Android ListView and Buttons inside Create Custom Command in ListView |
Android ListView and Buttons Command บทความนี้จะเป็นการสร้าง ListView ซึ่งภายใน ListView ก็จะมีข้อมูลอยู่หลาย Rows และภายในแต่ล่ะ Rows จะมีปุ่ม Button ที่ทำหน้าที่บรรจุด Command หรือคำสั่งต่าง ๆ ที่จะทำงานกับ Rows นั้น ๆ เช่น การคลิกเพื่อดูรายละเอียด การคิกแล้วแสดงเหตุการณ์อื่น ๆ การลบข้อมูลในกรายการที่เลือก หรือการทำ Context Menu เพื่อทำรายการไปยังรายการอื่นด้วยการ Intent Activity เป็นต้น โดยปุ่มเหล่านี้สามารถเลือกใช้เป็น Button หรือ ImageButton หรือจะเป็น Widgets อื่น ๆ ก็สามารถทำได้เช่นเดียวกัน
Screenshot ของ ListView และการสร้าง Button Command
การสร้าง Event เหล่านี้ สามารถทำได้โดยการสร้างภายใต้ ImageAdapter ที่ทำหน้าที่กับ BaseAdapter และสามารถทำการ findViewById ของแต่ล่ะ Item ภายใต้ method ที่มีชื่อว่า getView()
public View getView(final int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View lView;
if (convertView == null) {
lView = new View(context);
lView = inflater.inflate(R.layout.activity_column, null);
// ColImage
ImageView imageView = (ImageView) lView.findViewById(R.id.ColImgPath);
imageView.getLayoutParams().height = 80;
imageView.getLayoutParams().width = 80;
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
imageView.setPadding(5, 5, 5, 5);
int ResID = context.getResources().getIdentifier(MyArrList.get(position).get("ImagePath"), "drawable", context.getPackageName());
imageView.setImageResource(ResID);
// ColImgID
TextView txtPosition = (TextView) lView.findViewById(R.id.ColImgID);
txtPosition.setPadding(10, 0, 0, 0);
txtPosition.setText(MyArrList.get(position).get("ImageID") +".");
// ColPicname
TextView txtPicName = (TextView) lView.findViewById(R.id.ColImgDesc);
txtPicName.setPadding(5, 0, 0, 0);
txtPicName.setText(MyArrList.get(position).get("ImageDesc"));
//imgCmdShared
ImageButton cmdShared = (ImageButton) lView.findViewById(R.id.imgCmdShared);
//cmdShared.setBackgroundColor(Color.TRANSPARENT);
cmdShared.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Toast.makeText(MainActivity.this,"Your Shared (ImageID = " + MyArrList.get(position).get("ImageID") + ")", Toast.LENGTH_LONG).show();
/**
* Command for Shared (Intent to Another Activity)
* Intent newActivity = new Intent(ListDeleteActivity.this,SharedActivity.class);
* newActivity.putExtra("ImgID", MyArrList.get(position).get("ImageID"));
* startActivity(newActivity);
*/
}
});
//imgCmdView
ImageButton cmdView = (ImageButton) lView.findViewById(R.id.imgCmdView);
//cmdView.setBackgroundColor(Color.TRANSPARENT);
cmdView.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Toast.makeText(MainActivity.this,"Your View (ImageID = " + MyArrList.get(position).get("ImageID") + ")", Toast.LENGTH_LONG).show();
/**
* Command for Shared (Intent to Another Activity)
* Intent newActivity = new Intent(ListDeleteActivity.this,ViewActivity.class);
* newActivity.putExtra("ImgID", MyArrList.get(position).get("ImageID"));
* startActivity(newActivity);
*/
}
});
// imgCmdDelete
ImageButton cmdDelete = (ImageButton) lView.findViewById(R.id.imgCmdDelete);
//cmdDelete.setBackgroundColor(Color.TRANSPARENT);
final AlertDialog.Builder adb = new AlertDialog.Builder(MainActivity.this);
cmdDelete.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
adb.setTitle("Delete?");
adb.setMessage("Are you sure delete [" + MyArrList.get(position).get("ImageDesc") +"]");
adb.setNegativeButton("Cancel", null);
adb.setPositiveButton("Ok", new AlertDialog.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"Your Delete (ImageID = " + MyArrList.get(position).get("ImageID") + ")", Toast.LENGTH_LONG).show();
/**
* Command for Delete
* Eg : myDBClass.DeleteData(MyArrList.get(position).get("ImageID"));
*/
}});
adb.show();
}
});
} else {
lView = (View) convertView;
}
return lView;
}
จากตัวอย่างจะเห็นว่าภายใต้ method ของ getView() สามารถทำการ findViewById() ของ Widgets ที่อยู่ในแต่ล่ะ Rows โดยจะทำงานเป็น Loop และเมื่อ findViewById() เจอ Widgets ตัวนั้น ๆ ก็สามารถสร้าง Event ต่าง ๆ ได้โดยไม่ยาก
Code ทั้งหมด
โครงสร้างของไฟล์ประกอบด้วย 3 ไฟล์คือ MainActivity.java, activity_main.xml และ activity_column.xml
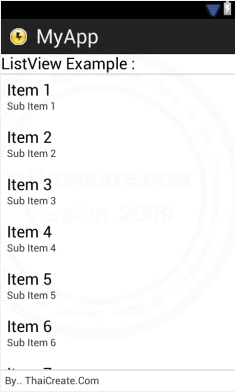
activity_main.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="ListView Example : "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1">
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip" >
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
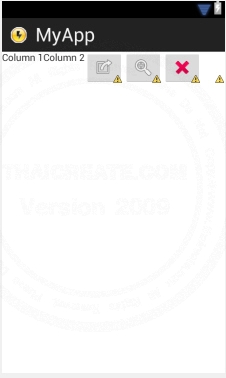
activity_column.xml
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<TableRow
android:id="@+id/tableRow3"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<ImageView
android:id="@+id/ColImgPath"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/ColImgID"
android:text="Column 1" />
<TextView
android:id="@+id/ColImgDesc"
android:text="Column 2" />
<ImageButton
android:id="@+id/imgCmdShared"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@android:drawable/ic_menu_set_as" />
<ImageButton
android:id="@+id/imgCmdView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@android:drawable/ic_menu_zoom" />
<ImageButton
android:id="@+id/imgCmdDelete"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@android:drawable/ic_delete" />
</TableRow>
</TableLayout>
ในไฟล์ของ activity_column.xml จะสมมุตว่ามีปุ่มอยู่ 3 ตัวคือ Shared, View และ Delete ซึ่ง Button เหล่านี้ใช้ Widgets ของ ImageButton
Code ของ Java
MainActivity.java
package com.myapp;
import java.util.ArrayList;
import java.util.HashMap;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageButton;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.Color;
public class MainActivity extends Activity {
ArrayList<HashMap<String, String>> MyArrList;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
MyArrList = new ArrayList<HashMap<String, String>>();
HashMap<String, String> map;
/*** Rows 1 ***/
map = new HashMap<String, String>();
map.put("ImageID", "1");
map.put("ImageDesc", "Sea View1");
map.put("ImagePath", "pic_a");
MyArrList.add(map);
/*** Rows 2 ***/
map = new HashMap<String, String>();
map.put("ImageID", "2");
map.put("ImageDesc", "Sea View2");
map.put("ImagePath", "pic_b");
MyArrList.add(map);
/*** Rows 3 ***/
map = new HashMap<String, String>();
map.put("ImageID", "3");
map.put("ImageDesc", "Sea View 3");
map.put("ImagePath", "pic_c");
MyArrList.add(map);
/*** Rows 4 ***/
map = new HashMap<String, String>();
map.put("ImageID", "4");
map.put("ImageDesc", "Sea View 4");
map.put("ImagePath", "pic_d");
MyArrList.add(map);
// listView1
final ListView lstView1 = (ListView)findViewById(R.id.listView1);
lstView1.setAdapter(new ImageAdapter(this));
}
public class ImageAdapter extends BaseAdapter
{
private Context context;
public ImageAdapter(Context c)
{
// TODO Auto-generated method stub
context = c;
}
public int getCount() {
// TODO Auto-generated method stub
return MyArrList.size();
}
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
public View getView(final int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
if (convertView == null) {
convertView = inflater.inflate(R.layout.activity_column, null);
}
// ColImage
ImageView imageView = (ImageView) convertView.findViewById(R.id.ColImgPath);
imageView.getLayoutParams().height = 80;
imageView.getLayoutParams().width = 80;
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
imageView.setPadding(5, 5, 5, 5);
int ResID = context.getResources().getIdentifier(MyArrList.get(position).get("ImagePath"), "drawable", context.getPackageName());
imageView.setImageResource(ResID);
// ColImgID
TextView txtPosition = (TextView) convertView.findViewById(R.id.ColImgID);
txtPosition.setPadding(10, 0, 0, 0);
txtPosition.setText(MyArrList.get(position).get("ImageID") +".");
// ColPicname
TextView txtPicName = (TextView) convertView.findViewById(R.id.ColImgDesc);
txtPicName.setPadding(5, 0, 0, 0);
txtPicName.setText(MyArrList.get(position).get("ImageDesc"));
//imgCmdShared
ImageButton cmdShared = (ImageButton) convertView.findViewById(R.id.imgCmdShared);
//cmdShared.setBackgroundColor(Color.TRANSPARENT);
cmdShared.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Toast.makeText(MainActivity.this,"Your Shared (ImageID = " + MyArrList.get(position).get("ImageID") + ")",Toast.LENGTH_LONG).show();
/**
* Command for Shared (Intent to Another Activity)
* Intent newActivity = new Intent(ListDeleteActivity.this,SharedActivity.class);
* newActivity.putExtra("ImgID", MyArrList.get(position).get("ImageID"));
* startActivity(newActivity);
*/
}
});
//imgCmdView
ImageButton cmdView = (ImageButton) convertView.findViewById(R.id.imgCmdView);
//cmdView.setBackgroundColor(Color.TRANSPARENT);
cmdView.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Toast.makeText(MainActivity.this,"Your View (ImageID = " + MyArrList.get(position).get("ImageID") + ")",Toast.LENGTH_LONG).show();
/**
* Command for Shared (Intent to Another Activity)
* Intent newActivity = new Intent(ListDeleteActivity.this,ViewActivity.class);
* newActivity.putExtra("ImgID", MyArrList.get(position).get("ImageID"));
* startActivity(newActivity);
*/
}
});
// imgCmdDelete
ImageButton cmdDelete = (ImageButton) convertView.findViewById(R.id.imgCmdDelete);
//cmdDelete.setBackgroundColor(Color.TRANSPARENT);
final AlertDialog.Builder adb = new AlertDialog.Builder(MainActivity.this);
cmdDelete.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
adb.setTitle("Delete?");
adb.setMessage("Are you sure delete [" + MyArrList.get(position).get("ImageDesc") +"]");
adb.setNegativeButton("Cancel", null);
adb.setPositiveButton("Ok", new AlertDialog.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"Your Delete (ImageID = " + MyArrList.get(position).get("ImageID") + ")",Toast.LENGTH_LONG).show();
/**
* Command for Delete
* Eg : myDBClass.DeleteData(MyArrList.get(position).get("ImageID"));
*/
}});
adb.show();
}
});
return convertView;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
คำอธิบาย
ใน Code ของ Java จะมีการสร้างข้อมูลของ ArrayList แบบ Hashmap ที่มีอยู่ 4 รายการดังรูป
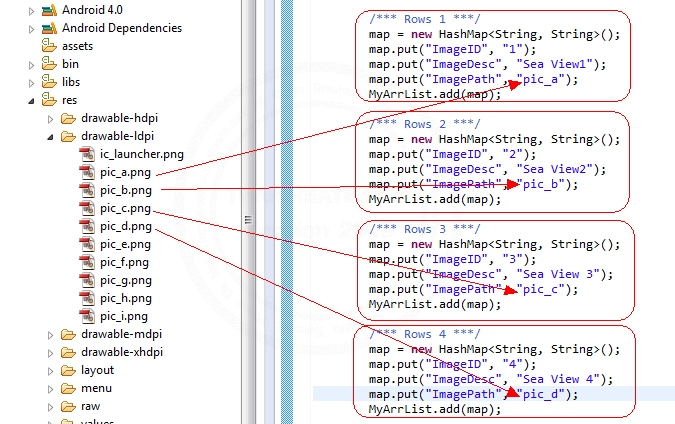
โดยใช้ Widgets ของ ImageView และเรีกยภาพทีอยู่ใน Resource ดังรูป
Screenshot
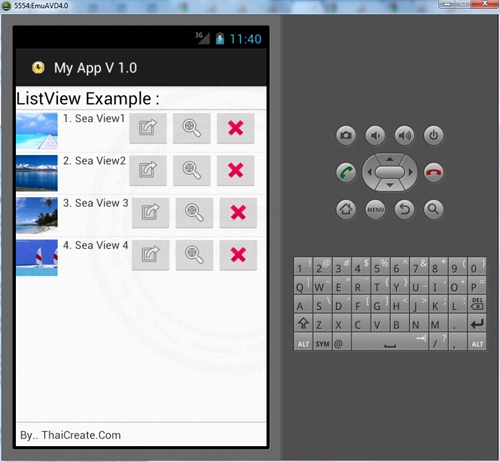
แสดงหน้าแรกซึ่ง ListView จะมีข้อมูลอยู่ 4 รายการ
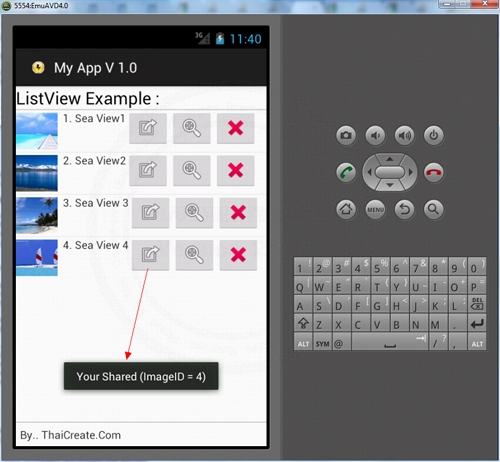
ลองคลิกที่ปุ่ม Shared ก็จะเป็นการเลือกรายการ Shared ข้อมูล
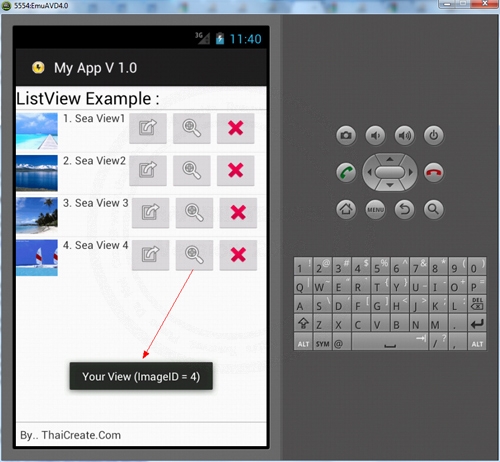
หรือปุ่ม View จะเป็นการ View ดูข้อมูล
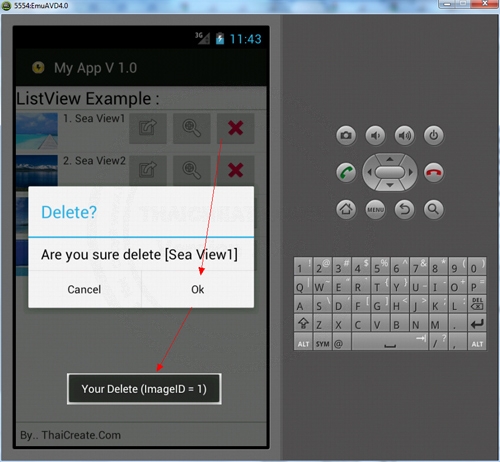
กรณีที่เป็นปุ่มลบ Delete จะใส่ Confirm Button เข้าไปด้วย
เพิ่มเติม
สำหรับปุ่มในแต่ล่ะเหตุการณ์สามารถเชื่อมโยงข้อมูลเหล่านี้ไปยัง Activity อื่น ๆ ด้วยการใช้ Intent Activity หรือการทำเป็น Context Menu เพื่อจะควบคุม Event ต่าง ๆ ว่าจะให้เกิดอะไรขึ้น
|