting.Indented);
return json;
Code ทั้งหมด
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Data;
using Newtonsoft.Json;
using System.Data.OleDb;
namespace myAppWeb
{
/// <summary>
/// Summary description for myWSV
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class myWSV : System.Web.Services.WebService
{
[WebMethod]
public string getCustomerData()
{
OleDbConnection objConn = new OleDbConnection();
OleDbCommand objCmd = new OleDbCommand();
OleDbDataAdapter dtAdapter = new OleDbDataAdapter();
DataSet ds = new DataSet();
DataTable dt;
String strConnString, strSQL;
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" + Server.MapPath("database/mydatabase.mdb") + ";Jet OLEDB:Database Password=;";
strSQL = "SELECT * FROM customer";
objConn.ConnectionString = strConnString;
objCmd.Connection = objConn;
objCmd.CommandText = strSQL;
objCmd.CommandType = CommandType.Text;
dtAdapter.SelectCommand = objCmd;
dtAdapter.Fill(ds);
dt = ds.Tables[0];
dtAdapter = null;
objConn.Close();
objConn = null;
string json = JsonConvert.SerializeObject(dt, Formatting.Indented);
return json;
}
}
}
เป็นไฟล์ Web Services และการติดต่อกับ MS Access Database ซึ่งเราจะส่งค่า JSON กลับมา
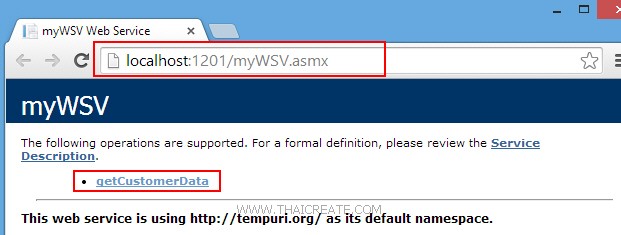
ทดสอบการทำงานของ Web Services
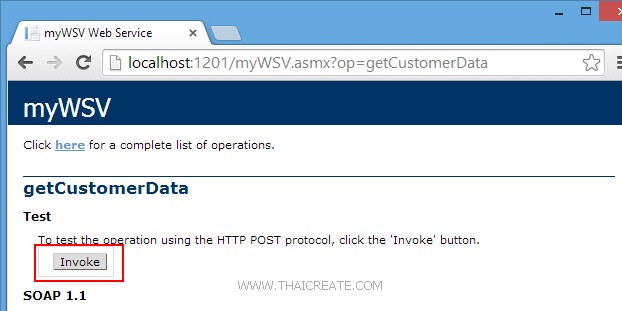
คลิก Invoke
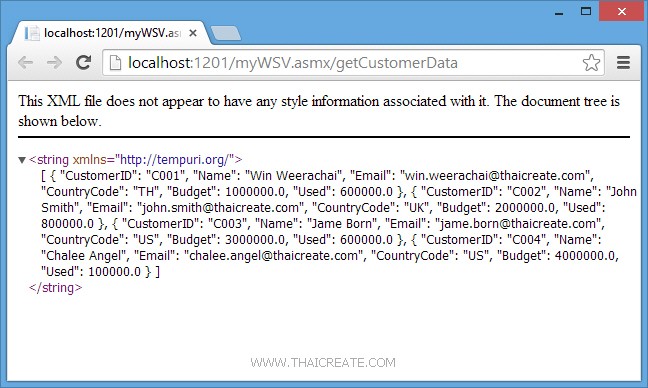
เป็นตัวอย่างไฟล์ JSON ที่ถูกส่งมาจาก Web Services
[ { "CustomerID": "C001", "Name": "Win Weerachai", "Email": "[email protected]", "CountryCode": "TH", "Budget": 1000000.0, "Used": 600000.0 }, { "CustomerID": "C002", "Name": "John Smith", "Email": "[email protected]", "CountryCode": "UK", "Budget": 2000000.0, "Used": 800000.0 }, { "CustomerID": "C003", "Name": "Jame Born", "Email": "[email protected]", "CountryCode": "US", "Budget": 3000000.0, "Used": 600000.0 }, { "CustomerID": "C004", "Name": "Chalee Angel", "Email": "[email protected]", "CountryCode": "US", "Budget": 4000000.0, "Used": 100000.0 } ]
ขั้นตอนที่ 2 การสร้าง Windows Store Apps เพื่อเรียกใช้งาน Web Services ที่ติดต่อกับ MS Access
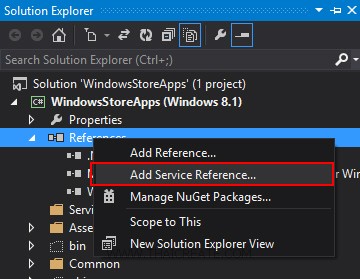
คลิกวาที่ Reference เลือก Add Service Reference
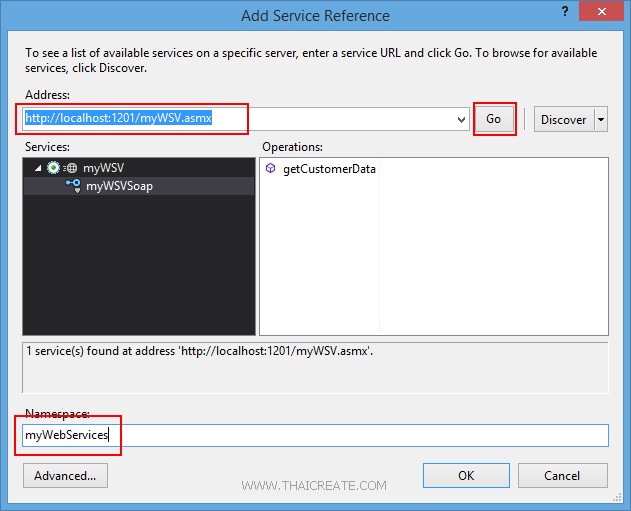
จากนั้นกรอก URL ของ Services และระบุชื่อ Services Name ที่ต้องการเรียกใช้งาน
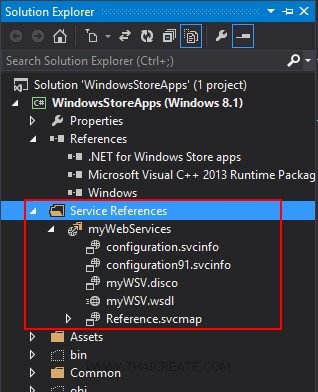
ได้ Services เรียบร้อยแล้ว จากนั้นเราจะเรียกใช้งาน Web Services บน Windows Store Apps
MainPage.xaml
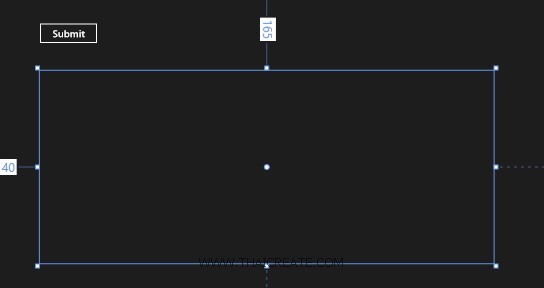
ออกแบบหน้าจอดังรูป
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Button x:Name="btnSubmit" Content="Submit" HorizontalAlignment="Left" Margin="137,68,0,0" VerticalAlignment="Top" FontSize="20" Width="120" Click="btnSubmit_Click"/>
<ListView x:Name="myListView" HorizontalAlignment="Left" Height="386" Margin="140,165,0,0" VerticalAlignment="Top" Width="907">
<ListView.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<StackPanel Width="80">
<TextBlock Text="{Binding CustomerID}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0" FontFamily="Global User Interface"/>
</StackPanel>
<StackPanel Width="150">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="300">
<TextBlock Text="{Binding Email}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="50">
<TextBlock Text="{Binding CountryCode}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Budget}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Used}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using WindowsStoreApps.myWebServices;
using System.Xml.Linq;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Json;
using System.Collections.ObjectModel;
using System.Text;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
private async void btnSubmit_Click(object sender, RoutedEventArgs e)
{
var client = new myWebServices.myWSVSoapClient();
var result = await client.getCustomerDataAsync();
string jsonData = result.Body.getCustomerDataResult;
MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(jsonData));
ObservableCollection<myCustomer> list = new ObservableCollection<myCustomer>();
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(ObservableCollection<myCustomer>));
list = (ObservableCollection<myCustomer>)serializer.ReadObject(ms);
this.myListView.ItemsSource = list;
}
}
}
Result
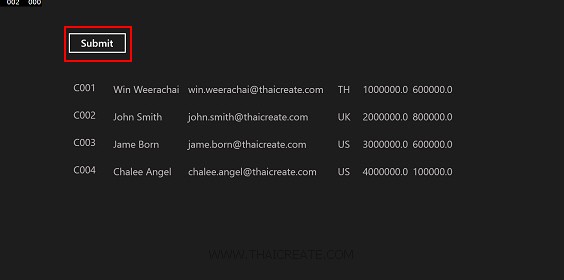
ค่าที่ได้จาก Web Services ที่อ่านจาก MS Access Database
อ่านเพิ่มเติม Windows Store App and Web Services (C#)
ตัวอย่างการ Insert ข้อมูล
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Data;
using Newtonsoft.Json;
using System.Data.OleDb;
namespace myAppWeb
{
/// <summary>
/// Summary description for myWSV
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class myWSV : System.Web.Services.WebService
{
[WebMethod]
public string AddData(string sCustomerID,
string sName,
string sEmail,
string sCountryCode,
string sBudget,
string sUsed)
{
try
{
OleDbConnection objConn = new OleDbConnection();
OleDbCommand objCmd = new OleDbCommand();
String strConnString, strSQL;
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" + Server.MapPath("database/mydatabase.mdb") + ";Jet OLEDB:Database Password=;";
objConn.ConnectionString = strConnString;
objConn.Open();
strSQL = "INSERT INTO customer (CustomerID,Name,Email,CountryCode,Budget,Used) VALUES " +
" ('" + sCustomerID + "' " +
" ,'" + sName + "' " +
" ,'" + sEmail + "' " +
" ,'" + sCountryCode + "' " +
" ,'" + sBudget + "' " +
" ,'" + sUsed + "') ";
objCmd = new OleDbCommand();
objCmd.Connection = objConn;
objCmd.CommandText = strSQL;
objCmd.CommandType = CommandType.Text;
objCmd.ExecuteNonQuery();
return "1"; // return sccess.
}
catch (Exception ex)
{
return "0"; // return failed.
}
}
}
}
private async void btnSave_Click(object sender, RoutedEventArgs e)
{
var client = new myWebServices.myWSVSoapClient();
var result = await client.AddDataAsync(this.txtCustomerID.Text,
this.txtName.Text,
this.txtEmail.Text,
this.txtCountryCode.Text,
this.txtBudget.Text,
this.txtUsed.Text);
string jsonData = result.Body.AddDataResult;
if (jsonData == "0")
{
MessageDialog msgDialog = new MessageDialog("Add Data Failed", "Error");
await msgDialog.ShowAsync();
}
else
{
MessageDialog msgDialog = new MessageDialog("Add Data Success.", "Success");
await msgDialog.ShowAsync();
}
}
ตัวอย่างการ Update ข้อมูล
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Data;
using Newtonsoft.Json;
using System.Data.OleDb;
namespace myAppWeb
{
/// <summary>
/// Summary description for myWSV
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class myWSV : System.Web.Services.WebService
{
[WebMethod]
public void UpdateData(string sCustomerID,
string sName,
string sEmail,
string sCountryCode,
string sBudget,
string sUsed)
{
OleDbConnection objConn = new OleDbConnection();
OleDbCommand objCmd = new OleDbCommand();
String strConnString, strSQL;
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" + Server.MapPath("database/mydatabase.mdb") + ";Jet OLEDB:Database Password=;";
objConn.ConnectionString = strConnString;
objConn.Open();
strSQL = "UPDATE customer SET " +
" Name = '" + sName + "' " +
" ,Email = '" + sEmail + "' " +
" ,CountryCode = '" + sCountryCode + "' " +
" ,Budget = '" + sBudget + "' " +
" ,Used = '" + sUsed + "' " +
" WHERE CustomerID = '" + sCustomerID + "' ";
objCmd = new OleDbCommand();
objCmd.Connection = objConn;
objCmd.CommandText = strSQL;
objCmd.CommandType = CommandType.Text;
objCmd.ExecuteNonQuery();
}
}
}
private async void btnSave_Click(object sender, RoutedEventArgs e)
{
var client = new myWebServices.myWSVSoapClient();
var result = await client.UpdateDataAsync(this.lblCustomerID.Text,
this.txtName.Text,
this.txtEmail.Text,
this.txtCountryCode.Text,
this.txtBudget.Text,
this.txtUsed.Text);
this.Frame.Navigate(typeof(MainPage));
}
ตัวอย่างการ Delete ข้อมูล
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Data;
using Newtonsoft.Json;
using System.Data.OleDb;
namespace myAppWeb
{
/// <summary>
/// Summary description for myWSV
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class myWSV : System.Web.Services.WebService
{
[WebMethod]
public void DeleteData(string sCustomerID)
{
OleDbConnection objConn = new OleDbConnection();
OleDbCommand objCmd = new OleDbCommand();
String strConnString, strSQL;
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" + Server.MapPath("database/mydatabase.mdb") + ";Jet OLEDB:Database Password=;";
strSQL = "DELETE FROM customer WHERE CustomerID ='" + sCustomerID + "'";
objConn.ConnectionString = strConnString;
objConn.Open();
objCmd = new OleDbCommand();
objCmd.Connection = objConn;
objCmd.CommandText = strSQL;
objCmd.CommandType = CommandType.Text;
objCmd.ExecuteNonQuery();
}
}
}
private async void btnDelete_Click(object sender, RoutedEventArgs e)
{
string CustomerID = "C001";
var client = new myWebServices.myWSVSoapClient();
var result = await client.DeleteDataAsync(CustomerID);
}
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2014-06-28 16:10:35 /
2017-03-19 15:09:58 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|