Windows Store Apps and SQL Server Database Part 1 (C#) |
Windows Store Apps and SQL Server Database Part 1 (C#) บทความนี้จะเป็นการเขียน Windows Store Apps เพื่อติดต่อกับ SQL Server Database ด้วยการอ่านข้อมูลที่อยู่บน Table (ตาราง) มาแสดงบนหน้าจอ Apps แบบง่าย ๆ ก่อนที่จะเขียนติดต่อกับ SQL Server นั้นให้ทำความเข้าใจนิดหนึ่งก่อนว่า WinRT API นั้นจะไม่มี Class ที่เกี่ยวข้องกับ System.Data และ System.Data.SqlClient ฉะนั้นการที่จะเขียน Windows Store Apps เพื่อติดต่อกับ SQL Server โดยตรงเหมือนกับ .NET Application ทั่ว ๆ ไป นั้นจะใช้ไมได้กับ Windows Store Apps ฉะนั้นทางเลือกที่เราจะเขียนติดต่อกันได้คือจะต้องผ่านตัวกลาง อาจจะอยู่ในรูปแบบของ API ,Restful หรือ Web Services แต่วิธที่จะแนะนำและง่ายที่สุดคือ Web Services เพราะมีรูปแบบการใช้งานที่ง่าย โดยเราสามารถเขียนและออกแบบ Method บน Web Services ต่าง ๆ จากนั้นเพียงแค่ใช้ Windows Store Apps ไป Call ตัว Method ที่อยู่บน Web Services ก็จะได้ค่าต่าง ๆ ที่ต้องการกลับมา
Windows Store Apps and SQL Server Database (C#)
ซึ่งรูปแบบการใช้ Web Services กับ SQL Server นั้นเราจะต้องมีตัวกลาง ก็คือจะต้องมี Web Services ที่ทำหน้าที่คอยบริการ Services และติดต่อกับ SQL Server Database อยุ่ตลอดเวลา
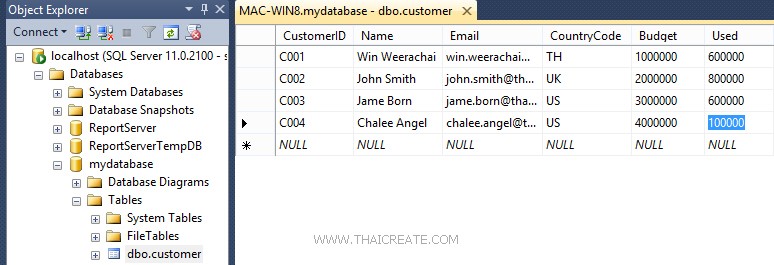
CREATE TABLE [dbo].[customer](
[CustomerID] [varchar](4) NOT NULL,
[Name] [varchar](50) NULL,
[Email] [varchar](50) NULL,
[CountryCode] [varchar](2) NULL,
[Budget] [float] NULL,
[Used] [float] NULL,
CONSTRAINT [PK_customer] PRIMARY KEY CLUSTERED
(
[CustomerID] ASC
))
INSERT INTO [customer] VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000)
INSERT INTO [customer] VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000)
INSERT INTO [customer] VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000)
INSERT INTO [customer] VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000)
ตัวอย่างฐานข้อมูลที่อยู่บน SQL Server Database
ขั้นตอนที่ 1 การสร้าง Web Services เพื่อเรียกใช้งาน SQL Server ซึ่งในบทความนี้จะใช้ ASP.Net เป็นตัวที่จะทำหน้าที่เป็น Web Services

ในการสร้าง Web Services ด้วย ASP.Net ให้เลือกสร้าง Item ชื่อว่า Web Services (ASMX) โดยไฟล์ Web Services ของ ASP.Net จะได้นามสกุลไฟล์เป็น .asmx

เรียกใช้งาน Class สำหรับติดต่อกับ SQL Server และการสร้าง JSON
using System.Data;
using Newtonsoft.Json;
using System.Data.SqlClient;
คำสั่งการติดต่อกับ SQL Server
strConnString = "Server=localhost;UID=sa;PASSWORD=mypassword;Database=mydatabase;Max Pool Size=400;Connect Timeout=600;";
strSQL = "SELECT * FROM customer";
objConn.ConnectionString = strConnString;
objCmd.Connection = objConn;
objCmd.CommandText = strSQL;
objCmd.CommandType = CommandType.Text;
การแปลงค่าเป็น JSON
string json = JsonConvert.SerializeObject(dt, Formatting.Indented);
return json;
Code ทั้งหมด
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Data;
using Newtonsoft.Json;
using System.Data.SqlClient;
namespace myAppWeb
{
/// <summary>
/// Summary description for myWSV
/// </summary>
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class myWSV : System.Web.Services.WebService
{
[WebMethod]
public string getCustomerData()
{
SqlConnection objConn = new SqlConnection();
SqlCommand objCmd = new SqlCommand();
SqlDataAdapter dtAdapter = new SqlDataAdapter();
DataSet ds = new DataSet();
DataTable dt;
String strConnString, strSQL;
strConnString = "Server=localhost;UID=sa;PASSWORD=mypassword;Database=mydatabase;Max Pool Size=400;Connect Timeout=600;";
strSQL = "SELECT * FROM customer";
objConn.ConnectionString = strConnString;
objCmd.Connection = objConn;
objCmd.CommandText = strSQL;
objCmd.CommandType = CommandType.Text;
dtAdapter.SelectCommand = objCmd;
dtAdapter.Fill(ds);
dt = ds.Tables[0];
dtAdapter = null;
objConn.Close();
objConn = null;
string json = JsonConvert.SerializeObject(dt, Formatting.Indented);
return json;
}
}
}
เป็นไฟล์ Web Services และการติดต่อกับ SQL Server Database ซึ่งเราจะส่งค่า JSON กลับมา
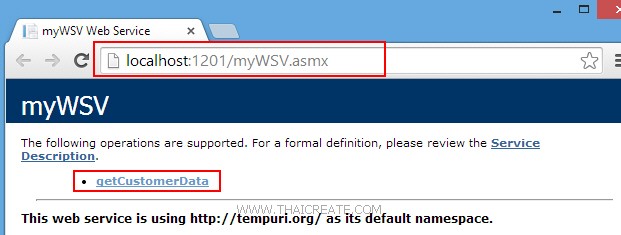
ทดสอบการทำงานของ Web Services
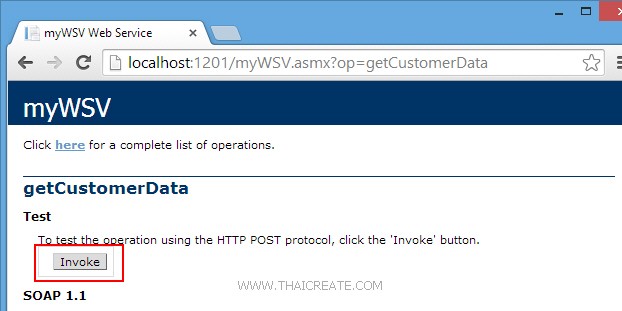
คลิก Invoke

เป็นตัวอย่างไฟล์ JSON ที่ถูกส่งมาจาก Web Services
[ { "CustomerID": "C001", "Name": "Win Weerachai", "Email": "[email protected]", "CountryCode": "TH", "Budget": 1000000.0, "Used": 600000.0 }, { "CustomerID": "C002", "Name": "John Smith", "Email": "[email protected]", "CountryCode": "UK", "Budget": 2000000.0, "Used": 800000.0 }, { "CustomerID": "C003", "Name": "Jame Born", "Email": "[email protected]", "CountryCode": "US", "Budget": 3000000.0, "Used": 600000.0 }, { "CustomerID": "C004", "Name": "Chalee Angel", "Email": "[email protected]", "CountryCode": "US", "Budget": 4000000.0, "Used": 100000.0 } ]
ขั้นตอนที่ 2 การสร้าง Windows Store Apps เพื่อเรียกใช้งาน Web Services ที่ติดต่อกับ SQL Server

คลิกวาที่ Reference เลือก Add Service Reference
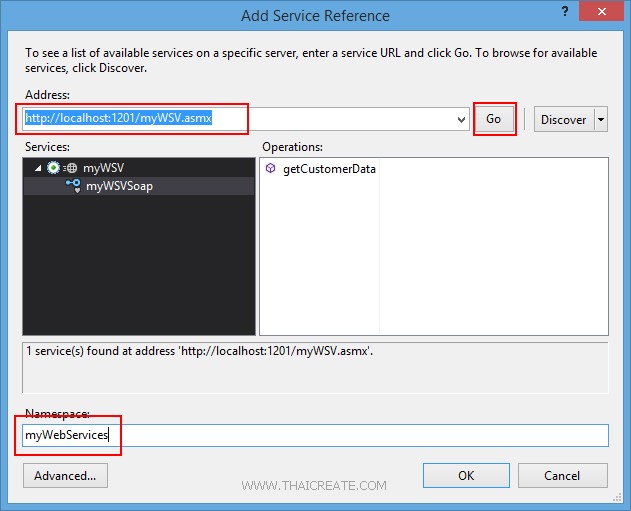
จากนั้นกรอก URL ของ Services และระบุชื่อ Services Name ที่ต้องการเรียกใช้งาน
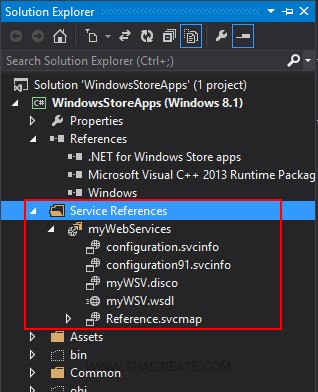
ได้ Services เรียบร้อยแล้ว จากนั้นเราจะเรียกใช้งาน Web Services บน Windows Store Apps
MainPage.xaml
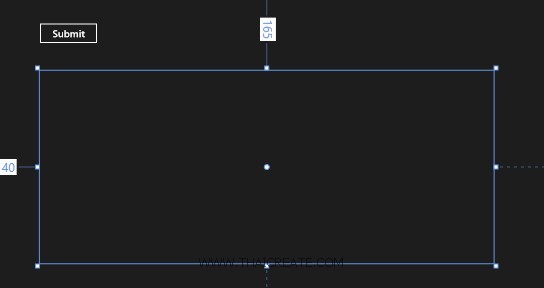
ออกแบบหน้าจอดังรูป
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Button x:Name="btnSubmit" Content="Submit" HorizontalAlignment="Left" Margin="137,68,0,0" VerticalAlignment="Top" FontSize="20" Width="120" Click="btnSubmit_Click"/>
<ListView x:Name="myListView" HorizontalAlignment="Left" Height="386" Margin="140,165,0,0" VerticalAlignment="Top" Width="907">
<ListView.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<StackPanel Width="80">
<TextBlock Text="{Binding CustomerID}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0" FontFamily="Global User Interface"/>
</StackPanel>
<StackPanel Width="150">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="300">
<TextBlock Text="{Binding Email}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="50">
<TextBlock Text="{Binding CountryCode}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Budget}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Used}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using WindowsStoreApps.myWebServices;
using System.Xml.Linq;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Json;
using System.Collections.ObjectModel;
using System.Text;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
private async void btnSubmit_Click(object sender, RoutedEventArgs e)
{
var client = new myWebServices.myWSVSoapClient();
var result = await client.getCustomerDataAsync();
string jsonData = result.Body.getCustomerDataResult;
MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(jsonData));
ObservableCollection<myCustomer> list = new ObservableCollection<myCustomer>();
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(ObservableCollection<myCustomer>));
list = (ObservableCollection<myCustomer>)serializer.ReadObject(ms);
this.myListView.ItemsSource = list;
}
}
}
Result
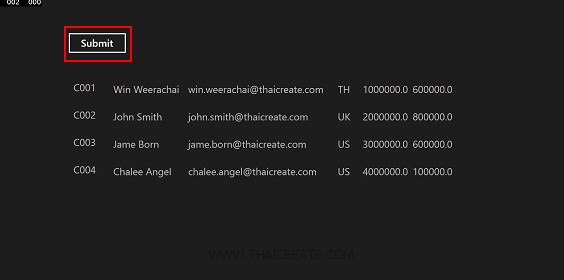
ค่าที่ได้จาก Web Services ที่อ่านจาก SQL Server Database
อ่านเพิ่มเติม Windows Store App and Web Services (C#)
.
|