ตอนที่ 4 : Android C# (Xamarin) อ่าน Data จาก Table ของ Mobile Services และแสดงผลบน App |
ตอนที่ 4 : Android C# (Xamarin) อ่าน Data จาก Table ของ Mobile Services และแสดงผลบน App บทความนี้จะเป็นการใช้ Android C# (Xamarin) อ่านข้อมูลจาก Table ของ Azure Mobile Services ที่อยู่บน Windows Azure โดยข้อมูลของ Table ได้ถูกจัดเก็บแบบ Column และ Rows คล้าย ๆ กับ Table ของ SQL Database ทั่ว ๆ ไป และใน Android จะมีการใช้ ListView ซึ่งจะแสดงข้อมูลที่อยู่ในรูปแบบของ ArrayAdapter ด้วยการ Loop ข้อมูลและนำข้อมูลที่ได้แสดงผลรายการใน ListView
รูปแบบการอ่านข้อมูลจาก Table ของ Mobile Services ด้วย Android C#
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
memberTable = client.GetTable<MyMember>();
var items = await memberTable.ToListAsync();
Mapping ข้อมูลที่ได้กับ ListView เพื่อแสดงข้อมูลบน Column ผ่าน Adapter
ListView lstView1 = (ListView)FindViewById(Resource.Id.listView1);
mAdapter = new MyMemberAdapter(this, Resource.Layout.Column, items);
lstView1.Adapter = mAdapter;
การแสดงผลต่าง ๆ สามารถเขียนคำสั่งต่าง ๆ ได้ใน BaseAdapter
public class MyMemberAdapter : BaseAdapter<MyMember>
{
public override View GetView(int position, View convertView, ViewGroup parent)
{
}
}
รูปแบบการใช้ Android อ่านข้อมูลจาก Mobile Services บน Windows Azure
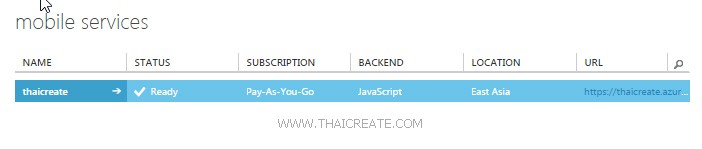
ตอนนี้เรามี Mobile Services อยู่ 1 รายการ
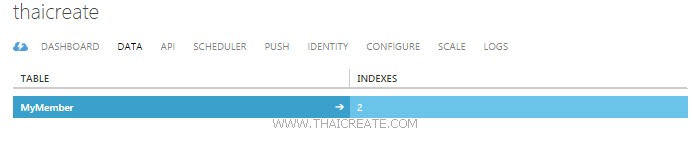
ชื่อตารางว่า MyMember
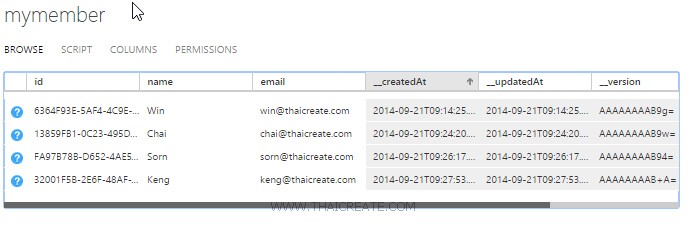
ข้อมูลในตาราง MyMember ประกอบด้วย Column ชื่อว่า id,name,email
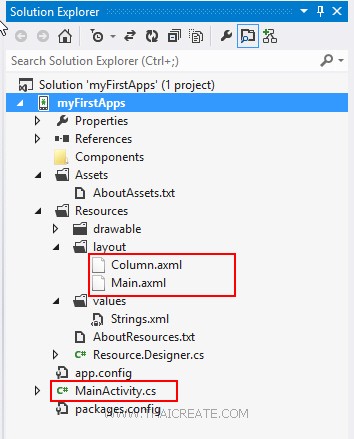
โครงสร้างไฟล์ทั้งหมด
Column.axml
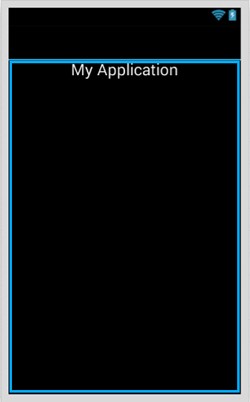
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/linearLayout1"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:id="@+id/col_name"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="name" />
<TextView
android:id="@+id/col_email"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="2"
android:text="email" />
</LinearLayout>
Main.axml
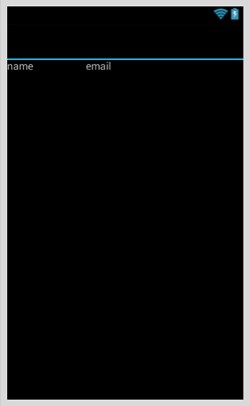
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:minWidth="25px"
android:minHeight="25px">
<TextView
android:text="My Application"
android:textAppearance="?android:attr/textAppearanceLarge"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/textView1"
android:gravity="center" />
<ListView
android:id="@+id/listView1"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
MainActivity.cs
using System;
using Android.App;
using Android.Content;
using Android.Runtime;
using Android.Views;
using Android.Widget;
using Android.OS;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
using System.Collections.Generic;
namespace myFirstApps
{
public class MyMember
{
public int Id { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "email")]
public string Email { get; set; }
}
[Activity(Label = "myFirstApps", MainLauncher = true, Icon = "@drawable/icon")]
public class MainActivity : Activity
{
public const string ApplicationURL = @"https://thaicreate.azure-mobile.net/";
public const string ApplicationKey = @"wXYfvYuGLIaDCZdqHjTwDgeDSHZOtL94";
private MobileServiceClient client; // Mobile Service Client references
private IMobileServiceTable<MyMember> memberTable; // Mobile Service Table used to access data
private MyMemberAdapter mAdapter;
protected override void OnCreate(Bundle bundle)
{
base.OnCreate(bundle);
// Set our view from the "main" layout resource
SetContentView(Resource.Layout.Main);
this.ListData();
}
public async void ListData()
{
client = new MobileServiceClient(ApplicationURL, ApplicationKey);
memberTable = client.GetTable<MyMember>();
var items = await memberTable.ToListAsync();
ListView lstView1 = (ListView)FindViewById(Resource.Id.listView1);
mAdapter = new MyMemberAdapter(this, Resource.Layout.Column, items);
lstView1.Adapter = mAdapter;
}
public class MyMemberAdapter : BaseAdapter<MyMember>
{
int layoutResourceId;
Activity activity;
List<MyMember> items;
public MyMemberAdapter(Activity activity, int layoutResourceId, List<MyMember> items)
: base()
{
this.activity = activity;
this.layoutResourceId = layoutResourceId;
this.items = items;
}
public override long GetItemId(int position)
{
return position;
}
public override int Count
{
get { return items.Count; }
}
public override View GetView(int position, View convertView, ViewGroup parent)
{
View view = convertView; // re-use an existing view, if one is available
if (view == null) // otherwise create a new one
view = activity.LayoutInflater.Inflate(Resource.Layout.Column, null);
var currentItem = items[position];
// name
view.FindViewById<TextView>(Resource.Id.col_name).Text = currentItem.Name;
// email
view.FindViewById<TextView>(Resource.Id.col_email).Text = currentItem.Email;
return view;
}
public override MyMember this[int position]
{
get
{
return items[position];
}
}
}
}
}

ทดสอบการทำงาน
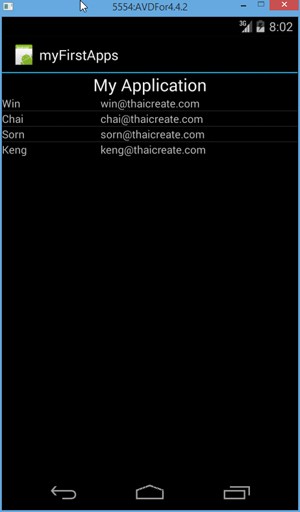
แสดงข้อมูลจาก Table ของ Mobile Services บน Android App
บทความที่เกี่ยวข้อง
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-09-23 13:13:11 /
2017-03-26 21:01:30 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|