ตอนที่ 6 : Show Case 2 : Login User Password (Android C# (Xamarin) and Mobile Services) |
ตอนที่ 6 : Show Case 2 : Login User Password (Android C# (Xamarin) and Mobile Services) หลังจากบทความก่อนหน้านี้เราได้ทำระบบ Register Form บน Android C# (Xamarin) และส่งข้อมูลไปเก็บไว้ที่ Azure Mobile Services ไปเรียบร้อยแล้ว บทความนี้จะเป็นการทำระบบ Login Form ด้วย Username และ Password โดยข้อมูลและ Table ถูกจัดเก็บไว้ที่ Mobile Services บน Windows Azure และหงัจากที่ Login ผ่านแล้ว เราจะดึงข้อมูลของ User ที่ Login มาแสดงในหน้า Info
Android C# (Xamarin) Mobile Services Login Form
รูปแบบการตรวจสอบ Username และ Password
EditText txtUsername = FindViewById<EditText>(Resource.Id.txtUsername);
EditText txtPassword = FindViewById<EditText>(Resource.Id.txtPassword);
memberTable = client.GetTable<MyMember>();
var data = await memberTable.Where(item => item.Username == txtUsername.Text
&& item.Password == txtPassword.Text).ToListAsync();
if (data.Count == 0)
{
AlertDialog.Builder builder = new AlertDialog.Builder(this);
AlertDialog alert = builder.Create();
alert.SetTitle("Result");
alert.SetMessage("Incorrect Username and Password!");
alert.Show();
}
else
{
var detail = new Intent(this, typeof(DetailActivity));
detail.PutExtra("sUsername", txtUsername.Text);
StartActivity(detail);
}
รูปแบบการแสดง Member Info
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
string strUsername = Intent.GetStringExtra("sUsername");
TextView txtUsername = FindViewById<TextView>(Resource.Id.txtUsername);
TextView txtPassword = FindViewById<TextView>(Resource.Id.txtPassword);
TextView txtName = FindViewById<TextView>(Resource.Id.txtName);
TextView txtEmail = FindViewById<TextView>(Resource.Id.txtEmail);
TextView txtTel = FindViewById<TextView>(Resource.Id.txtTel);
memberTable = client.GetTable<MyMember>();
var info = await memberTable.Where(item => item.Username == strUsername).ToCollectionAsync();
if (info.Count > 0)
{
MyMember member = info[0];
txtUsername.Text = member.Username.ToString();
txtPassword.Text = member.Password.ToString();
txtName.Text = member.Name.ToString();
txtEmail.Text = member.Email.ToString();
txtTel.Text = member.Tel.ToString();
}
สำหรับขั้นตอนนั้นก็คือสร้าง Form Activity สำหรับตรวจสอบ Username และ Password และเมื่อ Login ผ่านก็จะทำการ Intent ไปยังอีก Activity เพื่อแสดงข้อมูลของ User นั้น ๆ ที่ได้ทำการ Login เข้ามาในระบบ
Example ตัวอย่างการทำระบบล็อดอิน Member Login และแสดง Member Information
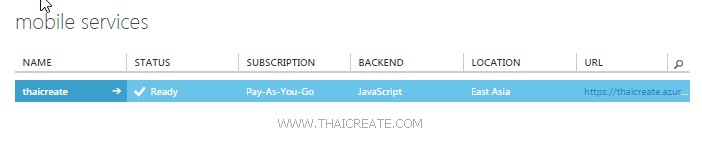
ตอนนี้เรามี Mobile Services อยู่ 1 รายการ
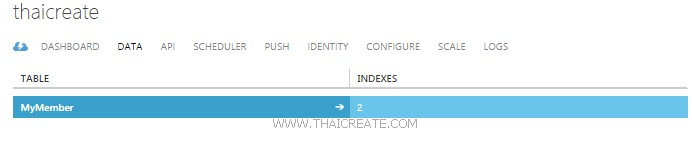
ชื่อตารางว่า MyMember
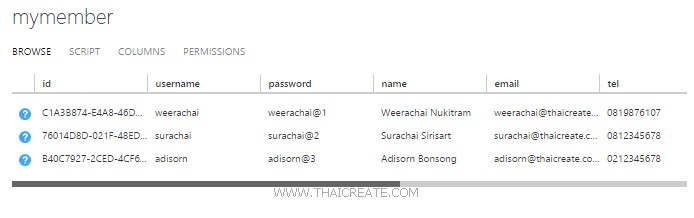
มีข้อมูลอยู่ 3 รายการ
กลับมายัง Android C# Project บนโปรแกรม Visual Studio
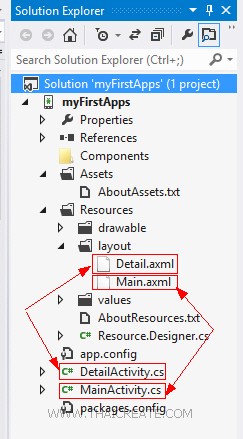
เรามีไฟล์ Activity และ Layout อยู่ 2 ชุดคือ
- MainActivity.cs กับ Main.axml เป็นไฟล์ที่ทำหน้าที่แสดงหน้า Login และตรวจสอบ Username / Password
- DetailActivity.cs และ Detail.axml แสดง Member Info และรายละเอียดของ Member ที่ Login เข้ามาในระบบ
Main.axml
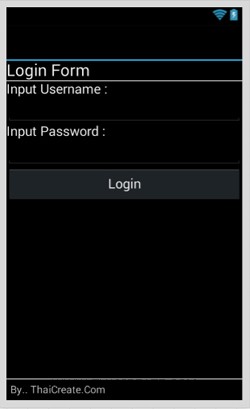
<?xml version="1.0" encoding="utf-8"?>
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="Login Form "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Username :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10" />
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Input Password :"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPassword" />
<Button
android:id="@+id/btnLogin"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Login" />
</TableLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip">
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
MainActivity.cs
using System;
using Android.App;
using Android.Content;
using Android.Runtime;
using Android.Views;
using Android.Widget;
using Android.OS;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
namespace myFirstApps
{
public class MyMember
{
public int Id { get; set; }
[JsonProperty(PropertyName = "username")]
public string Username { get; set; }
[JsonProperty(PropertyName = "password")]
public string Password { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "email")]
public string Email { get; set; }
[JsonProperty(PropertyName = "tel")]
public string Tel { get; set; }
}
[Activity(Label = "myFirstApps", MainLauncher = true, Icon = "@drawable/icon")]
public class MainActivity : Activity
{
public const string ApplicationURL = @"https://thaicreate.azure-mobile.net/";
public const string ApplicationKey = @"wXYfvYuGLIaDCZdqHjTwDgeDSHZOtL94";
private MobileServiceClient client; // Mobile Service Client references
private IMobileServiceTable<MyMember> memberTable; // Mobile Service Table used to access data
protected override void OnCreate(Bundle bundle)
{
base.OnCreate(bundle);
// Set our view from the "main" layout resource
SetContentView(Resource.Layout.Main);
// Login
Button btnLogin = FindViewById<Button>(Resource.Id.btnLogin);
btnLogin.Click += (object sender, EventArgs e) =>
{
btnLoginClick(sender, e);
};
}
private async void btnLoginClick(object sender, EventArgs e)
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
EditText txtUsername = FindViewById<EditText>(Resource.Id.txtUsername);
EditText txtPassword = FindViewById<EditText>(Resource.Id.txtPassword);
memberTable = client.GetTable<MyMember>();
var data = await memberTable.Where(item => item.Username == txtUsername.Text
&& item.Password == txtPassword.Text).ToListAsync();
if (data.Count == 0)
{
AlertDialog.Builder builder = new AlertDialog.Builder(this);
AlertDialog alert = builder.Create();
alert.SetTitle("Result");
alert.SetMessage("Incorrect Username and Password!");
alert.Show();
}
else
{
var detail = new Intent(this, typeof(DetailActivity));
detail.PutExtra("sUsername", txtUsername.Text);
StartActivity(detail);
}
}
}
}
Detail.axml
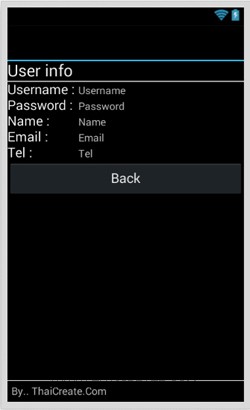
<?xml version="1.0" encoding="utf-8"?>
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/tableLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TableRow
android:id="@+id/tableRow1"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center"
android:text="User info "
android:layout_span="1"
android:textAppearance="?android:attr/textAppearanceLarge" />
</TableRow>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<TableLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="0.1"
android:orientation="horizontal">
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtUsername"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtPassword"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Password" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Email" />
</TableRow>
<TableRow
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tel : "
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/txtTel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tel" />
</TableRow>
<Button
android:id="@+id/btnBack"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Back" />
</TableLayout>
<View
android:layout_height="1dip"
android:background="#CCCCCC" />
<LinearLayout
android:id="@+id/LinearLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="5dip">
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="By.. ThaiCreate.Com" />
</LinearLayout>
</TableLayout>
DetailActivity.cs
using System;
using Android.App;
using Android.Content;
using Android.Runtime;
using Android.Views;
using Android.Widget;
using Android.OS;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
namespace myFirstApps
{
[Activity(Label = "Detail")]
public class DetailActivity : Activity
{
public const string ApplicationURL = @"https://thaicreate.azure-mobile.net/";
public const string ApplicationKey = @"wXYfvYuGLIaDCZdqHjTwDgeDSHZOtL94";
private MobileServiceClient client; // Mobile Service Client references
private IMobileServiceTable<MyMember> memberTable; // Mobile Service Table used to access data
protected override void OnCreate(Bundle bundle)
{
base.OnCreate(bundle);
// Set our view from the "detail" layout resource
SetContentView(Resource.Layout.Detail);
LoadInfo();
}
private async void LoadInfo()
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
string strUsername = Intent.GetStringExtra("sUsername");
TextView txtUsername = FindViewById<TextView>(Resource.Id.txtUsername);
TextView txtPassword = FindViewById<TextView>(Resource.Id.txtPassword);
TextView txtName = FindViewById<TextView>(Resource.Id.txtName);
TextView txtEmail = FindViewById<TextView>(Resource.Id.txtEmail);
TextView txtTel = FindViewById<TextView>(Resource.Id.txtTel);
memberTable = client.GetTable<MyMember>();
var info = await memberTable.Where(item => item.Username == strUsername).ToCollectionAsync();
if (info.Count > 0)
{
MyMember member = info[0];
txtUsername.Text = member.Username.ToString();
txtPassword.Text = member.Password.ToString();
txtName.Text = member.Name.ToString();
txtEmail.Text = member.Email.ToString();
txtTel.Text = member.Tel.ToString();
}
}
}
}
ทดสอบการทำงาน
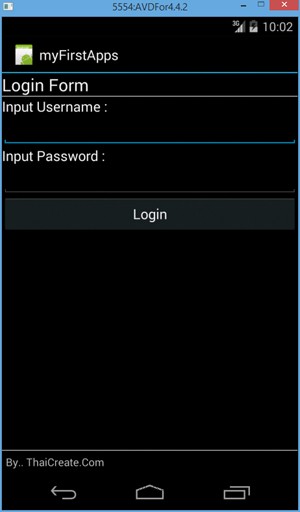
Login Form บน Android Emulator
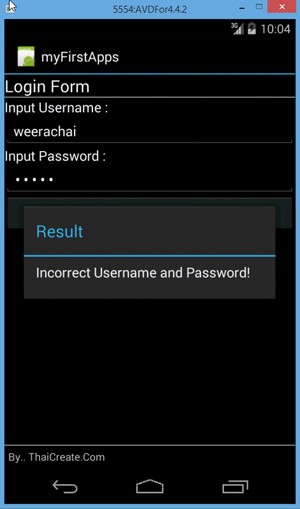
กรณีที่ Login ข้อมูล Username และ Password ผิดพลาด
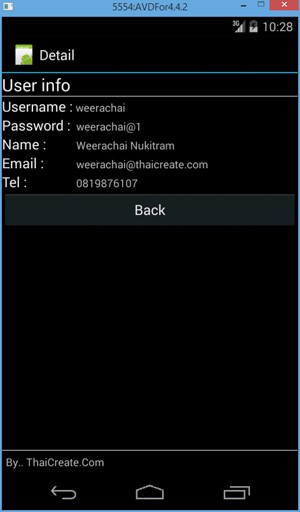
กรณีที่ Login ถูกต้อง จะแสดง Information ของสมาชิกที่ Login เข้ามาในระบบ
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-09-23 13:14:31 /
2017-03-26 21:02:38 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|