Android EditText or Textbox in ListView |
Android EditText or Textbox in ListView ตัวอย่างการเขียน Android เพื่ออ้างถึงและอ่านข้อมูลจาก EditText หรือ Textbox ที่อยู่ใน ListView ซึ่ง EditText เหล่านี้จะแสดงแสดงผลตามจำนวนของรายการข้อมูล และเราจะเขียนคำสั่งเพื่ออ้างถึงรายการแต่ล่ะรายการที่ได้ Input เข้าไป
แสดง EditText หรือ Textbox ใน Items ของ ListView ซึ่งจะเห็นว่ามีหลายรายการ ขึ้นอยู่กับจำนวนแถวของข้อมูล
Example
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
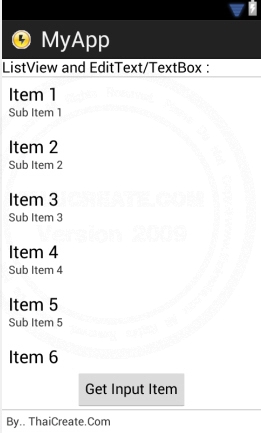
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="ListView and EditText/TextBox : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceMedium" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<RelativeLayout
android:layout_gravity="center_vertical|center_horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/btnGetItem"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:text="Get Input Item" />
</RelativeLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
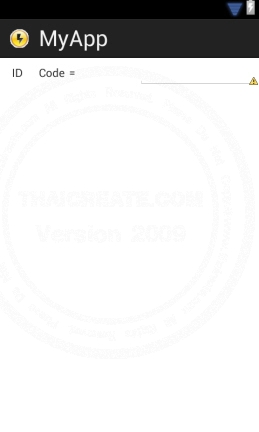
activity_column.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TextView
android:id="@+id/ColID"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center"
android:text="ID"/>
<TextView
android:id="@+id/ColCode"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center"
android:text="Code"/>
<TextView
android:id="@+id/ColCountry"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="=" />
<EditText
android:id="@+id/txtInput"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:ems="5" />
</LinearLayout>
MainActivity.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import android.os.Bundle;
import android.app.Activity;
import android.content.Context;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.LinearLayout;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
ArrayList<HashMap<String, String>> MyArrList;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// listView1
final ListView lisView1 = (ListView)findViewById(R.id.listView1);
MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
/*** Rows 1 ***/
map = new HashMap<String, String>();
map.put("ID", "1");
map.put("Code", "TH");
MyArrList.add(map);
/*** Rows 2 ***/
map = new HashMap<String, String>();
map.put("ID", "2");
map.put("Code", "VN");
MyArrList.add(map);
/*** Rows 3 ***/
map = new HashMap<String, String>();
map.put("ID", "3");
map.put("Code", "ID");
MyArrList.add(map);
/*** Rows 4 ***/
map = new HashMap<String, String>();
map.put("ID", "4");
map.put("Code", "LA");
MyArrList.add(map);
/*** Rows 5 ***/
map = new HashMap<String, String>();
map.put("ID", "5");
map.put("Code", "MY");
MyArrList.add(map);
lisView1.setAdapter(new CountryAdapter(this));
// Get Item Input
Button btnGetItem = (Button) findViewById(R.id.btnGetItem);
btnGetItem.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
int count = lisView1.getAdapter().getCount();
for (int i = 0; i < count; i++) {
LinearLayout itemLayout = (LinearLayout)lisView1.getChildAt(i); // Find by under LinearLayout
TextView txtCode = (TextView)itemLayout.findViewById(R.id.ColCode);
EditText txtInput = (EditText)itemLayout.findViewById(R.id.txtInput);
String Code = txtCode.getText().toString();
String Country = txtInput.getText().toString();
Log.d(Code, Country);
Toast.makeText(MainActivity.this, Code + " = " + Country ,Toast.LENGTH_LONG).show();
}
}
});
}
public class CountryAdapter extends BaseAdapter
{
private Context context;
public CountryAdapter(Context c)
{
//super( c, R.layout.activity_column, R.id.rowTextView, );
// TODO Auto-generated method stub
context = c;
}
public int getCount() {
// TODO Auto-generated method stub
return MyArrList.size();
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(final int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColID
TextView txtID = (TextView) convertView.findViewById(R.id.ColID);
txtID.setText(MyArrList.get(position).get("ID") +".");
// ColCode
TextView txtCode = (TextView) convertView.findViewById(R.id.ColCode);
txtCode.setText(MyArrList.get(position).get("Code"));
return convertView;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Screenshot

EditText หรือ Textbox ที่อยู่ใน ListView
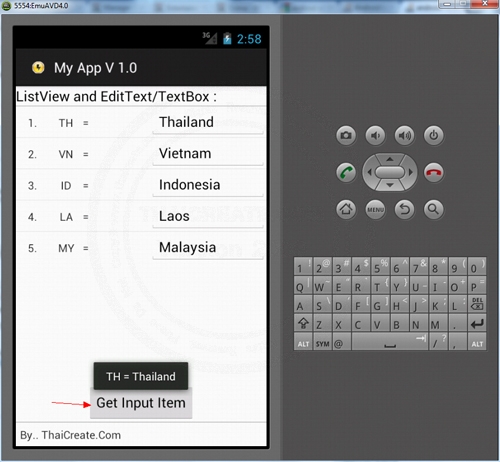
ทดสอบ Input ข้อมูลลงในแต่ล่ะช่องรายการของ Items
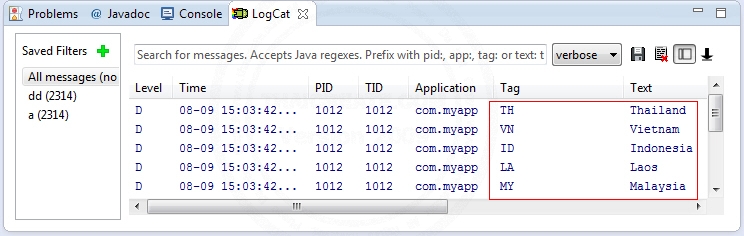
รายการ Item และ Input ที่ได้แสดงบน LogCat
สำหรับ Code และคำอธิบายสามารถศึกษาได้จาก Code ของ Java ซึ่งเป็นรูปแบบการทำงานง่าย ๆ ที่ทำงานคล้าย ๆ กับ Checkbox กับ GridView และ Checkbox กับ ListView
|