ตอนที่ 5 : Show Case 1 : Register Form (Android C# (Xamarin) and Mobile Services) |
ตอนที่ 5 : Show Case 1 : Register Form (Android C# (Xamarin) and Mobile Services) บทความนี้จะเป็นการตัวอย่างการทำระบบ Member register รับข้อมูลจากเครื่อง Android ด้วยภาษา C# (Xamarin) เช่น username , password , name , email และ tel โดยจะทำการส่งข้อมูลทั้งหมดเหล่านี้ไปจัดเก็บไว้ที่ตาราง Table ของ Mobile Service ที่อยู่บน Windows Azure ในรูปแบบของการ Register Form หรือ ลงทะเบียนสมาชิก
Android C# (Xamarin) Mobile Services Register Form
บทความนี้ถือเป็นการทบทวนและประยุกต์ใช้จากการศึกษาในหัวข้อที่ผ่าน ๆ มา ซึ่งการทำนั้นก็ไม่ต่างอะไรกับการออกแบบ Form รับข้อมูลบน Android ด้วย C# และ Insert ลงไปใน Table ของ Mobile Services
Syntax การ Register ข้อมูล
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
EditText txtUsername = FindViewById<EditText>(Resource.Id.txtUsername);
EditText txtPassword = FindViewById<EditText>(Resource.Id.txtPassword);
EditText txtName = FindViewById<EditText>(Resource.Id.txtName);
EditText txtEmail = FindViewById<EditText>(Resource.Id.txtEmail);
EditText txtTel = FindViewById<EditText>(Resource.Id.txtTel);
memberTable = client.GetTable<MyMember>();
var item = new MyMember
{
Username = txtUsername.Text,
Password = txtPassword.Text,
Name = txtName.Text,
Email = txtEmail.Text,
Tel = txtTel.Text
};
await memberTable.InsertAsync(item);
Example ตัวอย่างการทำระบบลงทะเบียน Member Register Form ด้วย Android C# (Xamarin)
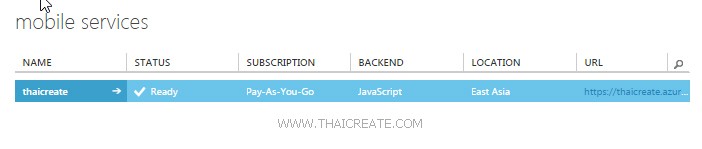
ตอนนี้เรามี Mobile Services อยู่ 1 รายการ
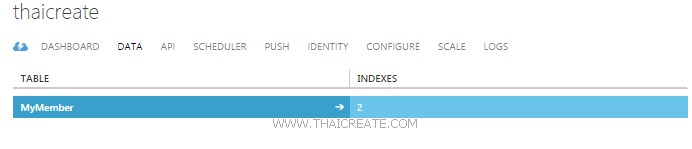
ชื่อตารางว่า MyMember
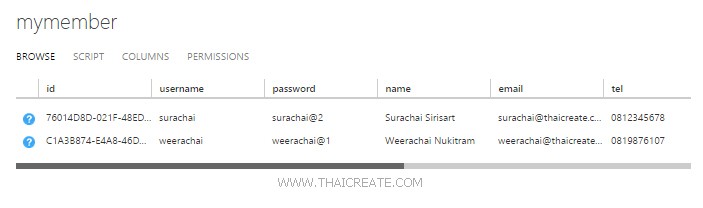
มีข้อมูลอยู่ 2 รายการ

กลับมายัง Android Project บนโปรแกรม Visual Studio
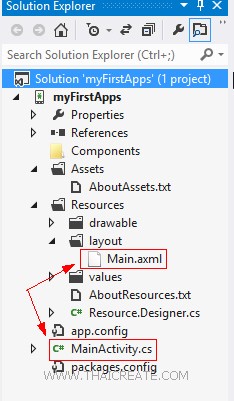
เป็นโครงสร้างของไฟล์ทั้งหมด
Main.axml
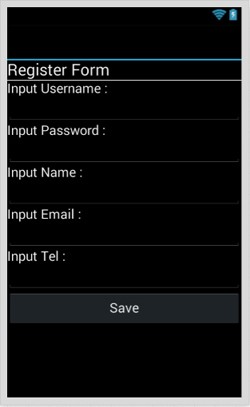
<?xml version="1.0" encoding="utf-8"?>
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Register Form "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Username :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Password :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Name :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Email :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:ems="10" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Tel :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtTel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="phone"
android:ems="10" />
<Button
android:id="@+id/btnSave"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Save" />
</TableLayout>
</TableLayout>
MainActivity.cs
using System;
using Android.App;
using Android.Content;
using Android.Runtime;
using Android.Views;
using Android.Widget;
using Android.OS;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
namespace myFirstApps
{
public class MyMember
{
public int Id { get; set; }
[JsonProperty(PropertyName = "username")]
public string Username { get; set; }
[JsonProperty(PropertyName = "password")]
public string Password { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "email")]
public string Email { get; set; }
[JsonProperty(PropertyName = "tel")]
public string Tel { get; set; }
}
[Activity(Label = "myFirstApps", MainLauncher = true, Icon = "@drawable/icon")]
public class MainActivity : Activity
{
public const string ApplicationURL = @"https://thaicreate.azure-mobile.net/";
public const string ApplicationKey = @"wXYfvYuGLIaDCZdqHjTwDgeDSHZOtL94";
private MobileServiceClient client; // Mobile Service Client references
private IMobileServiceTable<MyMember> memberTable; // Mobile Service Table used to access data
protected override void OnCreate(Bundle bundle)
{
base.OnCreate(bundle);
// Set our view from the "main" layout resource
SetContentView(Resource.Layout.Main);
// Save
Button btnSave = FindViewById<Button>(Resource.Id.btnSave);
btnSave.Click += (object sender, EventArgs e) =>
{
btnSaveDataClick(sender, e);
};
}
private async void btnSaveDataClick(object sender, EventArgs e)
{
try
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
EditText txtUsername = FindViewById<EditText>(Resource.Id.txtUsername);
EditText txtPassword = FindViewById<EditText>(Resource.Id.txtPassword);
EditText txtName = FindViewById<EditText>(Resource.Id.txtName);
EditText txtEmail = FindViewById<EditText>(Resource.Id.txtEmail);
EditText txtTel = FindViewById<EditText>(Resource.Id.txtTel);
memberTable = client.GetTable<MyMember>();
var item = new MyMember
{
Username = txtUsername.Text,
Password = txtPassword.Text,
Name = txtName.Text,
Email = txtEmail.Text,
Tel = txtTel.Text
};
await memberTable.InsertAsync(item);
AlertDialog.Builder builder = new AlertDialog.Builder(this);
AlertDialog alert = builder.Create();
alert.SetTitle("Result");
alert.SetMessage("Register Data Successfully.");
alert.Show();
}
catch (Exception ex)
{
AlertDialog.Builder builder = new AlertDialog.Builder(this);
AlertDialog alert = builder.Create();
alert.SetTitle("Result");
alert.SetMessage("Error : " + ex.Message);
alert.Show();
}
}
}
}

ทดสอบการทำงาน
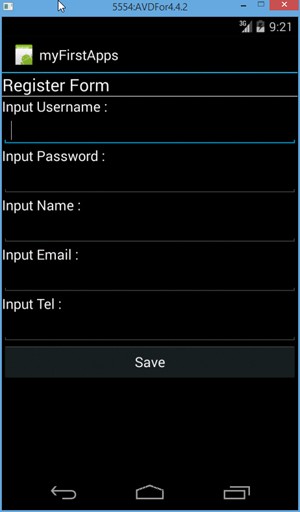
Register Form ทำการ Input ข้อมูล ผ่าน Android Emulator
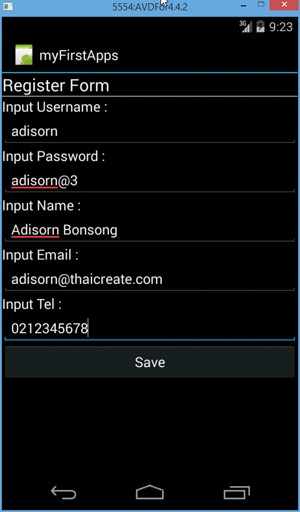
Register Form ทดสอบการ Input ข้อมูล
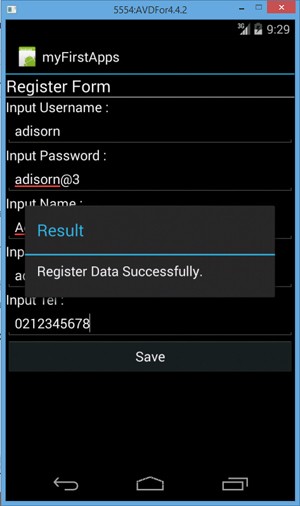
เมื่อ Input ข้อมูลเสร็จสิ้น
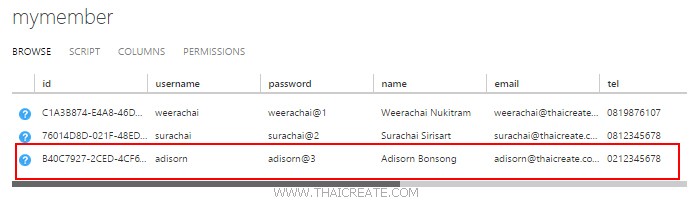
เมื่อกลับไปดูที่ Mobile Services บน Windows Azure ก็จะมีข้อมูลกรากฏขึ้นมา 1 รายการ
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-09-23 13:13:26 /
2017-03-26 21:02:06 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|